Optimizing Database Performance with Efficient Recycle Bin Management
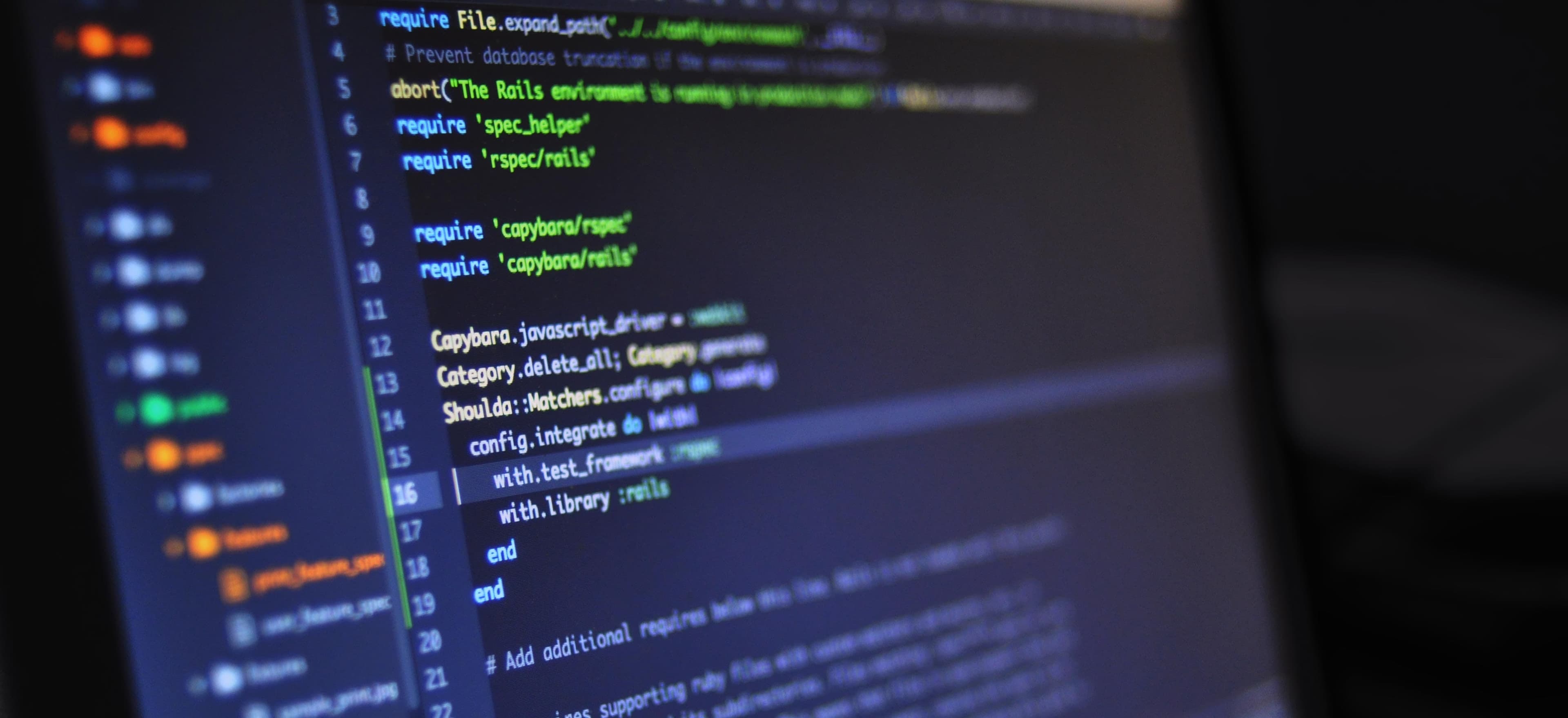
- Published on
Maximizing Database Performance with Efficient Recycle Bin Management
In a Java application, database performance is crucial for ensuring optimal user experience and overall system efficiency. One often overlooked aspect of database performance is the management of the recycle bin. The recycle bin plays a vital role in ensuring data integrity and recoverability in a database system. However, improper handling of the recycle bin can lead to performance bottlenecks and resource wastage. In this article, we will explore the importance of efficient recycle bin management and how Java developers can optimize database performance by implementing best practices for recycle bin management.
Understanding the Recycle Bin
In Oracle Database, the recycle bin is a feature that provides a way to manage dropped database objects, such as tables, indexes, and other schema objects. When an object is dropped, it is not immediately removed from the database. Instead, it is moved to the recycle bin, where it can be recovered if needed. This mechanism adds a layer of data protection by allowing accidental drops to be reversed.
Impact of Inefficient Recycle Bin Management
While the recycle bin offers invaluable benefits, inefficient management can lead to several performance issues:
-
Increased Storage Overhead: Dropped objects in the recycle bin continue to occupy storage space, potentially leading to unnecessary resource consumption.
-
Degraded Query Performance: The presence of unused objects in the recycle bin can impact query execution plans, leading to suboptimal performance.
-
I/O Overhead: The recycle bin adds overhead to various database operations, such as backup and recovery processes, impacting overall I/O performance.
Best Practices for Efficient Recycle Bin Management
To mitigate the potential performance impact of the recycle bin, Java developers can implement the following best practices:
1. Purging Unused Objects Regularly
Code Example 1: Purging recycle bin using Java
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import java.sql.Statement;
public class RecycleBinManager {
public void purgeRecycleBin(String connectionString) {
try (Connection connection = DriverManager.getConnection(connectionString)) {
Statement statement = connection.createStatement();
statement.execute("PURGE RECYCLEBIN");
} catch (SQLException e) {
// Handle exception
}
}
}
Why: Regularly purging the recycle bin removes dropped objects permanently, freeing up storage space and reducing overhead. Refer to Oracle Documentation on PURGE statement
2. Automating Recycle Bin Maintenance Tasks
Code Example 2: Automating recycle bin purging using ScheduledExecutorService
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
public class RecycleBinScheduler {
public void scheduleRecycleBinPurging(RecycleBinManager recycleBinManager) {
ScheduledExecutorService executor = Executors.newSingleThreadScheduledExecutor();
executor.scheduleAtFixedRate(recycleBinManager::purgeRecycleBin, 0, 24, TimeUnit.HOURS);
}
}
Why: Automating the purging process ensures that the recycle bin is regularly cleaned, reducing manual intervention and minimizing the risk of neglecting maintenance tasks.
3. Monitoring Recycle Bin Utilization
Code Example 3: Monitoring recycle bin utilization using Java Mission Control
import javax.management.MBeanServer;
import com.sun.management.HotSpotDiagnosticMXBean;
import java.lang.management.ManagementFactory;
public class RecycleBinMonitor {
public long getRecycleBinUtilization() {
MBeanServer server = ManagementFactory.getPlatformMBeanServer();
HotSpotDiagnosticMXBean mxBean = ManagementFactory.newPlatformMXBeanProxy(
server, "com.sun.management:type=HotSpotDiagnostic", HotSpotDiagnosticMXBean.class);
return mxBean.getCompressedClassSpaceUsed();
}
}
Why: Monitoring recycle bin utilization provides insights into the impact of dropped objects on database resources, enabling proactive management and optimization.
Final Considerations
Efficient recycle bin management is a critical component of database performance optimization. By adhering to best practices such as regular purging, automation, and monitoring, Java developers can mitigate the potential performance impact of the recycle bin and ensure a streamlined database environment. Incorporating these practices into database management strategies not only enhances performance but also contributes to overall system reliability and resource utilization.
Optimizing recycle bin management is just one aspect of database performance tuning. As Java developers, it's essential to continuously explore and implement best practices to enhance the efficiency and reliability of database systems, ultimately delivering exceptional user experiences and system scalability.
Implementing efficient recycle bin management in Java applications is a proactive step towards ensuring sustained database performance, and by extension, an enhanced overall application experience.