Rethinking Exception Handling: Beyond Try-Catch
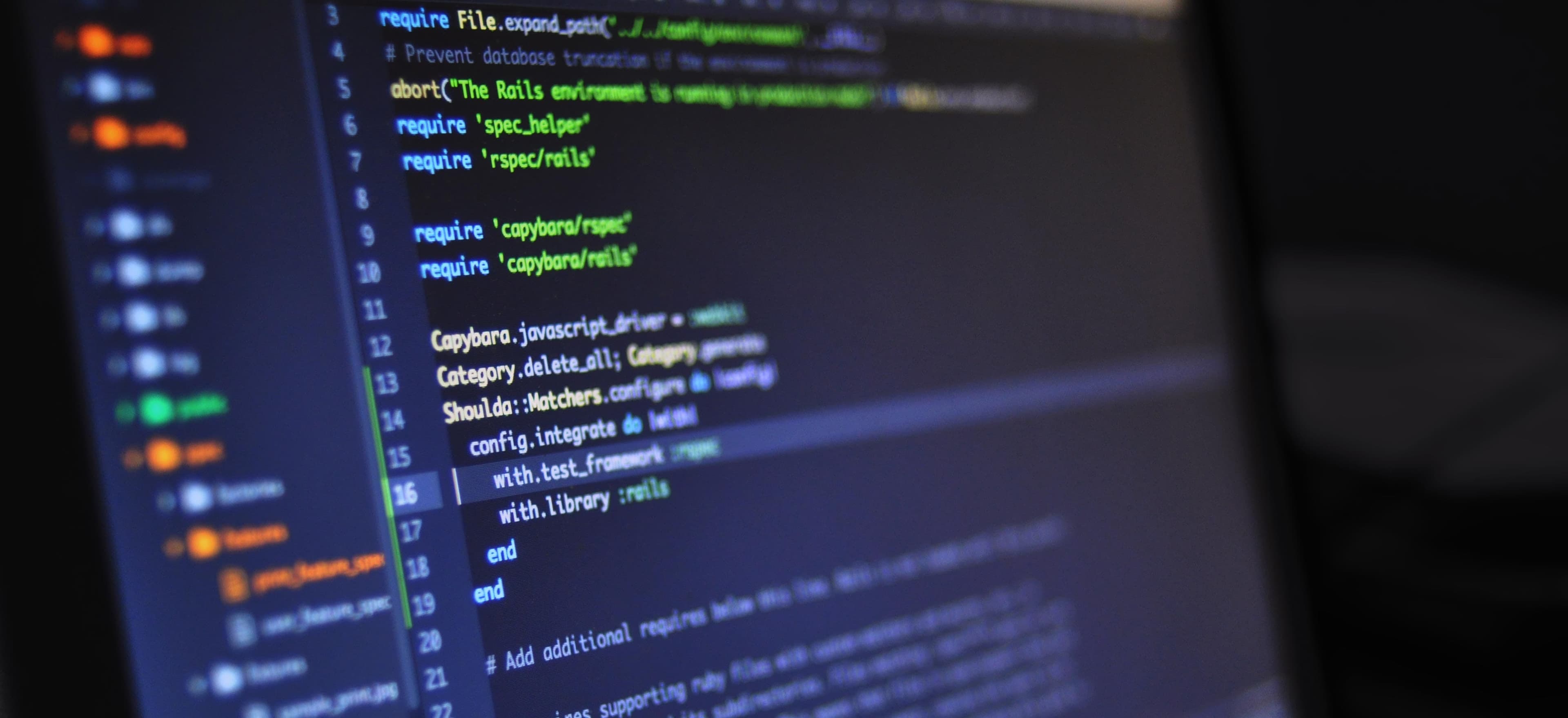
- Published on
Rethinking Exception Handling: Beyond Try-Catch
Exception handling is a critical aspect of Java programming, allowing developers to gracefully manage unexpected events and errors. While the traditional try-catch
block is widely used for handling exceptions, there are alternative approaches that offer more flexibility and control. In this article, we'll explore advanced exception handling techniques in Java, going beyond the conventional try-catch
paradigm.
The Limitations of Try-Catch
The try-catch
block is the cornerstone of Java's exception handling mechanism. It enables developers to encapsulate code that may throw exceptions and provides a structured way to handle those exceptions. However, try-catch
has its limitations:
- Overbearing Syntax: For every block of code that might throw an exception, a corresponding
try-catch
block is required, leading to verbose and cluttered code. - Limited Granularity: Exceptions are caught at the granularity of
try
blocks, potentially making it difficult to distinguish between different types of exceptions within the same block. - Nested Complexity: When working with nested
try-catch
blocks, the code can quickly become convoluted and hard to maintain.
To address these limitations, Java offers more sophisticated constructs for exception handling.
Utilizing Try-With-Resources
Introduced in Java 7, the try-with-resources
statement simplifies resource management by automatically closing resources at the end of the block. It is especially useful when working with I/O operations, such as file handling or database connections.
try (BufferedReader br = new BufferedReader(new FileReader("file.txt"))) {
System.out.println(br.readLine());
} catch (IOException e) {
// Handle the exception
}
In the above example, the BufferedReader
is automatically closed at the end of the try
block, mitigating the need for explicit resource cleanup code.
Custom Exception Handling
Java allows developers to define custom exception classes to encapsulate specific error scenarios within their applications. This provides a more structured and meaningful way to handle exceptional conditions, making the code more readable and maintainable.
class CustomException extends Exception {
public CustomException(String message) {
super(message);
}
}
public class CustomExceptionExample {
public void performOperation() throws CustomException {
// Business logic that may throw CustomException
if (/* error condition */) {
throw new CustomException("Custom error message");
}
}
public static void main(String[] args) {
try {
// Call performOperation method
} catch (CustomException e) {
// Handle CustomException
}
}
}
By creating custom exception classes, developers can convey the nature of the error more clearly and handle specific exceptions with precision.
Exception Chaining
In complex systems, it's common for exceptions to be caused by other exceptions. Java provides the means to capture this relationship through exception chaining, allowing developers to maintain a comprehensive trail of the exceptions that led to the current error.
try {
// Code that may throw an exception
} catch (IOException e) {
throw new CustomException("Failed to perform operation", e);
}
In the above example, the CustomException
is constructed with the original IOException
as its cause, enabling the propagation of the original exception context.
Leveraging Optional for Error Handling
Java 8 introduced the Optional
class, offering a more functional approach to handling potential absence of a value. While commonly used for dealing with null values, Optional
can also be leveraged for error handling scenarios.
Optional<String> result = someOperationThatMayReturnNull();
if (result.isPresent()) {
// Process the result
} else {
// Handle the absence of result
}
By embracing Optional
, developers can express error scenarios in a more expressive manner, promoting a clearer understanding of the code's intent.
Dealing with Unchecked Exceptions
Unchecked exceptions, such as RuntimeException
, do not need to be declared in a method's throws clause, making them convenient for handling unexpected errors. However, they can still be caught and processed using advanced techniques.
try {
// Code that may throw an unchecked exception
} catch (ArithmeticException | NullPointerException e) {
// Handle specific unchecked exceptions
}
By employing multi-catch clauses, developers can efficiently manage different types of unchecked exceptions within the same block, improving code conciseness and maintainability.
Lessons Learned
While the try-catch
block remains fundamental to Java's exception handling, advanced techniques such as try-with-resources
, custom exceptions, exception chaining, Optional
usage, and handling unchecked exceptions provide more sophisticated and expressive means for managing exceptional conditions in Java applications. By leveraging these techniques, developers can enhance code readability, maintainability, and error resilience.
In conclusion, rethinking exception handling in Java involves exploring and embracing the diverse and powerful tools available within the language, enabling developers to elevate their error management strategies to new heights.
By transcending the boundaries of traditional exception handling, Java developers can construct robust, resilient, and expressive code that adeptly navigates the complexities of error scenarios, thus contributing to the overall quality and reliability of their applications.
For more in-depth coverage of advanced Java techniques, consider exploring the official Java Tutorials provided by Oracle.
Incorporating advanced exception handling techniques into your Java arsenal can elevate your coding prowess and pave the way for more robust and maintainable applications. Embrace the power of advanced exception handling in Java and unlock the potential for creating exceptional software.