Managing Mutable Objects in Python
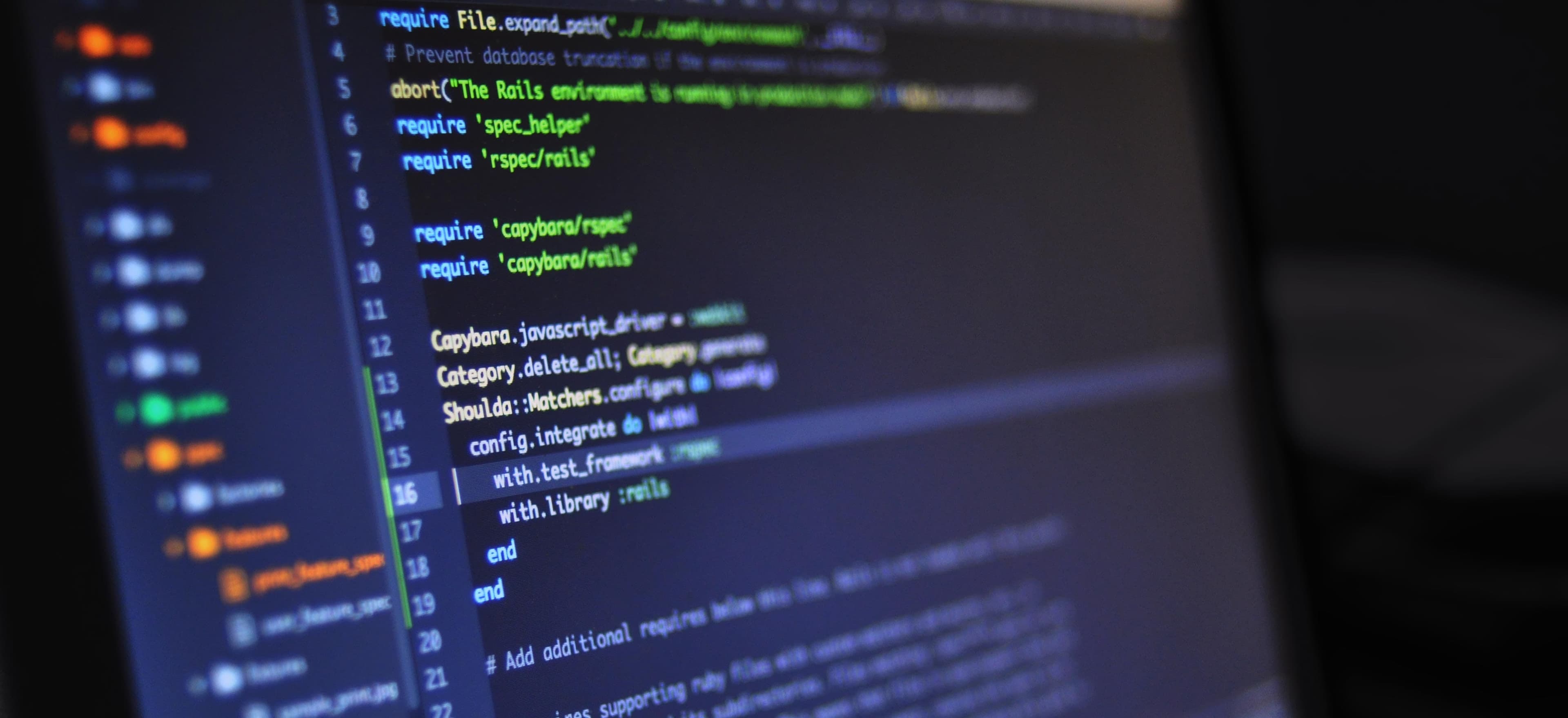
- Published on
Best Practices for Managing Mutable Objects in Python
When working with mutable objects in Python, it's crucial to understand how they behave and how to manage them effectively. Mutable objects, such as lists, dictionaries, and sets, can lead to unexpected behavior if not handled properly. In this post, we'll delve into best practices for managing mutable objects in Python, including common pitfalls and how to avoid them.
What are Mutable Objects?
Mutable objects in Python are those whose state can be changed after they are created. This means that you can modify the object's content, add or remove elements, or update its internal state without creating a new object. Examples of mutable objects in Python include lists, dictionaries, sets, and instances of custom classes with mutable attributes.
Pitfalls of Mutable Objects
Unexpected Side Effects
One of the primary pitfalls of mutable objects is the potential for unexpected side effects. When passing a mutable object as an argument to a function or method, any changes made to the object within the function will persist outside of the function scope. This can lead to unintended consequences and make the code harder to reason about.
Shared References
Mutable objects can also result in shared references, where multiple variables point to the same object in memory. Modifying the object through one reference will affect all other references pointing to the same object, which can lead to bugs that are difficult to trace.
Difficulty in Reasoning about Code
The mutability of objects can make it challenging to reason about the code, especially in a multi-threaded or concurrent environment. Managing shared mutable state across different threads or processes introduces complexities and potential race conditions.
Best Practices for Managing Mutable Objects
Immutability Where Possible
When designing data structures or classes, consider making them immutable where possible. Immutable objects, such as tuples and namedtuples, can help reduce the risk of unintended side effects and make the code easier to reason about.
from collections import namedtuple
Point = namedtuple('Point', ['x', 'y'])
p = Point(x=1, y=2)
In the example above, Point
is an immutable data structure, and once created, its state cannot be modified. This can help prevent accidental changes and facilitate cleaner code.
Avoiding In-Place Modifications
Avoid in-place modifications of mutable objects whenever feasible. Instead of modifying the original object, prefer creating a new object with the desired changes. For example, instead of modifying a list in place, use methods like append
, extend
, or list comprehensions to create a new list with the necessary modifications.
original_list = [1, 2, 3]
modified_list = original_list + [4] # Creates a new list with the added element
Copying Mutable Objects
When passing mutable objects as arguments to functions or methods, consider creating a copy of the object within the function to avoid unintended side effects. The copy
module provides a variety of functions for creating shallow or deep copies of mutable objects, depending on the specific use case.
import copy
original_dict = {'a': 1, 'b': 2}
copied_dict = copy.deepcopy(original_dict) # Creates a deep copy of the original dictionary
Using Immutable Data Types as Keys
When using mutable objects as keys in dictionaries or elements in sets, unexpected behavior can arise due to changes in the object's hash value. To avoid this, use immutable data types as keys to ensure consistent behavior.
mutable_key = [1, 2, 3]
immutable_key = tuple(mutable_key) # Using a tuple as an immutable key
Thread Safety and Concurrency
When dealing with mutable objects in a multi-threaded or concurrent environment, proper synchronization and locking mechanisms are essential to ensure thread safety. Consider using thread-safe data structures from the concurrent.futures
module or implementing locking mechanisms to protect shared mutable state.
Wrapping Up
In conclusion, while mutable objects offer flexibility and efficiency, they also introduce complexities that require careful management. By following best practices such as promoting immutability, avoiding in-place modifications, and using copies effectively, you can mitigate the risks associated with mutable objects and write more robust and predictable code.
Understanding the behavior of mutable objects and how to manage them effectively is a fundamental aspect of Python programming, especially in the context of data manipulation, concurrent programming, and performance optimization.
For further reading on mutable objects and best practices, refer to the official Python documentation on Mutable Sequence Types and Copy Operation.
Remember, with great mutability comes great responsibility!