Optimizing Database Performance with jOOQ in Java
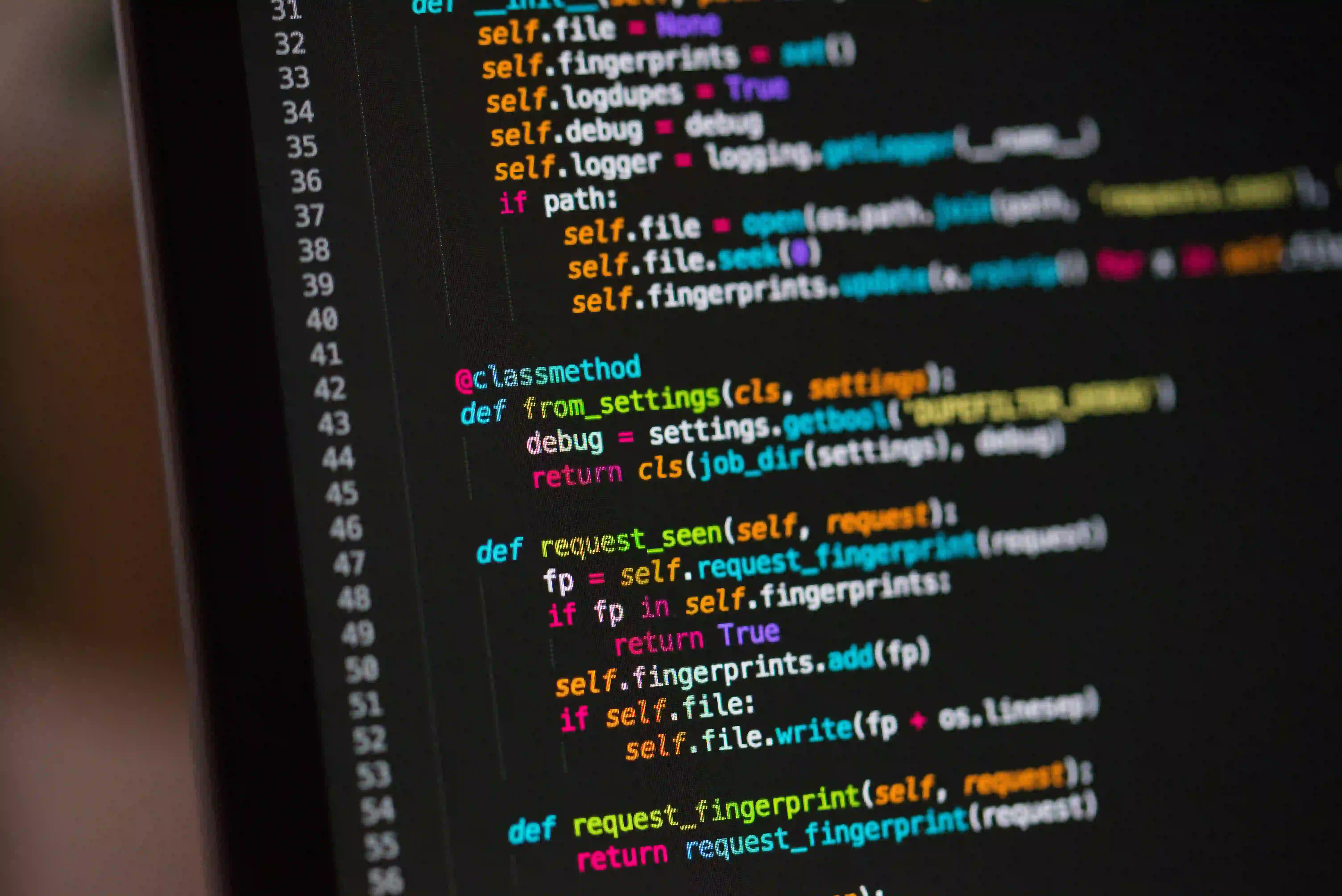
Optimizing Database Performance with jOOQ in Java
In the realm of Java development, efficient database interactions are pivotal for creating high-performing applications. Leveraging a robust and intuitive database access framework like jOOQ can significantly enhance the performance of your Java applications. In this post, we'll delve into the optimization of database performance using jOOQ in Java.
Understanding jOOQ
jOOQ is a type-safe SQL query construction framework for Java, which allows for fluent and expressive querying of a database using Java objects. By modeling the database schema as a set of Java objects, jOOQ empowers developers to write type-safe SQL queries with auto-completion and refactoring capabilities. This ultimately leads to more maintainable and performant code when interacting with the database.
Establishing Efficient Database Connections with jOOQ
One fundamental aspect of optimizing database performance is establishing efficient database connections. jOOQ facilitates this by providing a straightforward API to create and manage database connections. For instance, consider the following code snippet, where we initialize a jOOQ DSLContext
to establish a connection to the database using a DataSource
.
DSLContext context = DSL.using(dataSource, SQLDialect.POSTGRES);
Here, dataSource
represents the configured data source, and SQLDialect.POSTGRES
specifies the SQL dialect to be used. By employing jOOQ's DSLContext
, developers can seamlessly manage database connections, ensuring optimal performance and resource utilization.
Utilizing jOOQ's Query Execution Performance
Efficient query execution is paramount in optimizing database performance. jOOQ offers a diverse range of query execution strategies that can be leveraged to achieve optimal performance based on specific use cases. The following example demonstrates the execution of a simple SQL query using jOOQ:
Result<Record> result = context.select().from(USERS).where(USERS.AGE.greaterThan(25)).fetch();
In this snippet, context
represents the initialized DSLContext
, while USERS
corresponds to a jOOQ-generated table reference. By invoking the select
and where
methods in a fluent manner, jOOQ allows for concise yet powerful query construction. This streamlined approach to query execution not only enhances readability but also contributes to improved performance.
Leveraging jOOQ's Code Generation for Optimal Performance
jOOQ's code generation capabilities play a pivotal role in optimizing database performance. By generating Java classes from the existing database schema, jOOQ facilitates type-safe interaction with the database, thereby minimizing runtime errors and enhancing performance. Below is an example of using jOOQ's code generation to represent database tables as Java objects:
public class User {
private String name;
private int age;
// Getters and setters
}
By leveraging jOOQ's code generation, database entities are modeled as Java objects, enabling seamless integration of database operations within the Java codebase. This not only streamlines development but also contributes to improved performance through type safety and compile-time checks.
Optimizing Database Transactions with jOOQ
Efficient management of database transactions is crucial for ensuring optimal database performance. jOOQ simplifies transaction management by offering intuitive APIs that facilitate the execution of database operations within a transactional context. Consider the following code snippet, where a series of database operations are executed within a transaction using jOOQ:
context.transaction(configuration -> {
DSL.using(configuration)
.insertInto(USERS, USERS.NAME, USERS.AGE)
.values("John", 30)
.execute();
DSL.using(configuration)
.update(USERS)
.set(USERS.AGE, USERS.AGE.plus(1))
.where(USERS.NAME.eq("John"))
.execute();
});
Here, the transaction
method of the DSLContext
allows for the encapsulation of database operations within a transactional scope. By leveraging jOOQ's transaction management capabilities, developers can ensure data integrity while optimizing database performance.
Integrating jOOQ with Advanced Database Features
In addition to the core functionalities, jOOQ seamlessly integrates with advanced database features to enhance performance. For instance, jOOQ provides support for executing stored procedures, utilizing window functions, and leveraging vendor-specific database capabilities, contributing to optimized database interactions. The following example illustrates the execution of a stored procedure using jOOQ:
context.transaction(configuration -> {
DSL.using(configuration)
.call(DSL.createRoutine("stored_procedure"))
.execute();
});
By accommodating advanced database features, jOOQ empowers developers to harness the full potential of the underlying database, thereby optimizing performance in Java applications.
Bringing It All Together
In this post, we've explored the optimization of database performance using jOOQ in Java. By leveraging its intuitive query construction, code generation, transaction management, and support for advanced database features, jOOQ proves to be a powerful ally in enhancing the performance of Java applications. Harnessing the capabilities of jOOQ not only fosters maintainable and readable code but also contributes to the efficient execution of database interactions, ultimately leading to high-performing Java applications. Embrace jOOQ to unlock the full potential of database interactions in your Java projects and elevate their performance to new heights.