Optimizing Master-Detail CRUD Operations
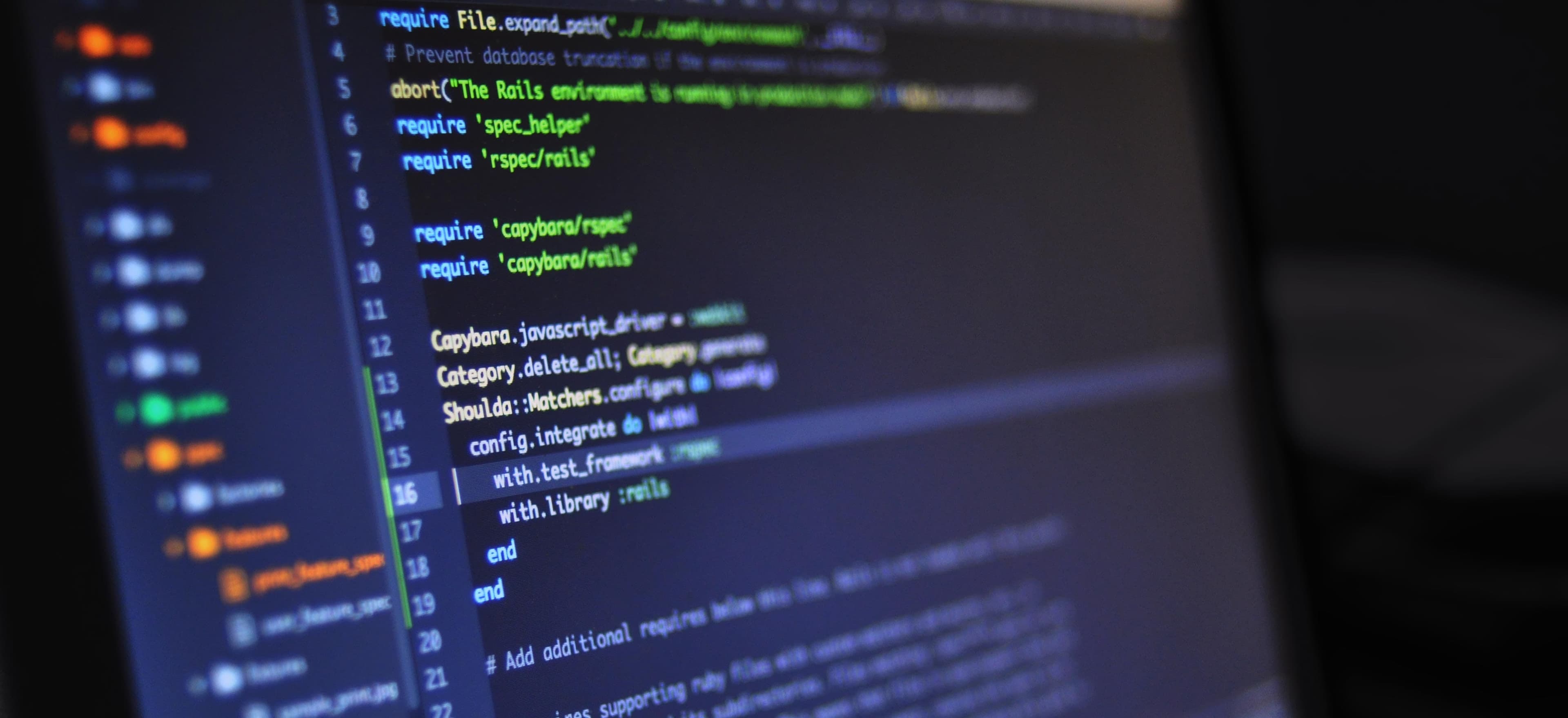
- Published on
Master-Detail CRUD Operations in Java: Optimizing Performance and Scalability
In the world of enterprise application development, creating and optimizing master-detail CRUD (Create, Read, Update, Delete) operations is crucial. This process involves managing the relationship between a master entity and its associated detail entities, and it's important to ensure that these operations are not only efficient but also scalable.
In this article, we'll explore best practices for optimizing master-detail CRUD operations in Java, focusing on performance, scalability, and maintainability. We'll cover various techniques and strategies that help in achieving efficient and scalable solutions, using Java as the primary programming language.
Understanding Master-Detail CRUD Operations
Master-detail CRUD operations involve managing the relationship between a parent (master) entity and its child (detail) entities. For example, in a typical e-commerce application, managing orders (master) and their line items (detail) would be a classic example of master-detail CRUD operations.
Optimizing Read Operations
Use Lazy Loading for Detail Entities
When dealing with large datasets, it's essential to use lazy loading for detail entities to avoid fetching all the associated details at once. Hibernate, a popular Java ORM framework, provides support for lazy loading through its association fetching strategies. By fetching detail entities lazily, we can minimize the amount of data retrieved from the database, improving the overall performance of read operations.
@Entity
public class MasterEntity {
// ...
@OneToMany(mappedBy = "master", fetch = FetchType.LAZY)
private List<DetailEntity> details;
// ...
}
Employ Caching Mechanisms
Utilize caching mechanisms such as Hibernate's second-level cache or a distributed caching solution like Redis to cache frequently accessed master and detail entities. Caching helps in reducing the number of database queries and improves the response time for read operations.
@Cacheable
@Entity
public class MasterEntity {
// ...
}
@Cacheable
@Entity
public class DetailEntity {
// ...
}
Optimizing Write Operations
Batch Insertion and Updates
When dealing with bulk inserts or updates of detail entities, consider using batch processing to minimize the number of database round trips. JPA provides the EntityManager
interface, which supports batch operations, allowing efficient insertion and updates of detail entities in bulk.
EntityManager entityManager = entityManagerFactory.createEntityManager();
EntityTransaction transaction = entityManager.getTransaction();
transaction.begin();
// Perform batch insert/update operations
transaction.commit();
entityManager.close();
Use Cascading and Orphan Removal
Utilize cascading operations to propagate state changes from the master entity to its associated detail entities. Additionally, enable orphan removal to automatically delete detail entities when they are removed from the master entity's collection. This minimizes the manual intervention required for managing the lifecycle of detail entities.
@Entity
public class MasterEntity {
// ...
@OneToMany(mappedBy = "master", cascade = CascadeType.ALL, orphanRemoval = true)
private List<DetailEntity> details;
// ...
}
Optimizing Delete Operations
Soft Deletion
Implement a soft deletion mechanism by introducing a flag in the detail entity to mark it as deleted instead of physically removing it from the database. This approach helps in maintaining data integrity and audit trails while avoiding the overhead of cascading delete operations.
@Entity
public class DetailEntity {
// ...
@Column(name = "deleted")
private boolean deleted;
// ...
}
Use Bulk Deletion
For scenarios where a large number of detail entities need to be deleted, prefer using bulk deletion queries over individual entity deletion. This optimizes the delete operation by executing a single SQL statement to remove multiple entities, reducing the overall database load and improving performance.
int deletedCount = entityManager.createQuery(
"DELETE FROM DetailEntity d WHERE d.master = :masterEntity"
)
.setParameter("masterEntity", masterEntity)
.executeUpdate();
Scalability Considerations
Load Testing and Performance Tuning
Conduct load testing using tools like Apache JMeter or Gatling to measure the performance of master-detail CRUD operations under varying levels of load. Based on the test results, fine-tune the database indexes, queries, and caching strategies to optimize the application's scalability.
Horizontal Scaling with Database Sharding
Consider implementing horizontal scaling techniques such as database sharding to distribute the master-detail data across multiple database instances. This approach helps in handling increased data volumes and improving overall system scalability.
In Conclusion, Here is What Matters
Optimizing master-detail CRUD operations is essential for building performant, scalable, and maintainable enterprise applications. By employing best practices like lazy loading, caching, batch operations, and scalability considerations, Java developers can ensure efficient handling of master-detail relationships. Furthermore, continuously monitoring and fine-tuning the application's performance is key to achieving optimal results.
Incorporating these optimization strategies into Java-based master-detail CRUD operations can lead to improved application performance, better utilization of resources, and enhanced user experience. By continuously evaluating and refining these practices, developers can ensure that their applications remain responsive and scalable, even as they handle increasing data volumes and user loads.