Handling Asynchronous Requests in Spring MVC 3
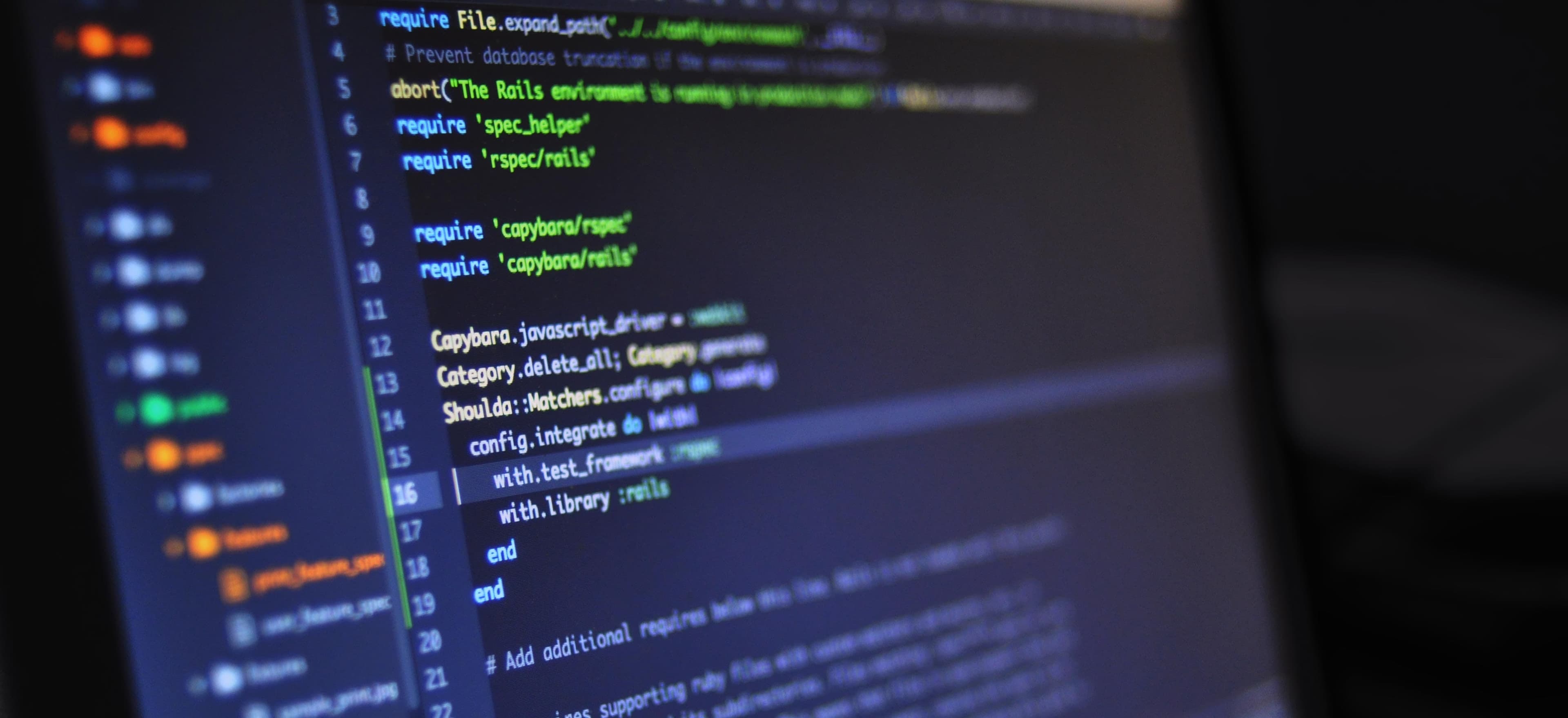
- Published on
Handling Asynchronous Requests in Spring MVC 3
In modern web application development, asynchronous processing has become an essential practice to improve user experience and resource utilization. In a traditional synchronous approach, the server processes one request at a time, which can lead to performance bottlenecks, especially in high-traffic situations. Asynchronous processing allows the server to handle multiple requests concurrently, thus improving responsiveness and scalability.
In this article, we will explore how to handle asynchronous requests in a Spring MVC 3 application, leveraging its built-in support for asynchronous processing.
Prerequisites
Before diving into the implementation, it's assumed that you have a basic understanding of the Spring Framework and its MVC module. If you're new to Spring MVC, I recommend going through the official documentation and tutorials to familiarize yourself with the fundamental concepts.
Setting Up the Project
To get started, let's create a new Spring MVC 3 project or use an existing one for implementing asynchronous request handling. Make sure you have the appropriate dependencies added to your project's build file (e.g., Maven pom.xml
or Gradle build.gradle
) for Spring MVC.
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>3.0.7.RELEASE</version>
</dependency>
Defining a Controller for Asynchronous Processing
In Spring MVC, you can handle asynchronous requests by defining a controller method that returns a Callable
or DeferredResult
as the response. Let's create a simple controller with an asynchronous request mapping to demonstrate this approach.
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.context.request.async.DeferredResult;
@RestController
public class AsyncController {
@RequestMapping("/asyncTask")
public DeferredResult<String> performAsyncTask() {
DeferredResult<String> deferredResult = new DeferredResult<>();
// Simulate a time-consuming task
new Thread(() -> {
// Perform asynchronous processing here
String result = "Async task completed";
deferredResult.setResult(result);
}).start();
return deferredResult;
}
}
In the above example, we've defined a controller AsyncController
with a method performAsyncTask()
mapped to the URL /asyncTask
. The method returns a DeferredResult<String>
to handle the asynchronous processing. Inside the method, we simulate a time-consuming task using a separate thread, and once the task is completed, we set the result on the DeferredResult
.
Why Use DeferredResult?
You might wonder why we use DeferredResult
instead of a standard Callable
. The main advantage of DeferredResult
is that it provides more flexibility in handling asynchronous processing, such as setting a timeout for the result or handling the result in a separate thread pool.
Configuring Asynchronous Support
To enable asynchronous request processing in Spring MVC 3, you need to configure the DispatcherServlet
with the task:annotation-driven
element in the Spring configuration file (e.g., dispatcher-servlet.xml
).
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:task="http://www.springframework.org/schema/task"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
http://www.springframework.org/schema/task
http://www.springframework.org/schema/task/spring-task-3.0.xsd">
<task:annotation-driven />
</beans>
The task:annotation-driven
element enables support for processing annotated methods with asynchronous execution semantics.
Handling Asynchronous Results
Once the asynchronous task is initiated, the client needs a way to receive the result when it's available. This can be done using JavaScript on the client side, making periodic Ajax requests to check for the completion of the task. Alternatively, you can utilize web sockets for real-time updates.
Closing the Chapter
In this article, we've delved into the world of asynchronous request handling in Spring MVC 3, exploring the use of DeferredResult
to facilitate non-blocking, asynchronous processing. By embracing asynchronous processing, you can significantly enhance the responsiveness and scalability of your web application, providing a seamless user experience even under heavy loads.
By implementing asynchronous request handling, developers can effectively mitigate potential performance bottlenecks and cater to the evolving demands of modern web applications. It's a powerful technique that every Spring MVC developer should have in their arsenal.