How to Write Efficient Data-Driven Tests in Java
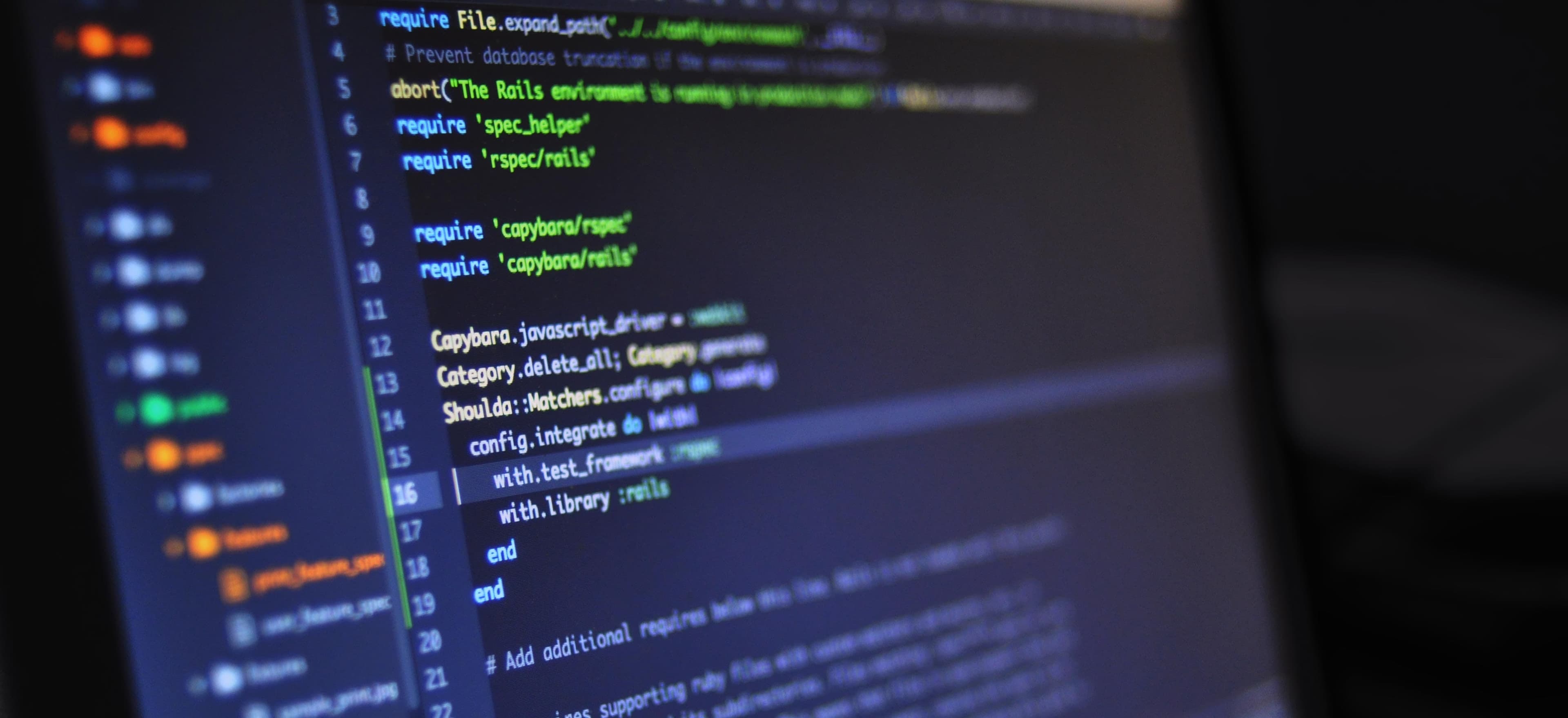
- Published on
How to Write Efficient Data-Driven Tests in Java
When it comes to testing in Java, data-driven tests play a crucial role in ensuring comprehensive test coverage. By incorporating multiple sets of data into a single test, data-driven testing allows you to efficiently validate the behavior of your code across various input scenarios. In this article, we will explore the best practices for writing efficient data-driven tests in Java, using popular frameworks such as JUnit and TestNG.
Understanding Data-Driven Testing
Data-driven testing involves running the same test logic with different input data sets. Instead of writing separate test methods for each set of input, data-driven tests enable you to reuse the test logic and focus on the variations in the input data. This approach not only reduces code duplication but also makes it easier to maintain and extend the test suite as the application evolves.
Setting Up Your Project
Before diving into writing data-driven tests, you need to set up a Java project with the necessary dependencies. For this example, we will use Maven to manage our project and dependencies. If you don't have Maven installed, you can follow the instructions on the official Maven website.
In your pom.xml
file, add the following dependencies for JUnit and TestNG:
<dependencies>
<!-- JUnit 5 Dependency -->
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>5.7.0</version>
<scope>test</scope>
</dependency>
<!-- TestNG Dependency -->
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>7.4.0</version>
<scope>test</scope>
</dependency>
</dependencies>
After adding the dependencies, execute mvn clean install
to ensure that the dependencies are downloaded and added to your project.
Writing Data-Driven Tests with JUnit
Using Parameterized Tests
JUnit 5 provides built-in support for data-driven testing through parameterized tests. You can use the @ParameterizedTest
and @CsvSource
annotations to define parameterized tests with CSV input.
Here's an example of a parameterized test that validates different combinations of input and expected output:
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.CsvSource;
import static org.junit.jupiter.api.Assertions.assertEquals;
class CalculatorTest {
@ParameterizedTest
@CsvSource({"1, 2, 3", "5, 5, 10", "10, 0, 10"})
void add(int a, int b, int expectedResult) {
Calculator calculator = new Calculator();
assertEquals(expectedResult, calculator.add(a, b));
}
}
In this example, the add
method of the Calculator
class is tested with different sets of input (a, b) and their corresponding expected results. The @CsvSource
annotation allows you to specify the input data in a comma-separated format within the test method signature.
Using ValueSource and EnumSource
In addition to @CsvSource
, JUnit 5 supports @ValueSource
and @EnumSource
for parameterized tests. These annotations allow you to provide individual or enumerated values as input to your test methods.
Here's an example using @ValueSource
to test different string lengths:
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.ValueSource;
import static org.junit.jupiter.api.Assertions.assertTrue;
class StringUtilsTest {
@ParameterizedTest
@ValueSource(strings = {"hello", "world", "java"})
void isStringValid(String input) {
assertTrue(input.length() > 0);
}
}
In this example, the isStringValid
method tests whether each input string has a length greater than 0.
Writing Data-Driven Tests with TestNG
Using DataProvider
TestNG, another popular testing framework in the Java ecosystem, offers data-driven testing capabilities through the use of @DataProvider
. With @DataProvider
, you can define a method that supplies data to your test methods.
Here's an example of a data-driven test using TestNG's @DataProvider
:
import org.testng.annotations.DataProvider;
import org.testng.annotations.Test;
import static org.testng.Assert.assertEquals;
public class ArrayUtilsTest {
@DataProvider(name = "arrayData")
public Object[][] arrayData() {
return new Object[][] {
{ new int[]{1, 2, 3}, 6 },
{ new int[]{5, 5, 5}, 15 },
{ new int[]{10, 0, 10}, 20 }
};
}
@Test(dataProvider = "arrayData")
void sumArray(int[] array, int expectedSum) {
ArrayUtils arrayUtils = new ArrayUtils();
assertEquals(expectedSum, arrayUtils.sum(array));
}
}
In this example, the sumArray
method of the ArrayUtils
class is tested with different integer arrays and their expected sums. The @DataProvider
annotation specifies a method that supplies the input data for the test method.
Best Practices for Data-Driven Testing
Keep Data and Logic Separate
When writing data-driven tests, it's essential to keep the test data separate from the test logic. By doing so, you can easily modify or add new test data without changing the test implementation.
Use Meaningful Data
Ensure that the input data in your data-driven tests covers meaningful scenarios and edge cases. This helps in uncovering potential issues and corner cases within your code.
Handle Data-Driven Test Failures Gracefully
When a data-driven test fails, it's crucial to provide clear and specific information about which set of data caused the failure. This aids in debugging and identifying the root cause of the failure.
Leverage External Data Sources
Consider using external data sources, such as CSV files, databases, or JSON files, to drive your data-driven tests. This approach facilitates scalability and reduces the maintenance overhead of managing test data within the test code.
Parameterize Your Tests
Parameterizing tests allows you to run the same test logic with different combinations of input data. This promotes reusability and helps in identifying how the code behaves across various input scenarios.
The Bottom Line
Data-driven testing is a powerful technique for enhancing the effectiveness and efficiency of your test suite. By leveraging frameworks like JUnit and TestNG, you can easily write and maintain data-driven tests that cover a wide range of input scenarios. Keep in mind the best practices outlined in this article to ensure that your data-driven tests are well-structured, meaningful, and resilient to changes in your application.
In conclusion, mastering data-driven testing in Java can significantly contribute to the overall quality and reliability of your software applications.
Start implementing data-driven tests in your Java projects today to experience the benefits firsthand!