Troubleshooting Common Errors in Servlet 3.0 Implementation
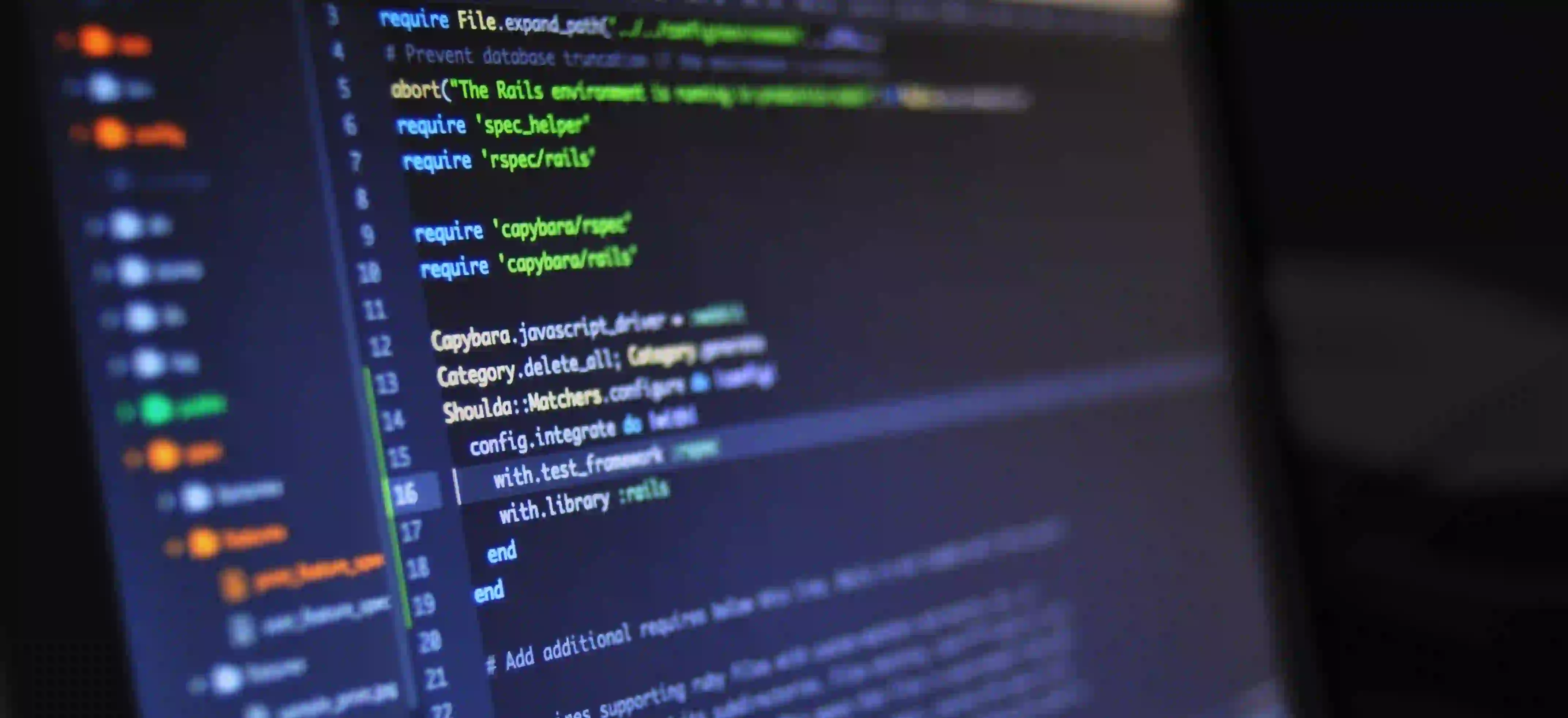
Troubleshooting Common Errors in Servlet 3.0 Implementation
Servlet 3.0 is a crucial component of Java web development, offering an efficient way to handle requests and responses. However, like any technology, it's not immune to errors. In this article, we'll explore some common errors encountered during Servlet 3.0 implementation and discuss how to troubleshoot them effectively.
Error 1: "HTTP Status 404 - Not Found"
The "HTTP Status 404 - Not Found" error is one of the most common issues when working with servlets. It occurs when the servlet container cannot locate the requested resource, typically due to incorrect URL mapping or deployment configuration.
Troubleshooting Steps:
-
Check the URL mapping in web.xml: Ensure that the servlet's URL pattern in the web.xml file matches the URL used to access the servlet. For example:
📄snippet.txt<servlet-mapping> <servlet-name>MyServlet</servlet-name> <url-pattern>/myservlet</url-pattern> </servlet-mapping>
-
Verify the servlet mapping in annotation: If using the annotation-based approach, validate that the
@WebServlet
annotation's URL pattern aligns with the requested URL.☕snippet.java@WebServlet(name = "MyServlet", urlPatterns = "/myservlet") public class MyServlet extends HttpServlet { // Servlet implementation }
-
Check the deployment descriptor: Ensure that the servlet configuration is present and correctly defined in the deployment descriptor (web.xml).
By thoroughly checking these areas, you can often identify and resolve the root cause of the "HTTP Status 404 - Not Found" error.
Error 2: "java.lang.ClassNotFoundException: javax.servlet.ServletContainerInitializer"
The "java.lang.ClassNotFoundException: javax.servlet.ServletContainerInitializer" error is encountered when the servlet container fails to find the specified class during initialization.
Troubleshooting Steps:
-
Verify the Servlet API version: Servlet 3.0 introduced the ServletContainerInitializer class, and it requires a compatible servlet container. Ensure that you are using a servlet container that supports Servlet 3.0 or later, such as Apache Tomcat 7 or later versions.
-
Check the dependencies: If you're using a build tool like Maven, make sure that the servlet API dependency is included in your project's dependencies.
📄snippet.txt<dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> <version>3.0.1</version> </dependency>
By ensuring the correct Servlet API version and including the necessary dependencies, you can resolve the "java.lang.ClassNotFoundException: javax.servlet.ServletContainerInitializer" error.
Error 3: "HTTP Status 405 - Method Not Allowed"
The "HTTP Status 405 - Method Not Allowed" error occurs when the HTTP method used in the request is not supported by the servlet.
Troubleshooting Steps:
-
Check the HTTP method used: Verify that the HTTP method (such as GET, POST, PUT, DELETE) in the request matches the supported methods in the servlet.
☕snippet.javaprotected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // Servlet logic for handling GET requests } protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // Servlet logic for handling POST requests }
-
Ensure proper method implementation: If the servlet supports a particular HTTP method, ensure that the corresponding method (doGet, doPost, etc.) is correctly implemented in the servlet code.
By matching the HTTP method with the supported methods in the servlet and ensuring proper method implementation, you can effectively address the "HTTP Status 405 - Method Not Allowed" error.
Closing Remarks:
In Java web development, understanding and troubleshooting common errors in Servlet 3.0 implementation is essential for building robust and reliable web applications. By following the troubleshooting steps outlined in this article, you can effectively diagnose and resolve issues related to URL mapping, class loading, and unsupported HTTP methods. Furthermore, staying updated with the latest Servlet API documentation and utilizing robust development tools can immensely aid in avoiding and resolving common errors in Servlet 3.0 implementation.
Remember, a thorough understanding of servlet configuration, deployment, and HTTP methods is key to mastering Servlet 3.0 and delivering seamless web experiences to users.
For further insights into Java servlets and web development, consider exploring the official Java Servlet API documentation and Servlet 3.0 tutorial.
Happy coding!