Maximizing Query Performance with Field Projections
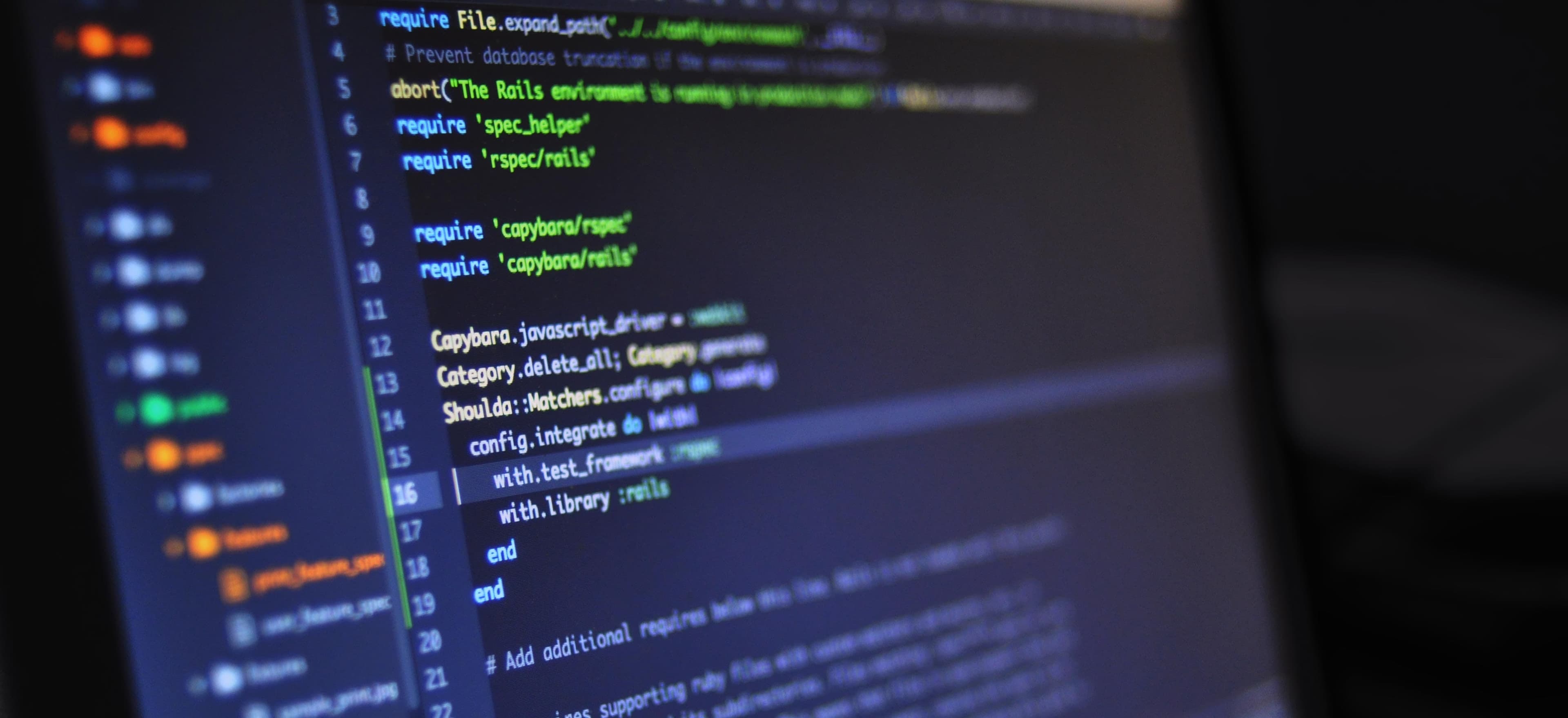
- Published on
Maximizing Query Performance with Field Projections
When working with large datasets in Java applications, optimizing query performance is crucial for delivering a responsive user experience. One powerful technique for improving performance is the use of field projections in database queries. In this article, we will explore what field projections are, how they can enhance query performance, and how to implement them in Java using popular frameworks such as Hibernate.
Understanding Field Projections
Field projections involve specifying which fields to retrieve from the database when executing a query, rather than fetching the entire record. By limiting the data returned to only the necessary fields, field projections can significantly reduce the amount of data transported between the database and the application, leading to faster query execution and improved overall performance.
The Benefits of Field Projections
Reduced Data Transfer
When a query retrieves only the required fields from the database, it reduces the amount of data transferred over the network. This can be particularly beneficial when dealing with large datasets, as it minimizes network latency and resource consumption.
Improved Memory Utilization
Fetching a smaller set of fields means that less memory is required to hold the query result. This can be especially advantageous in memory-constrained environments or when processing a high volume of concurrent queries.
Potential Index Utilization
Field projections can enable the database to optimize query execution by leveraging appropriate indexes more effectively. By limiting the fields in a query, the database may be able to utilize covering indexes, which store all the queried fields, resulting in faster lookups and reduced disk I/O.
Implementing Field Projections in Java
Now, let's take a look at how we can implement field projections in Java using Hibernate, a popular object-relational mapping (ORM) framework.
Using Hibernate's Criteria API
CriteriaBuilder cb = entityManager.getCriteriaBuilder();
CriteriaQuery<User> query = cb.createQuery(User.class);
Root<User> root = query.from(User.class);
// Specify the fields to be returned
query.select(cb.construct(User.class, root.get("id"), root.get("name")));
List<User> users = entityManager.createQuery(query).getResultList();
In this example, we use Hibernate's Criteria API to construct a query that selects only the "id" and "name" fields from the "User" entity. By specifying the fields to be returned, we optimize the query to fetch only the necessary data from the database.
Leveraging JPQL (Java Persistence Query Language)
TypedQuery<Object[]> query = entityManager.createQuery("SELECT u.id, u.name FROM User u", Object[].class);
List<Object[]> results = query.getResultList();
for(Object[] result : results) {
Long id = (Long) result[0];
String name = (String) result[1];
// Process the retrieved fields
}
In this JPQL example, we directly specify the fields to retrieve from the "User" entity, resulting in a query that only returns the "id" and "name" fields. By leveraging JPQL, we can achieve efficient field projections in our Java application.
Best Practices for Field Projections
Analyze Query Patterns
Before applying field projections, analyze the query patterns in your application to identify which fields are frequently accessed. By focusing on commonly used fields, you can maximize the impact of field projections on query performance.
Consider Read-Heavy Operations
Field projections are especially beneficial for read-heavy operations where data retrieval is a primary concern. By optimizing the fields returned in such scenarios, you can achieve substantial performance improvements.
Use Profiling and Benchmarking
Utilize profiling and benchmarking tools to measure the impact of field projections on query performance. This empirical approach can help validate the effectiveness of your field projection optimizations and guide further refinement.
Wrapping Up
Field projections offer a potent method for enhancing query performance in Java applications, particularly when dealing with large datasets and read-heavy operations. By selectively retrieving only the necessary fields from the database, developers can reduce data transfer, improve memory utilization, and enable potential index optimization, ultimately leading to significant performance gains. When applied judiciously and in alignment with the specific query patterns of an application, field projections can be a valuable tool for maximizing the efficiency of database queries.
Incorporating field projections as part of a comprehensive performance optimization strategy can yield tangible benefits, making it a technique well worth mastering for Java developers working with database-driven applications.
By integrating these best practices and examples into your Java development workflows, you can enrich your skillset and elevate the performance of your database queries. Embrace the power of field projections to unlock enhanced query performance and deliver robust, efficient Java applications to your users.
Remember that adopting efficient querying practices, such as field projections, not only fosters better user experiences but also demonstrates your commitment to delivering high-performance, well-architected solutions.
For further reading on Java query optimization and Hibernate best practices, consider exploring Hibernate Best Practices and Optimizing Database Access with Hibernate.
Level up your query optimization skills and elevate the performance of your Java applications with field projections!