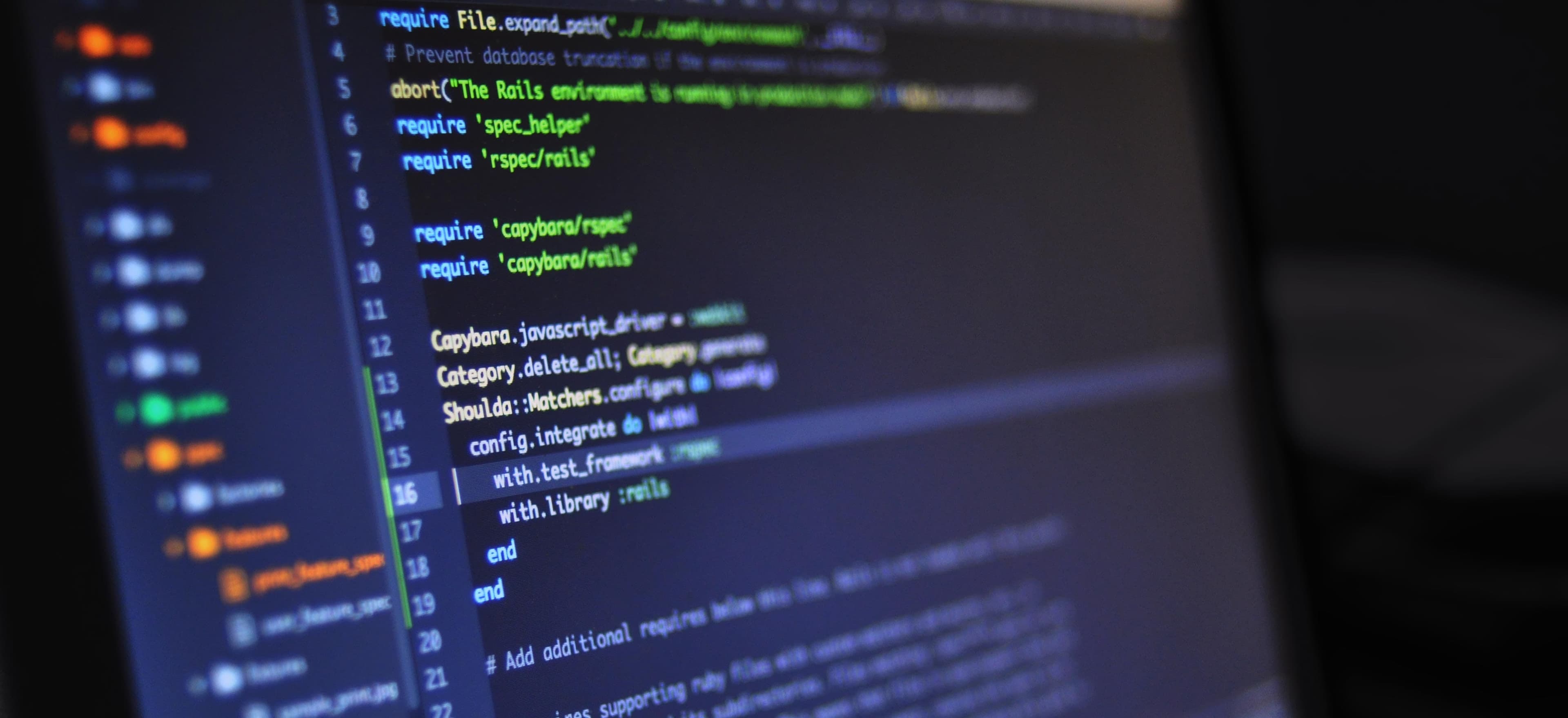
- Published on
Java EE 6 vs Spring 3: Java EE Hassles
When it comes to developing enterprise-level applications in Java, the choice of framework plays a critical role. Java EE 6 and Spring 3 are two popular choices, each with its own set of features, benefits, and limitations. In this post, we'll explore the hassles associated with Java EE 6 and how Spring 3 addresses them effectively.
The Hassles of Java EE 6
Cumbersome Configuration
Java EE 6 often requires verbose XML configuration, leading to a lot of boilerplate code. This can make the application configuration complex and hard to maintain. For instance, setting up a simple data source can involve several XML configurations spread across multiple files.
Tight Coupling and Dependency Injection Limitations
Java EE 6 can suffer from tight coupling, making it challenging to test components in isolation. Dependency Injection in Java EE 6 is limited to EJB and CDI, which can lead to difficulties in managing dependencies and mocking objects for testing.
Lack of Flexibility
Java EE 6 can be rigid in its approach, limiting the flexibility to customize certain aspects of the application. This can hinder developers from implementing certain design patterns and architectural choices, potentially impacting the overall quality and performance of the application.
Enter Spring 3
Simplified Configuration with Annotation-based Approach
Spring 3 simplifies configuration by using annotations such as @Autowired, @Component, @Service, and @Repository. This reduces the need for extensive XML configurations, leading to more concise and readable code. For example, setting up a data source in Spring requires minimal XML configuration when using the @Component and @Autowired annotations.
@Component
public class DataSourceConfig {
@Autowired
private DataSource dataSource;
// other methods and configurations
}
Loose Coupling and Enhanced Dependency Injection
Spring 3 promotes loose coupling and provides a robust dependency injection mechanism. With features like constructor injection, setter injection, and autowiring, Spring 3 allows for easier testing and a more modular approach to application design.
@Service
public class UserService {
private UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
// other methods
}
Increased Flexibility with Aspect-Oriented Programming (AOP) Support
Spring 3 offers comprehensive support for Aspect-Oriented Programming (AOP), enabling developers to modularize cross-cutting concerns such as logging, security, and transaction management. This flexibility empowers developers to encapsulate these concerns separately, promoting cleaner and more maintainable code.
Final Thoughts
While Java EE 6 is a robust enterprise framework, it comes with its own set of hassles that can hinder development and maintenance. Spring 3, on the other hand, addresses these hassles with its simplified configuration, enhanced dependency injection, and increased flexibility through AOP support.
In today's rapidly evolving enterprise application landscape, it's essential to choose a framework that not only streamlines development but also promotes best practices and maintainability. For many developers, Spring 3 has become the go-to choice for overcoming the hassles associated with Java EE 6 and delivering high-quality, scalable enterprise applications.
By leveraging the strengths of Spring 3, developers can tackle the complexities of enterprise application development with confidence, knowing they have a framework that addresses the hassles of Java EE 6 effectively.
In conclusion, while both Java EE 6 and Spring 3 have their merits, Spring 3's ability to simplify configuration, enhance dependency injection, and provide increased flexibility through AOP support makes it a compelling choice for enterprise application development.
To delve deeper into the differences between Java EE 6 and Spring 3, explore the following resources:
With the right framework at hand, the hassles become opportunities for innovation and efficiency in Java enterprise application development.