Avoiding Common Pitfalls in Hibernate
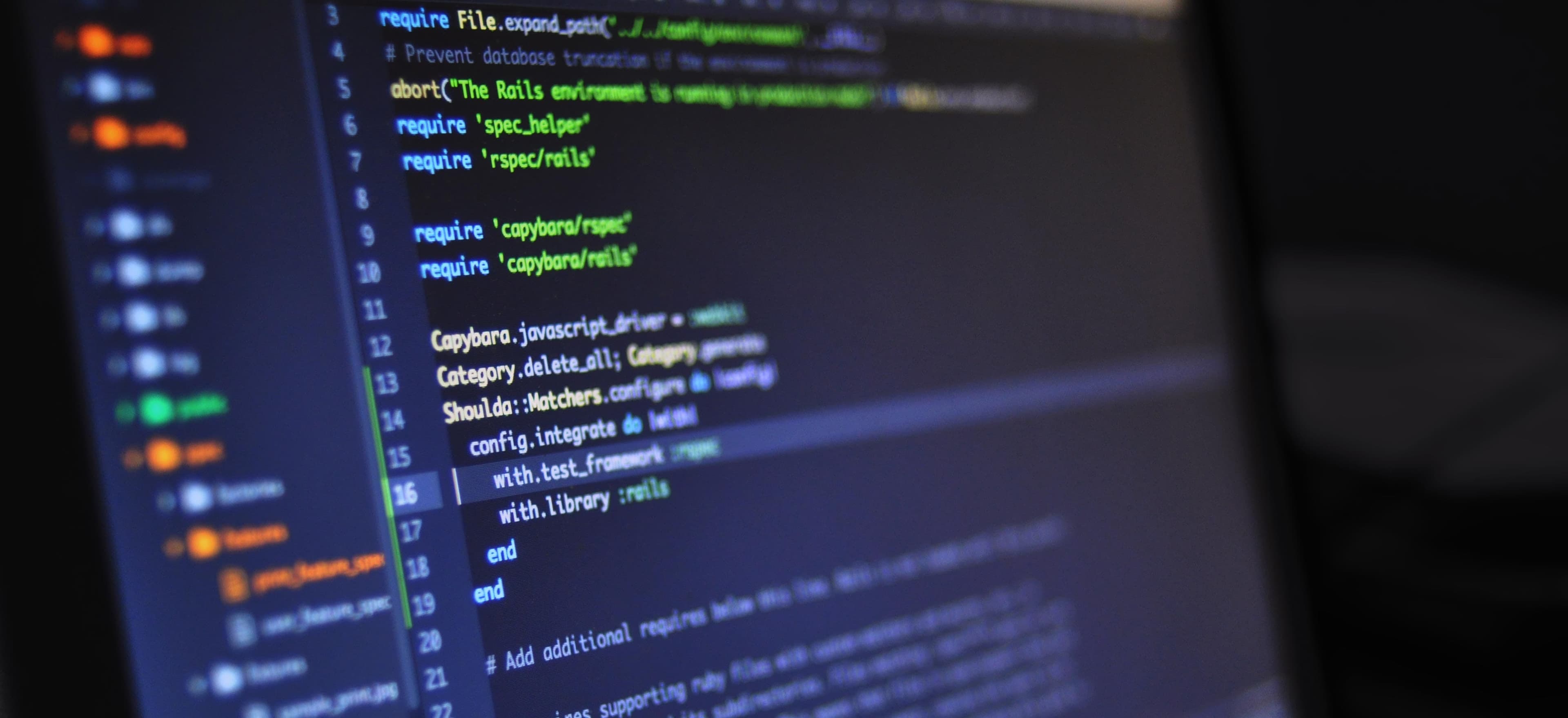
- Published on
Avoiding Common Pitfalls in Hibernate
Hibernate is a powerful and popular object-relational mapping (ORM) tool for Java, providing a framework for mapping an object-oriented domain model to a traditional relational database. While Hibernate offers numerous benefits, it also presents challenges and potential pitfalls. Understanding and addressing these pitfalls is crucial for maximizing the effectiveness of Hibernate in your Java projects.
In this article, we'll explore some common pitfalls in Hibernate and discuss strategies for avoiding them.
1. N+1 Query Problem
The N+1 query problem occurs when Hibernate executes a large number of individual SQL queries to fetch associated entities, leading to performance issues. This often happens when working with relationships between entities.
Example:
@Entity
public class Author {
// ...
@OneToMany(mappedBy = "author", cascade = CascadeType.ALL, fetch = FetchType.LAZY)
private List<Book> books;
// ...
}
List<Author> authors = entityManager.createQuery("SELECT a FROM Author a", Author.class)
.getResultList();
for (Author author : authors) {
for (Book book : author.getBooks()) {
// ...
}
}
In this example, if there are N authors, Hibernate will execute N+1 SQL queries to fetch all the associated books, resulting in a performance overhead.
Solution:
Use JOIN FETCH
in the query to fetch the associated entities eagerly:
List<Author> authors = entityManager.createQuery("SELECT DISTINCT a FROM Author a LEFT JOIN FETCH a.books", Author.class)
.getResultList();
2. Overuse of Eager Fetching
Eager fetching can lead to performance issues by fetching more data than necessary. When using eager fetching, related entities are loaded immediately along with the parent entity, even if they are not required at that point.
Example:
@Entity
public class Author {
// ...
@OneToMany(mappedBy = "author", cascade = CascadeType.ALL, fetch = FetchType.EAGER)
private List<Book> books;
// ...
}
In this example, every time an Author
entity is fetched, all associated Book
entities will also be fetched eagerly, potentially leading to unnecessary data retrieval and increased memory usage.
Solution:
Prefer lazy fetching when possible and use explicit fetching strategies where necessary. Use @ManyToOne
with FetchType.LAZY
for associations to avoid unnecessary data retrieval unless required.
3. Ignoring Transaction Boundaries
Hibernate requires a clear understanding of transaction boundaries. Ignoring transaction boundaries can lead to data consistency issues and potential errors, as Hibernate manages the persistence context within a transaction.
Example:
public void updateAuthor(Long authorId, String newName) {
Author author = entityManager.find(Author.class, authorId);
author.setName(newName);
// Missing transaction management
// entityManager.persist(author);
}
In this example, the update operation on the author
object is not within a transaction boundary, potentially leading to inconsistent data if an exception occurs during the update.
Solution:
Always manage transactions explicitly, either using Spring-managed transactions, Java EE container-managed transactions, or manual transaction demarcation. Annotate methods with @Transactional
or use EntityManager
within a try-catch-finally
block to ensure proper transaction boundaries.
4. Failure to Utilize Caching
Hibernate provides first-level and second-level caching mechanisms to improve performance by reducing the need for repeated database access. Failure to utilize caching can lead to suboptimal performance, especially in applications with high read-to-write ratios.
Example:
Author author1 = entityManager.find(Author.class, 1L); // First database hit
Author author2 = entityManager.find(Author.class, 1L); // Second database hit
In this example, the second find
operation will result in another database hit, even though the data for the Author
with ID 1
is already in the persistence context.
Solution:
Enable and configure appropriate caching mechanisms, such as the second-level cache using providers like Ehcache or Hazelcast. Utilize query caching and entity caching to reduce database access and improve application performance.
Bringing It All Together
Hibernate, while providing powerful ORM capabilities, requires careful handling to avoid common pitfalls that can impact performance and data consistency. By understanding and addressing these pitfalls, developers can leverage Hibernate effectively in their Java projects.
By addressing the N+1 query problem, using fetching strategies judiciously, managing transaction boundaries effectively, and leveraging caching mechanisms, developers can maximize the benefits of Hibernate while minimizing potential issues.
By mastering these aspects of Hibernate, developers can build high-performance, scalable, and efficient Java applications with robust persistence capabilities.
References:
- Hibernate Performance Tuning: https://www.baeldung.com/hibernate-performance-tuning
- Hibernate Caching Strategies: https://www.baeldung.com/hibernate-second-level-cache
Stay tuned for more insights on Java and Hibernate best practices!