Balancing Optimization: When to Proceed Mindfully
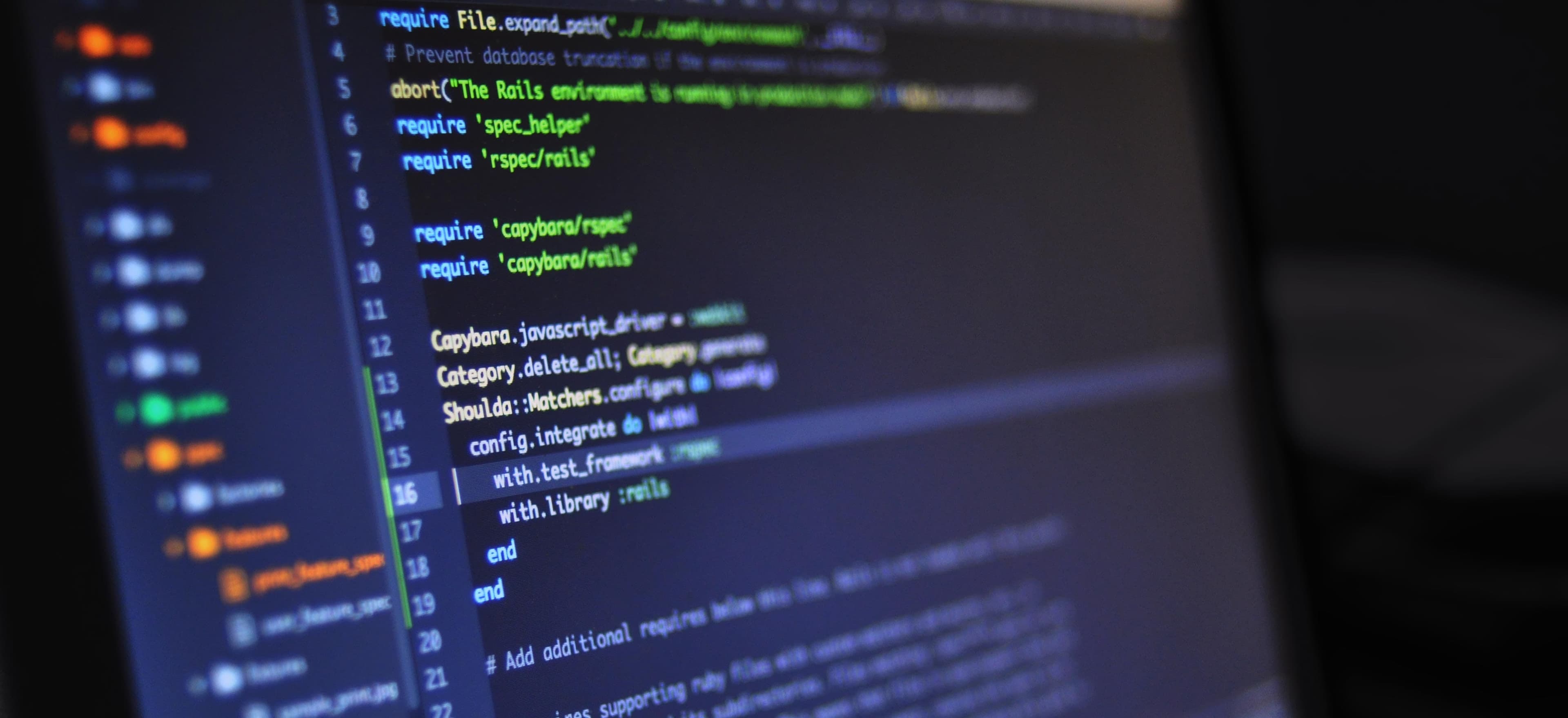
- Published on
Balancing Optimization: When to Proceed Mindfully
As a Java developer, it's crucial to strike a balance between writing clean, maintainable code and optimizing performance. While it's tempting to prioritize optimization from the onset, premature optimization can lead to convoluted, hard-to-maintain code. On the other hand, neglecting optimization until late in the development cycle can result in significant rework. In this blog post, we'll explore the art of balancing optimization in Java development, knowing when to optimize, and techniques to ensure that optimization doesn't compromise code quality.
Knowing When to Optimize
Optimization is essential, but it should be approached judiciously. Before diving into optimization, it's imperative to identify the performance bottlenecks. Profiling tools like JProfiler and YourKit can provide valuable insights into areas of code that require optimization.
In general, focus on optimization in the following scenarios:
- Measurable Performance Impact: Address performance bottlenecks only if they have a measurable impact on the application's performance. Optimizing code that has negligible performance implications can lead to diminishing returns.
- Repetitive Execution: If a piece of code is executed frequently or iterates over a large dataset, optimizing it can yield substantial performance gains.
- Scalability: Anticipating future scaling requirements can justify certain optimizations, but be cautious not to over-engineer for hypothetical scenarios.
Techniques for Mindful Optimization
1. Effective Data Structures and Algorithms
Choosing the right data structures and algorithms can significantly impact the performance of your Java applications. For instance, when dealing with large datasets, opting for efficient data structures such as HashMaps instead of ArrayLists can reduce lookup times.
Example:
// Using HashMap for efficient lookup
Map<String, Integer> studentScores = new HashMap<>();
studentScores.put("Alice", 95);
studentScores.put("Bob", 87);
int score = studentScores.get("Alice");
In this example, using a HashMap for student scores provides efficient O(1) lookup time, as opposed to iterating through a list.
2. Lazy Initialization
Applying lazy initialization can defer the creation of objects or calculation of values until the point of usage, thereby saving resources when certain components are not immediately required.
Example:
public class LazyInitializationExample {
private ExpensiveResource resource;
public ExpensiveResource getResource() {
if (resource == null) {
resource = new ExpensiveResource();
}
return resource;
}
}
In this case, the ExpensiveResource
is only created when the getResource
method is called for the first time, avoiding unnecessary resource allocation.
3. Minimize Object Creation
Frequent object creation can lead to increased memory consumption and garbage collection overhead. Reusing objects or employing object pooling can mitigate these performance drawbacks.
Example:
// Reusing StringBuilder instead of creating new instances
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 1000; i++) {
sb.setLength(0); // Clearing the StringBuilder for reuse
sb.append("Iteration ").append(i);
System.out.println(sb.toString());
}
By reusing the StringBuilder
instance, unnecessary object creations and memory allocations are avoided.
Optimization Best Practices
1. Benchmarking
Before and after making optimizations, it's crucial to benchmark the performance to objectively measure the impact of the changes. Tools like JMH (Java Microbenchmarking Harness) provide a reliable means of benchmarking Java code.
2. Profile Guided Optimization
Profiling the application under realistic workloads can reveal the hotspots that truly warrant optimization. Tools like VisualVM and Java Mission Control can aid in identifying performance bottlenecks during runtime.
3. Code Reviews and Collaboration
Encouraging code reviews and seeking input from peers can unearth optimization opportunities and ensure that the proposed optimizations align with best practices and maintainability.
Bringing It All Together
Optimization is an integral part of Java development, but it should be approached mindfully. Profiling, targeted optimizations, and adhering to best practices are essential components in achieving the delicate balance between performance and maintainability. By meticulously identifying areas that truly warrant optimization and implementing thoughtful optimizations, Java developers can yield high-performance applications without compromising code quality. Striking this balance ensures that your Java code not only runs efficiently but remains maintainable and adaptable in the long run.
In conclusion, the mantra to follow is: Measure, Mindfulness, and Maintainability. Always optimize with purpose, measure the impact, and ensure that the optimizations uphold the code's quality and maintainability.
Remember, optimize not for the sake of it, but with a clear understanding of the problem and its potential solutions.
By fostering a commitment to mindful optimization, Java developers can create robust and high-performing applications that stand the test of time.