Managing Transient Variables in Activiti Workflows
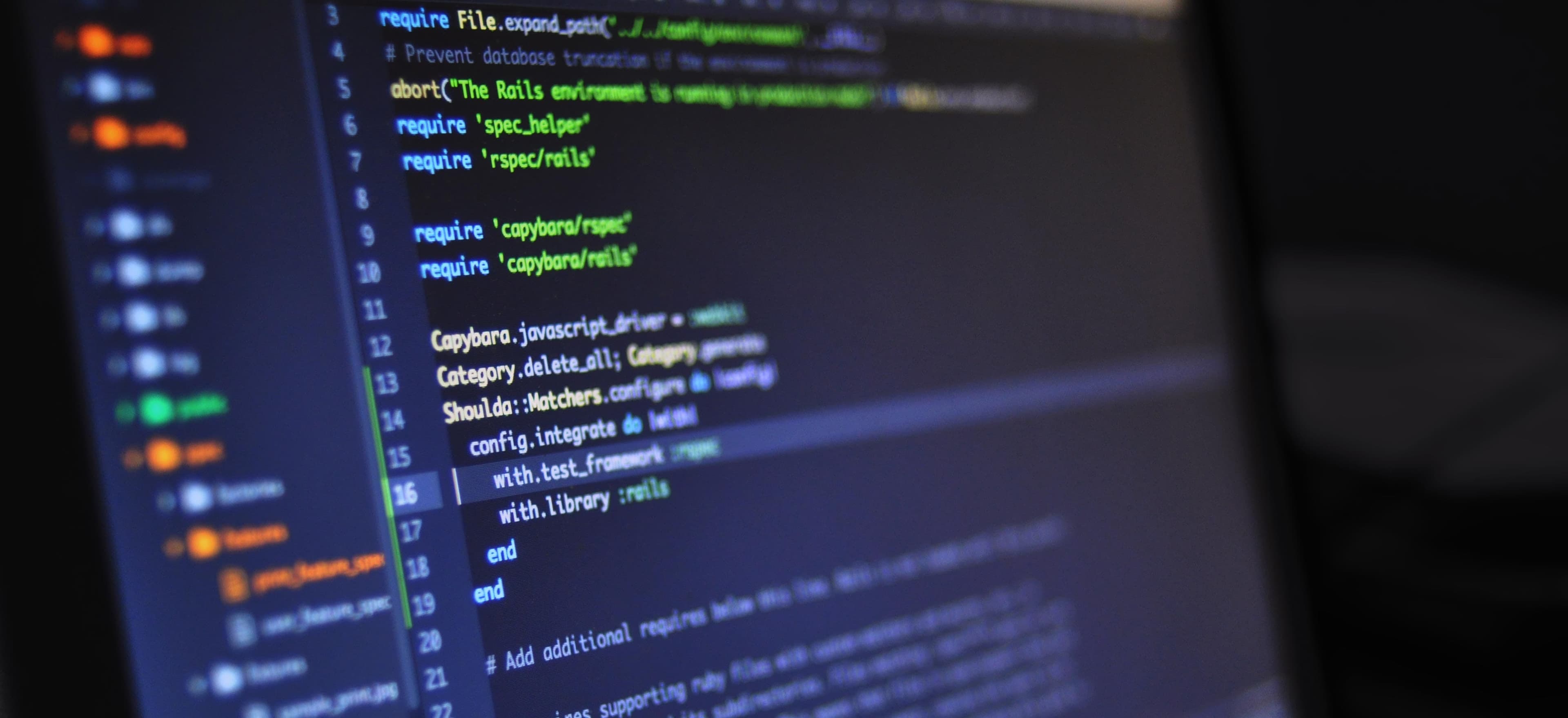
- Published on
Understanding Transient Variables in Activiti Workflows
When working with Activiti, a powerful workflow and Business Process Management (BPM) platform, developers often come across the need to manage transient variables within their workflows. Transient variables play a crucial role in passing data between different tasks in a workflow and ensuring the smooth execution of processes. In this article, we will delve into the concept of transient variables, understand their significance, and explore how to effectively manage them in Activiti workflows using Java.
What are Transient Variables?
In the context of Activiti workflows, transient variables are used to pass temporary data between different activities within a process instance. Unlike process variables, which are stored in the Activiti database and have a longer lifecycle, transient variables exist only for the duration of a single service task or user task and are not persisted in the database.
Why Use Transient Variables?
Transient variables offer several advantages in workflow management:
-
Efficient Data Passing: Transient variables facilitate the efficient passing of temporary data between different tasks, without cluttering the database with short-lived information.
-
Isolation of Task-Specific Data: By using transient variables, each task within a workflow can maintain its own isolated set of temporary data, preventing interference between different tasks.
-
Improved Performance: Since transient variables are not stored in the database, they contribute to better performance and reduced database load, especially in scenarios involving frequent data exchange between tasks.
Managing Transient Variables in Activiti Workflows using Java
Now, let's dive into the practical aspects of managing transient variables in Activiti workflows using Java. We will explore how to set transient variables during the execution of a service task and how to retrieve them in a subsequent task.
Setting Transient Variables
In the context of a JavaDelegate implementation for a service task, transient variables can be set using the DelegateExecution
object provided by Activiti. Below is an example of how to set transient variables within a service task:
public class SampleServiceTask implements JavaDelegate {
@Override
public void execute(DelegateExecution execution) {
// Setting a transient variable
execution.setTransientVariable("tempData", "sampleValue");
// Your task logic goes here
}
}
In this example, the execute
method of the JavaDelegate
implementation sets a transient variable named "tempData" with the value "sampleValue". It's important to note that when setting transient variables, the data type of the value should be serializable, as it needs to be passed between different JVMs during the workflow execution.
Retrieving Transient Variables
Once a transient variable has been set in a service task, it can be retrieved in a subsequent task within the same process instance. Here's an example of how to retrieve a transient variable in another JavaDelegate implementation:
public class AnotherServiceTask implements JavaDelegate {
@Override
public void execute(DelegateExecution execution) {
// Retrieving a transient variable
String tempData = (String) execution.getTransientVariable("tempData");
// Your task logic goes here
}
}
In this snippet, the execute
method of the JavaDelegate
implementation retrieves the transient variable "tempData" that was set earlier in a different service task. The data type of the retrieved value should match the original data type used when setting the transient variable.
The Bottom Line
In conclusion, transient variables play a crucial role in managing temporary data within Activiti workflows. By using these variables effectively, developers can ensure efficient data passing between different tasks and contribute to the overall performance and scalability of their workflow solutions.
In this article, we've discussed the concept of transient variables, their significance in workflow management, and how to manage them in Activiti workflows using Java. By understanding and harnessing the power of transient variables, developers can elevate the robustness and efficiency of their Activiti-based workflow solutions.
To further enhance your understanding of Activiti workflows, you may find it beneficial to explore Activiti's official documentation and Best Practices for Activiti BPM.
Happy workflow designing with Activiti!