Efficient Java EE and Camel Integration Best Practices
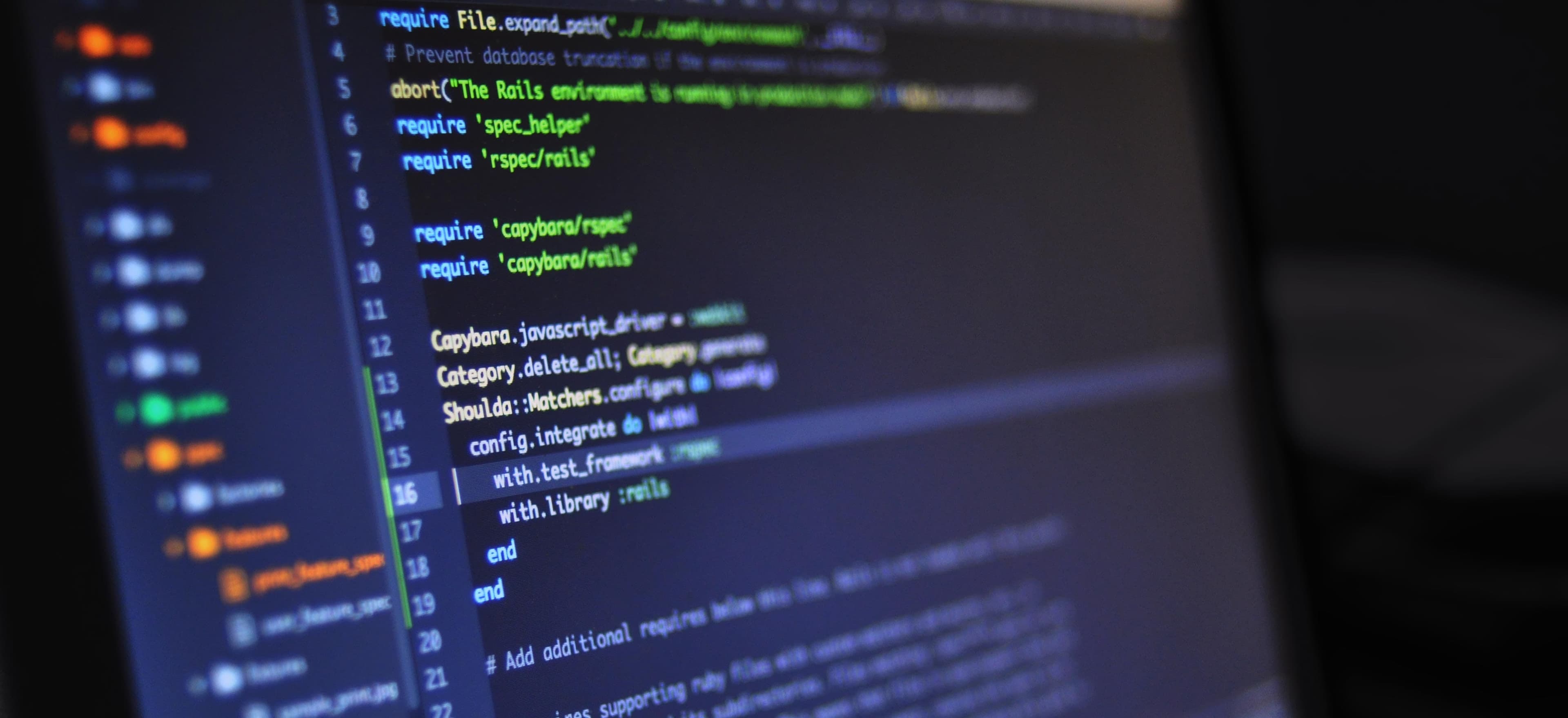
- Published on
Efficient Java EE and Camel Integration Best Practices
In the world of enterprise application development, integrating different systems and technologies is a common challenge. Apache Camel, a powerful open-source integration framework, and Java EE, a widely-used platform for building enterprise applications, are often used in combination to meet these integration needs. In this article, we will explore some best practices for integrating Apache Camel with Java EE to develop efficient and maintainable enterprise applications.
Why Integrate Apache Camel with Java EE?
Before we delve into best practices, it's important to understand the rationale behind integrating Apache Camel with Java EE. Apache Camel provides a versatile and expressive domain-specific language (DSL) for integration patterns, making it an ideal choice for implementing complex routing and mediation rules. On the other hand, Java EE offers a robust platform for building enterprise applications, providing features such as dependency injection, transaction management, and enterprise messaging.
By integrating Apache Camel with Java EE, developers can leverage the strengths of both frameworks. Camel can handle the integration aspects, while Java EE provides a solid foundation for building and deploying enterprise-grade applications.
Best Practices for Integrating Apache Camel with Java EE
1. Use Dependency Injection for Camel Context
When integrating Apache Camel with Java EE, it's crucial to utilize dependency injection for the Camel context. By doing so, you can benefit from the inversion of control provided by the Java EE container, allowing the container to manage the lifecycle of the Camel context. This promotes a more modular and maintainable architecture, as well as facilitating proper resource management within the Java EE environment.
import org.apache.camel.CamelContext;
import javax.inject.Inject;
public class MyCamelRoute {
@Inject
private CamelContext camelContext;
// Camel route implementation
}
2. Leverage Java EE Transactions
In enterprise applications, transaction management is of paramount importance. When integrating Apache Camel with Java EE, it's recommended to make use of Java EE's transaction management capabilities. By leveraging container-managed transactions, you can ensure data integrity and consistency across the integrated systems. This is particularly critical when dealing with message-driven or batch processing routes in Camel.
import org.apache.camel.builder.RouteBuilder;
import javax.transaction.Transactional;
@Transactional
public class MyCamelRoute extends RouteBuilder {
@Override
public void configure() throws Exception {
// Route configuration
}
}
3. Employ JMS for Asynchronous Messaging
Asynchronous messaging is a cornerstone of enterprise integration, enabling systems to communicate in a decoupled manner. Java EE provides support for Java Message Service (JMS), a robust messaging standard. When integrating Apache Camel with Java EE, consider using JMS for asynchronous communication between components. Camel's JMS component offers seamless integration with Java EE's JMS resources, allowing for reliable and scalable messaging within the enterprise application.
from("jms:queue:inputQueue")
.to("bean:processDataBean")
.to("jms:queue:outputQueue");
4. Implement Error Handling and Logging
Error handling and logging are integral parts of any robust integration solution. When integrating Apache Camel with Java EE, it's imperative to implement comprehensive error handling strategies and logging mechanisms. Camel's error handling capabilities, such as the onException
DSL, can be combined with Java EE's logging facilities to ensure that errors and exceptions are appropriately handled and logged within the enterprise application.
onException(Exception.class)
.handled(true)
.log("Error processing message: ${body}")
.to("jms:queue:errorQueue");
5. Ensure Scalability and Performance
Scalability and performance are critical considerations in enterprise integration. When integrating Apache Camel with Java EE, strive to design scalable and performant routes. Utilize Camel's multithreading and asynchronous processing capabilities, combined with Java EE's resource management features, to achieve optimal scalability and performance. Additionally, consider employing enterprise integration patterns such as throttling and load balancing to ensure efficient utilization of resources.
from("jms:queue:inputQueue")
.threads(5)
.to("bean:processDataBean");
The Closing Argument
Integrating Apache Camel with Java EE offers a powerful combination for developing efficient and maintainable enterprise applications. By adhering to best practices such as utilizing dependency injection, leveraging Java EE transactions, employing JMS for asynchronous messaging, implementing error handling and logging, and ensuring scalability and performance, developers can build robust integration solutions that meet the demands of the modern enterprise environment.
In conclusion, the seamless integration of Apache Camel with Java EE empowers developers to tackle complex integration challenges while leveraging the strengths of both frameworks. By following best practices and harnessing the capabilities of these technologies, organizations can achieve efficient and resilient enterprise integration solutions.