Handling Secure WCF SOAP Service Invocation
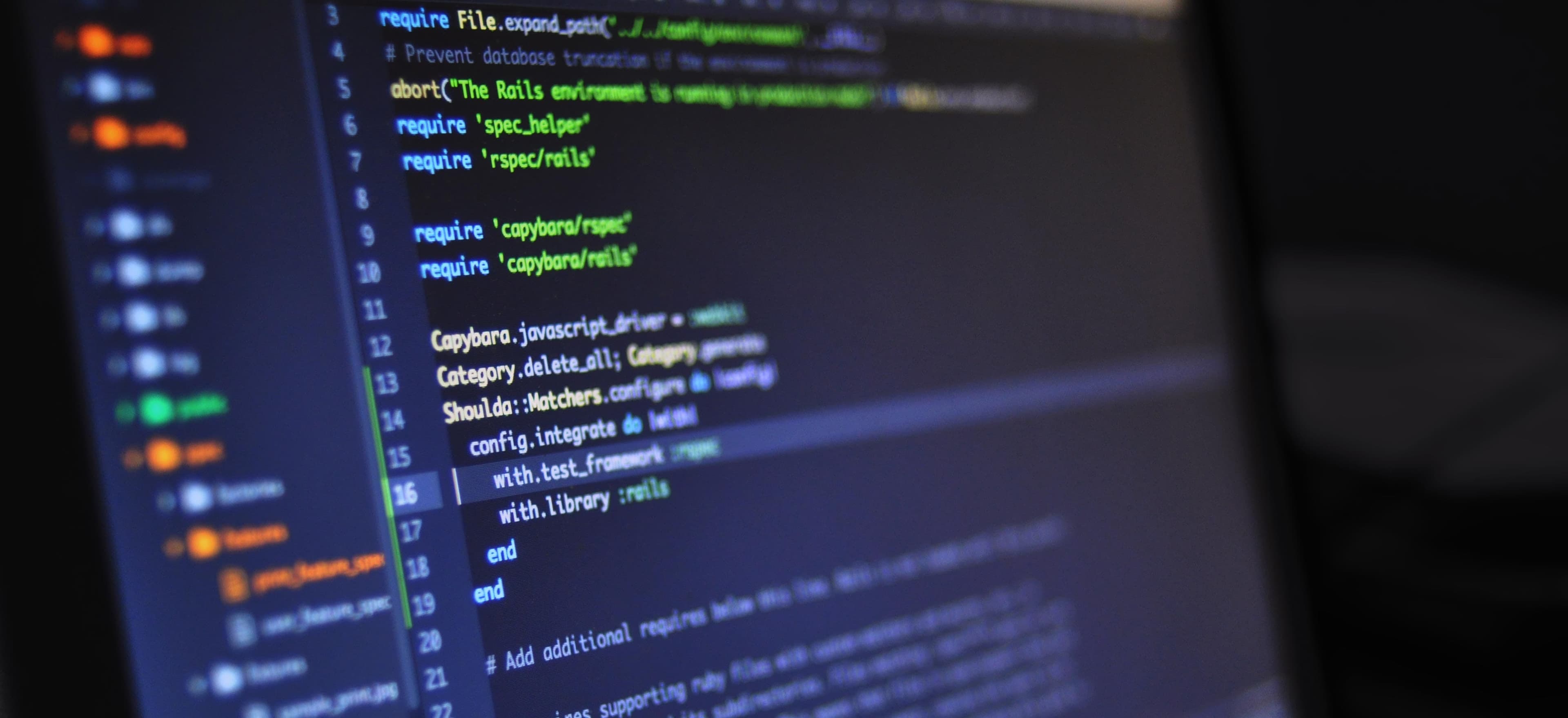
- Published on
Securing WCF SOAP Service Invocation in Java
When dealing with secure communication between client and server in Java, we often encounter the need to invoke WCF SOAP services. However, this can be a bit tricky, especially when it comes to authentication, encryption, and ensuring data integrity. In this post, we'll dive into the process of securely invoking WCF SOAP services in Java, discussing authentication, message encryption, and the overall best practices.
Setting Up the Environment
Before we begin, it's important to have the necessary tools for working with WCF SOAP services in Java. In order to handle SOAP requests and responses, we'll be using the Spring Web Services framework, which provides comprehensive support for interacting with SOAP-based services. Additionally, for secure communication, we can utilize the Spring Web Services Security module to configure the required security features.
Authentication
First and foremost, let's tackle the issue of authentication. When invoking a WCF SOAP service, it's crucial to establish the client's identity. This is commonly achieved through the use of security tokens, such as username tokens or X.509 certificates, which are sent along with the SOAP message to authenticate the client.
Username Token Authentication
public class WebServiceMessageSenderWithUsernameToken extends WebServiceMessageSenderDecorator {
private final UsernamePasswordCredentials credentials;
public WebServiceMessageSenderWithUsernameToken(WebServiceMessageSender messageSender, String username, String password) {
super(messageSender);
this.credentials = new UsernamePasswordCredentials(username, password);
}
@Override
public void send(MessageContext messageContext) throws WebServiceClientException {
WebServiceMessage request = messageContext.getRequest();
// Attach the credentials to the SOAP message
// ...
super.send(messageContext);
}
}
In the code above, we create a custom WebServiceMessageSender
that decorates the original sender with the client's username and password, effectively adding the necessary credentials to the SOAP message.
X.509 Certificate Authentication
When dealing with X.509 certificate authentication, we need to configure our Spring Web Service client to utilize the client's certificate when making requests to the WCF SOAP service. This involves setting up a keystores and configuring the WebServiceTemplate
to utilize the specified keystore for client authentication.
Message Encryption
Once we have established the client's identity through authentication, the next step is to ensure that the communication is encrypted to protect the confidentiality and integrity of the data being transmitted.
public class Wss4jSecurityInterceptorConfig extends Wss4jSecurityInterceptor {
public Wss4jSecurityInterceptorConfig() {
setSecurementActions("UsernameToken Timestamp Encrypt");
setSecurementEncryptionUser("server_public_key_alias");
setSecurementUsername("client_username");
setSecurementPassword("client_password");
}
}
In the snippet above, we configure a Wss4jSecurityInterceptor
to apply security actions including username token, timestamp, and encryption to the outgoing SOAP message. This ensures that the message is encrypted using the server's public key, providing confidentiality and integrity guarantees.
Best Practices
Adhering to best practices is crucial when dealing with secure communication. Some essential best practices include:
- Use TLS/SSL: Transport Layer Security (TLS) or Secure Sockets Layer (SSL) should be utilized to establish a secure communication channel between the client and server.
- Implement Proper Error Handling: Gracefully handling security-related errors and exceptions is a must. This includes scenarios such as authentication failures, decryption errors, and certificate validation issues.
- Keep Libraries Updated: Regularly update the libraries and frameworks used for secure communication to mitigate potential vulnerabilities and exploits.
My Closing Thoughts on the Matter
In this post, we've delved into the realm of securely invoking WCF SOAP services in Java. We covered authentication, message encryption, and highlighted the best practices to follow when dealing with secure communication. By leveraging the capabilities of Spring Web Services and its security module, Java developers can confidently handle the intricacies of WCF SOAP service invocation while maintaining the highest standards of security.
For additional information on securing SOAP web services using Spring, you can refer to the official documentation here.
Now that you've learned how to handle secure WCF SOAP service invocation, it's time to put this knowledge into practice and ensure the security of your Java applications when interacting with WCF services!