Optimizing Performance: Solving Common Application Server Bottlenecks
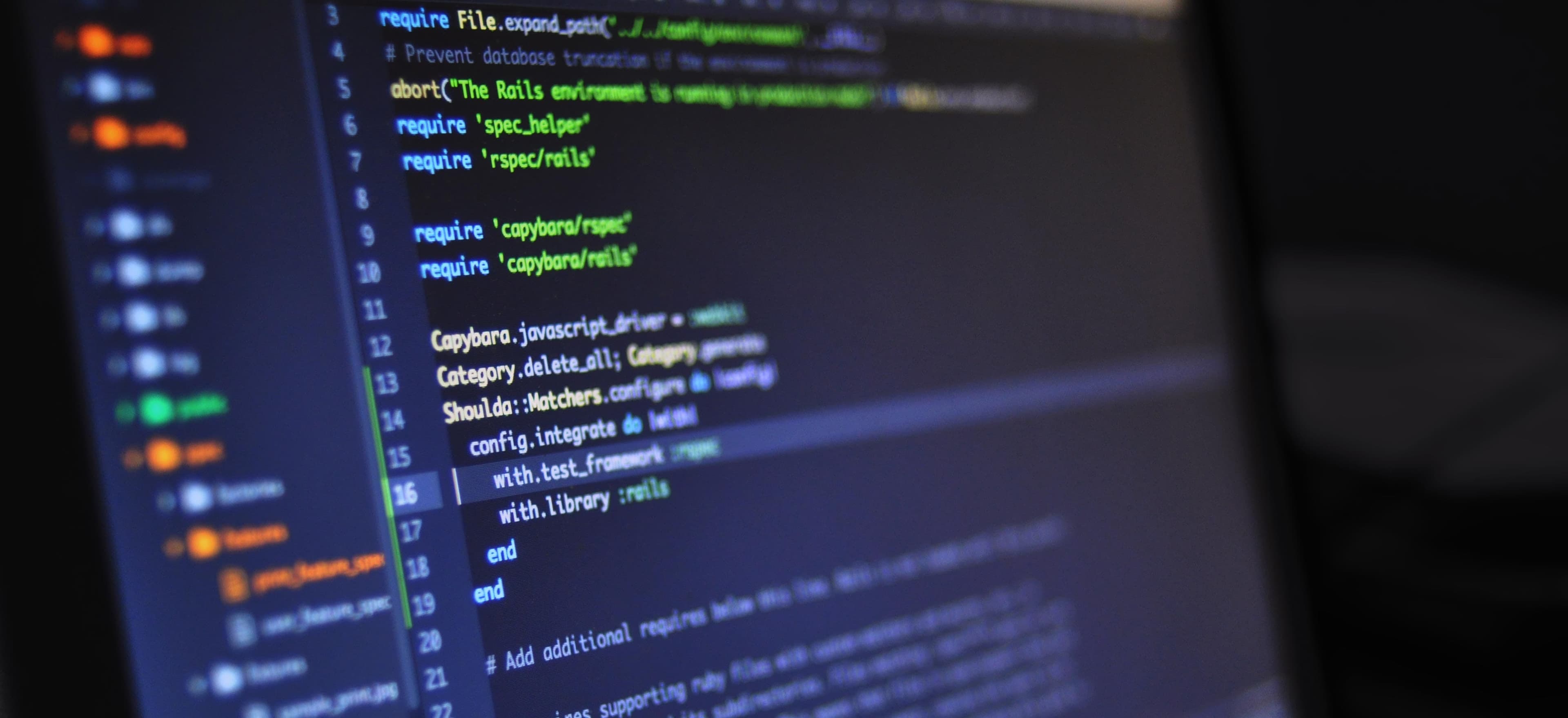
- Published on
Optimizing Performance: Solving Common Application Server Bottlenecks
In the realm of Java application development, ensuring optimal performance is a crucial aspect of delivering a successful product. Application servers play a pivotal role in the performance of Java applications and several common bottlenecks can hinder their efficiency. In this article, we will explore some of the most prevalent performance bottlenecks in Java application servers and discuss strategies to effectively address them.
Understanding Performance Bottlenecks
Performance bottlenecks in application servers can manifest in various forms, such as slow response times, high CPU or memory utilization, and inefficient handling of concurrent requests. Identifying and resolving these bottlenecks is essential for delivering a responsive and scalable application.
Let's delve into some of the common performance bottlenecks and learn how to mitigate them.
Thread Management
In a Java application server, inefficient thread management can significantly impact performance. When the server is unable to effectively manage threads to handle incoming requests, it can lead to thread contention, increased response times, and even thread exhaustion.
public class ThreadManagementExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(10);
for (int i = 0; i < 100; i++) {
executor.execute(() -> {
// Task execution logic
});
}
executor.shutdown();
}
}
In the above example, we use Executors.newFixedThreadPool
to create a fixed-size thread pool, which helps in managing threads efficiently. By controlling the number of threads, we can avoid potential thread exhaustion and contention, thereby optimizing performance.
Memory Leaks
Memory leaks are notorious for causing performance degradation in Java applications. In the context of application servers, inefficient memory management can result in excessive garbage collection, increased memory utilization, and ultimately, degraded performance.
public class MemoryLeakExample {
private static List<Object> memoryLeakList = new ArrayList<>();
public void addToMemoryLeakList(Object obj) {
memoryLeakList.add(obj);
}
}
In the above example, the MemoryLeakExample
class maintains a static list memoryLeakList
to which objects are continuously added without removal. This can lead to a memory leak, as objects are held in memory indefinitely, causing increased memory consumption. Implementing proper object lifecycle management and ensuring timely cleanup can mitigate such memory leaks.
Database Connection Management
Effective management of database connections is crucial for maintaining optimal performance in Java application servers. Inefficient handling of database connections, such as not releasing connections after use or exceeding connection pool limits, can lead to contention, reduced throughput, and increased response times.
public class DatabaseConnectionExample {
public void performDatabaseOperation() {
DataSource dataSource = getDataSource(); // Obtain the data source
try (Connection connection = dataSource.getConnection()) {
// Execute database operations
} catch (SQLException ex) {
// Exception handling
}
}
}
In the above example, the performDatabaseOperation
method demonstrates the use of try-with-resources to ensure proper management of database connections. By utilizing try-with-resources, we guarantee that the connection is automatically closed after its use, preventing connection leaks and optimizing resource utilization.
Inefficient Caching
While caching is instrumental in improving application performance, improper caching strategies can lead to inefficiency and performance bottlenecks. Inadequate cache size, excessive cache misses, and outdated caching policies can diminish the advantages of caching and impact overall application performance.
public class InefficientCachingExample {
private CacheManager cacheManager;
public Object getCachedData(String key) {
Cache cache = cacheManager.getCache("dataCache");
Element element = cache.get(key);
if (element == null) {
// Fetch data from source and add to cache
}
return element.getObjectValue();
}
}
In the above example, the getCachedData
method retrieves data from the cache and fetches from the source if the data is not present in the cache. However, the absence of proper cache eviction policies and size management can lead to inefficient use of memory and diminished caching benefits. Implementing an appropriate cache eviction strategy and monitoring cache utilization can effectively address this bottleneck.
The Bottom Line
In conclusion, optimizing the performance of Java application servers involves identifying and addressing common bottlenecks such as inefficient thread management, memory leaks, database connection management, and inefficient caching. By implementing effective mitigation strategies, such as proper thread pool management, memory cleanup, efficient database connection handling, and optimized caching policies, developers can significantly enhance the performance and scalability of their Java applications.
By recognizing and resolving these performance bottlenecks, Java developers can ensure that their application servers operate at peak efficiency, delivering a seamless and responsive user experience.
For further insights into optimizing Java application performance, check out Java Performance Optimization: 10 Tips and Tricks for valuable techniques and best practices.
Remember, a well-optimized application server is not only a testament to your development prowess but also a cornerstone for delivering exceptional user experiences.
Let's code efficiently and keep our applications performing at their best!