How to Effectively Test Abstract Classes and Template Methods
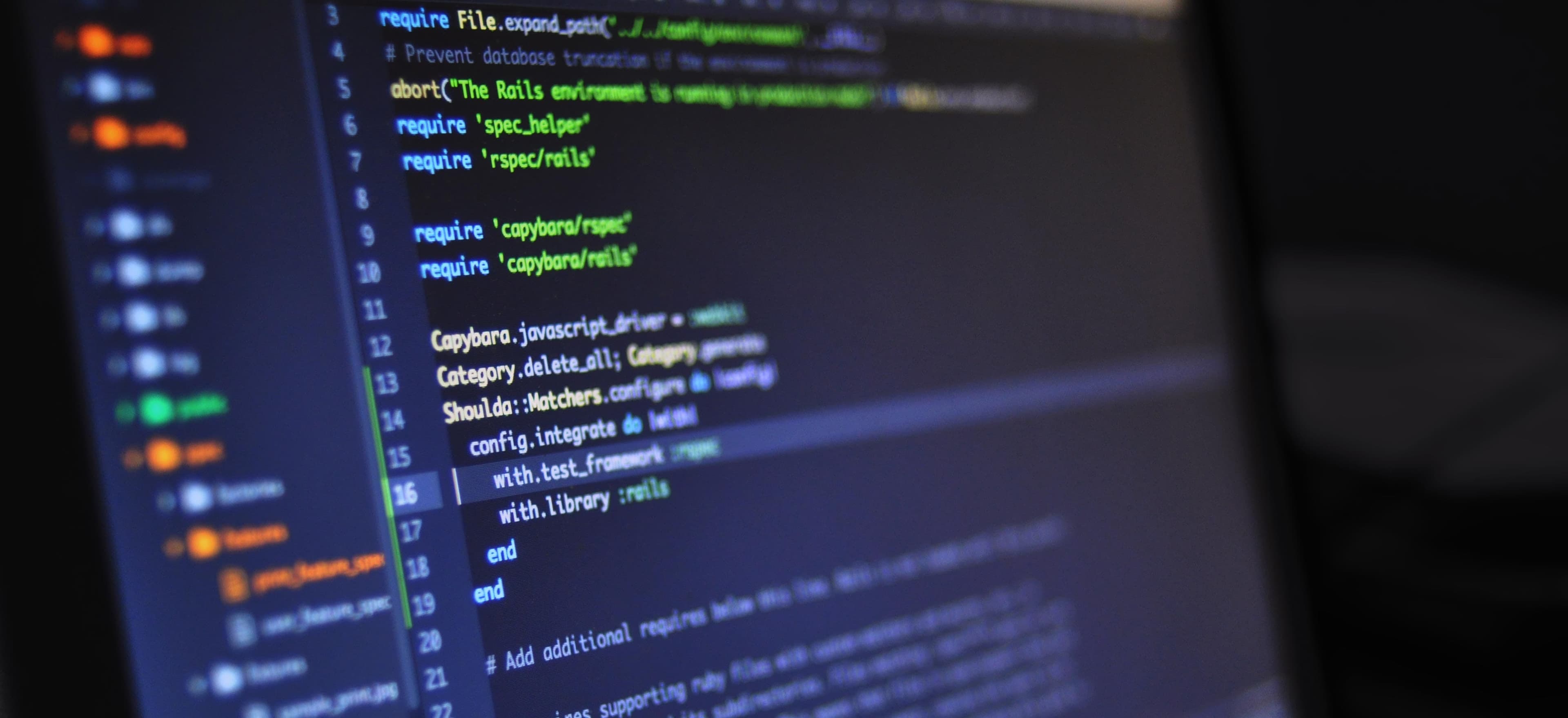
- Published on
Testing Abstract Classes and Template Methods in Java
When it comes to writing robust and maintainable Java code, testing is paramount. In the context of abstract classes and template methods, testing becomes particularly important due to the complexities involved in testing abstract classes and methods that are meant to be overridden by subclasses.
In this article, we will delve into the strategies and best practices for effectively testing abstract classes and template methods in Java.
Understanding Abstract Classes and Template Methods
Before we dive into testing, let's briefly revisit what abstract classes and template methods are in Java.
Abstract Classes
An abstract class in Java is a class that cannot be instantiated on its own and may contain abstract methods, i.e., methods without a body. Abstract classes are meant to be extended by subclasses, which provide the implementation for the abstract methods.
public abstract class AbstractShape {
public abstract double calculateArea();
}
Template Methods
Template methods are methods in an abstract class that define the structure of an algorithm but leave some steps to be implemented by subclasses. This pattern is commonly used to define a skeleton of an algorithm in the superclass but defer some implementation details to subclasses.
public abstract class AbstractShape {
// Template method
public double calculateArea() {
double[] dimensions = getDimensions();
validateDimensions(dimensions);
return calculateAreaFormula(dimensions);
}
protected abstract double[] getDimensions();
protected abstract void validateDimensions(double[] dimensions);
protected abstract double calculateAreaFormula(double[] dimensions);
}
Testing Abstract Classes and Template Methods
The Challenge
When testing abstract classes and template methods, the challenge lies in effectively testing the behavior of the abstract methods and ensuring that the template method executes the expected algorithm while allowing for variations in the implementation provided by subclasses.
Testing Strategies
1. Testing Concrete Methods
Even though abstract classes contain abstract methods, they can also contain concrete (non-abstract) methods. Testing these concrete methods can provide valuable insights into the behavior of the class.
Example:
public abstract class AbstractShape {
public double calculatePerimeter() {
double[] dimensions = getDimensions();
return calculatePerimeterFormula(dimensions);
}
protected abstract double[] getDimensions();
protected abstract double calculatePerimeterFormula(double[] dimensions);
}
Testing the calculatePerimeter
method involves providing sample dimensions, mocking the behavior of abstract methods, and asserting the expected perimeter value.
2. Testing Template Methods
Testing the template method involves verifying that the algorithm defined in the template method behaves as expected and that the abstract methods are appropriately called.
Example:
public class Circle extends AbstractShape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
protected double[] getDimensions() {
return new double[]{radius};
}
@Override
protected void validateDimensions(double[] dimensions) {
if (dimensions.length != 1 || dimensions[0] <= 0) {
throw new IllegalArgumentException("Invalid dimensions for circle: " + Arrays.toString(dimensions));
}
}
@Override
protected double calculateAreaFormula(double[] dimensions) {
return Math.PI * Math.pow(dimensions[0], 2);
}
@Override
protected double calculatePerimeterFormula(double[] dimensions) {
return 2 * Math.PI * dimensions[0];
}
}
public class CircleTest {
@Test
public void testCalculateArea() {
Circle circle = new Circle(5);
assertEquals(78.539, circle.calculateArea(), 0.001);
}
@Test
public void testCalculatePerimeter() {
Circle circle = new Circle(5);
assertEquals(31.416, circle.calculatePerimeter(), 0.001);
}
}
In this example, we test the behavior of the calculateArea
and calculatePerimeter
methods of the Circle
class, which extends the AbstractShape
class.
3. Using Mocking Frameworks
When testing abstract classes with complex dependencies or interactions, using mocking frameworks such as Mockito can be beneficial. Mocking allows the behavior of abstract methods or external dependencies to be controlled and verified.
Example:
public abstract class AbstractShapeService {
private AbstractShapeDao shapeDao;
public AbstractShapeService(AbstractShapeDao shapeDao) {
this.shapeDao = shapeDao;
}
public double calculateTotalArea() {
List<AbstractShape> shapes = shapeDao.getShapes();
double totalArea = 0;
for (AbstractShape shape : shapes) {
totalArea += shape.calculateArea();
}
return totalArea;
}
}
public class AbstractShapeServiceTest {
@Test
public void testCalculateTotalArea() {
AbstractShapeDao mockedShapeDao = Mockito.mock(AbstractShapeDao.class);
when(mockedShapeDao.getShapes()).thenReturn(Arrays.asList(new Circle(5), new Rectangle(4, 6)));
AbstractShapeService shapeService = new AbstractShapeService(mockedShapeDao);
assertEquals(109.539, shapeService.calculateTotalArea(), 0.001);
}
}
In this example, we use Mockito to mock the behavior of the AbstractShapeDao
and test the calculateTotalArea
method of the AbstractShapeService
, ensuring that it accurately calculates the total area of shapes obtained from the data source.
In Conclusion, Here is What Matters
Testing abstract classes and template methods in Java requires a combination of traditional unit testing techniques, mocking, and careful attention to the behavior of concrete and abstract methods. By employing the strategies discussed in this article, developers can ensure that their abstract classes and template methods are thoroughly tested for correct behavior and maintainability.
Remember, testing is not just about asserting functionality; it's also about ensuring the stability and longevity of your codebase.
For additional insights on testing in Java, you can explore the JUnit documentation and Mockito documentation to further enhance your testing skills.
Keep testing, keep coding, and keep innovating!