Servlet Basic Authentication Issue in OSGi Environment
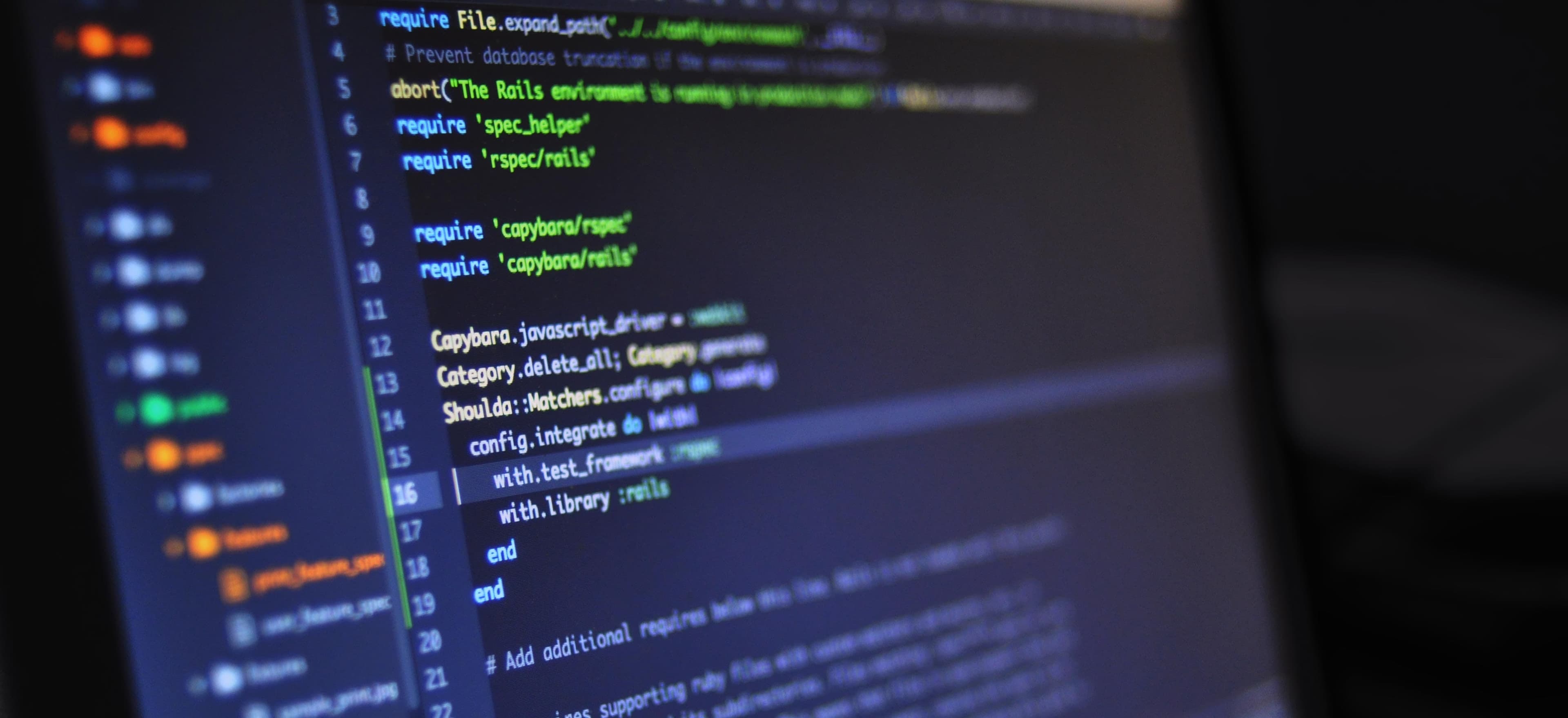
- Published on
Understanding Servlet Basic Authentication Issue in OSGi Environment
When working with Java applications in an OSGi (Open Service Gateway Initiative) environment, it's essential to have a clear understanding of how servlets and basic authentication work. In this blog post, we'll explore the challenges developers may face when implementing servlet basic authentication in an OSGi environment and discuss potential solutions to address these issues.
A Quick Look to Servlet Basic Authentication
Servlet basic authentication is a popular method for securing web applications by prompting users to enter their credentials (username and password) when accessing protected resources. It relies on the Authorization
header sent by the client in the form of Basic base64(username:password)
.
In a traditional Java web application, configuring servlet basic authentication involves defining security constraints in the web.xml
deployment descriptor and specifying the authentication method and roles required to access certain resources.
Challenges in OSGi Environment
In an OSGi environment, where applications are composed of modular and dynamic components, implementing servlet basic authentication can pose several challenges.
Class Loading and Visibility
One of the primary challenges in OSGi is class loading and visibility. Each bundle in OSGi has its own classloader, creating isolation between bundles. This isolation can lead to issues when trying to access classes or resources required for basic authentication across different bundles.
Service Registration and Discovery
Another challenge arises from service registration and discovery. The modular nature of OSGi applications means that servlets and authentication-related services may be provided by different bundles. Coordinating the registration and discovery of these services in a dynamic environment adds complexity to implementing basic authentication.
Addressing the Challenges
To address the challenges of implementing servlet basic authentication in an OSGi environment, developers can consider the following approaches:
Using OSGi Service Tracker
One way to handle service registration and discovery is by leveraging the OSGi ServiceTracker
class. This allows bundles to track the availability of specific services and react accordingly. When implementing servlet basic authentication, a ServiceTracker
can be used to dynamically discover and bind authentication-related services provided by other bundles.
ServiceTracker<AuthenticationService, AuthenticationService> tracker = new ServiceTracker<>(context, AuthenticationService.class, null);
tracker.open();
AuthenticationService authService = tracker.getService();
OSGi Service Registry Hooks
Another approach involves using OSGi service registry hooks to customize the behavior of service registrations. By intercepting the registration process, developers can enforce the proper registration of authentication-related services and ensure their availability to servlets requiring authentication.
ServiceRegistryHook hook = new AuthenticationHook();
ServiceObjects<AuthenticationService> authService = hook.getServiceObject(AuthenticationService.class);
Key Takeaways
In conclusion, implementing servlet basic authentication in an OSGi environment requires careful consideration of class loading, service registration, and discovery. By understanding the unique challenges posed by OSGi and utilizing appropriate OSGi mechanisms, developers can effectively implement servlet basic authentication in their modular Java applications.
For further reading on OSGi and servlet development, check out OSGi Alliance and Oracle's Java Servlet documentation for additional insights.
Implementing servlet basic authentication in OSGi may seem daunting at first, but with the right approach and understanding, developers can successfully overcome these challenges and ensure secure access to their web resources.