Understanding Type Safety Issues in Java Generics
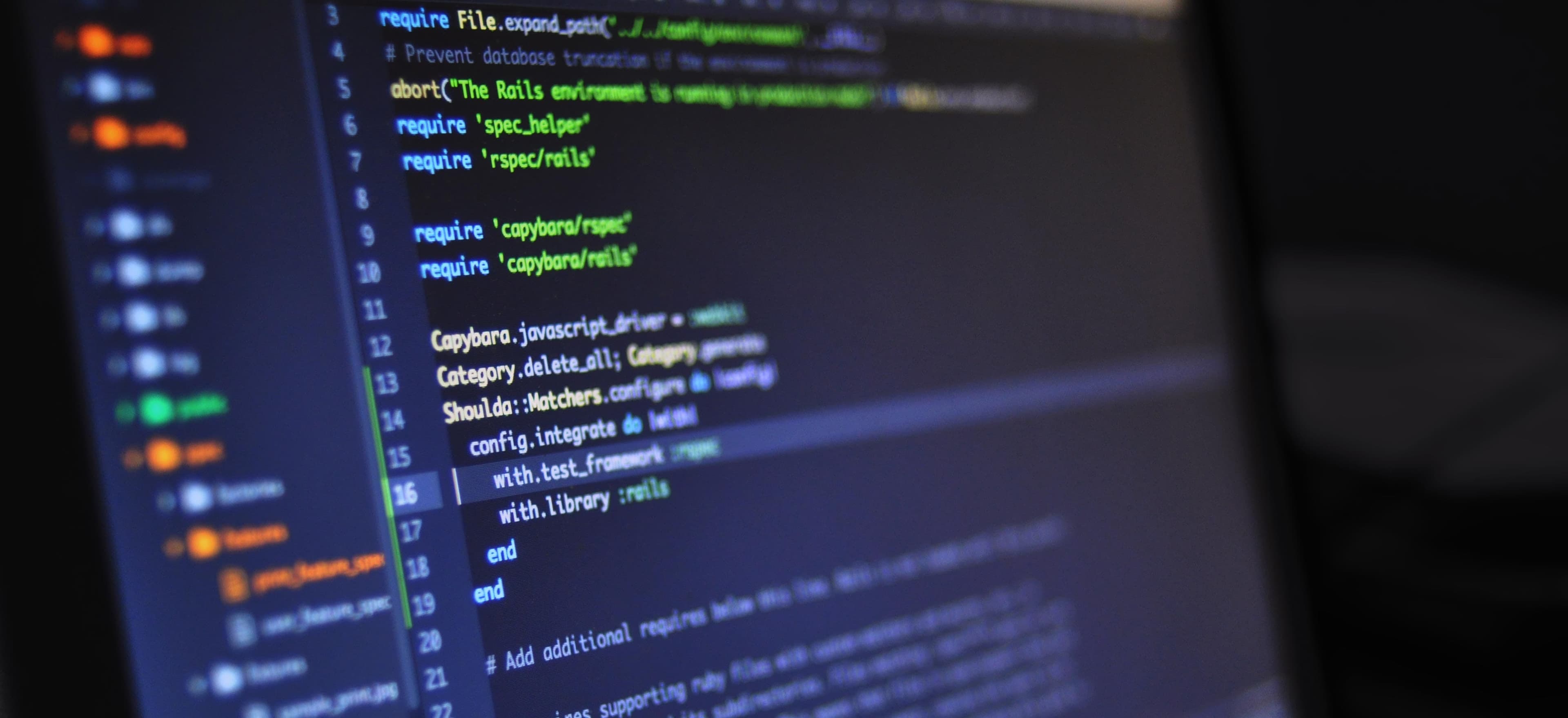
- Published on
Understanding Type Safety Issues in Java Generics
Java Generics, introduced in Java 5, brought a powerful feature to the language, allowing developers to implement generic classes and methods. This feature promotes code reusability and increases type safety. However, it's essential to understand that Generics is not without its complexities. In this blog post, we will delve into the type safety issues associated with Java Generics. We'll discuss what these issues are, how they manifest in Java, and provide strategies to mitigate them.
What Are Java Generics?
Java Generics enable the creation of classes, interfaces, and methods with a placeholder for data types. The primary advantage of Generics is to enable code that can operate on various data types while still providing compile-time type checking.
import java.util.ArrayList;
import java.util.List;
public class GenericListExample<T> {
private List<T> items;
public GenericListExample() {
items = new ArrayList<>();
}
public void addItem(T item) {
items.add(item);
}
public T getItem(int index) {
return items.get(index);
}
}
Why Use Generics?
In the code snippet above, we define a generic class GenericListExample
with a type parameter T
. This allows us to create a list that can store any object type, enhancing the flexibility of our code.
Type safety is implemented here since Java's compiler checks if the correct types are used, reducing the chances of ClassCastException
at runtime.
Type Safety Issues in Java Generics
Despite their many advantages, Java Generics can still introduce type safety issues. Below we outline several important concerns:
1. Raw Types
Using raw types directly bypasses the generic type checks provided by Java. For instance, if a generic class is used without specifying a type parameter, it defaults to a raw type.
GenericListExample list = new GenericListExample(); // Raw type
list.addItem("Hello");
list.addItem(123); // This is allowed, but can cause issues
Why Is This a Problem?
By using a raw type, the compiler does not perform any type checks. This could lead to situations where a runtime casting exception occurs. This example demonstrates that a list designed to hold any object can lead to fragile code that becomes more difficult to maintain.
2. Type Erasure
Java implements generics through a process called type erasure. The generic type information is removed during compilation. For example, a List<String>
simply becomes a List
in the compiled bytecode. This means that:
- The type parameter information is lost, triggering limitations in type checks at runtime.
- You cannot create an instance of a generic type directly, like
new T()
, since the actual type is not known.
Example:
public class Pair<K, V> {
private K key;
private V value;
public Pair(K key, V value) {
this.key = key;
this.value = value;
}
}
One significant impact of type erasure is that we cannot retrieve the generic type at runtime, making it tough to enforce strict type constraints.
3. Inheritance and Covariance
Generics can pose challenges when dealing with inheritance: specifically, with covariance and contravariance.
List<Object> objects = new ArrayList<String>(); // Compilation error
Why Does This Occur?
Although String
is a subtype of Object
, List<String>
is not considered a subtype of List<Object>
. This distinction is crucial for maintaining type safety because it prevents potential runtime exceptions from treating incompatible types as compatible.
4. Wildcards and Their Limits
Wildcards in Generics (? extends T
and ? super T
) provide flexibility but can also cause confusion.
public void printList(List<? extends Number> list) {
for (Number number : list) {
System.out.println(number);
}
}
Why Be Cautious?
While you can read items from this list, you can't add items to it without getting a compile-time error. This behavior can frustrate developers new to Java Generics. Learning how to apply wildcards effectively is important for avoiding potential pitfalls.
Best Practices for Maintaining Type Safety in Java Generics
Now that we understand some of the type safety issues associated with Java Generics, let's explore best practices for efficient and safe usage.
1. Always Specify Type Parameters
When defining or using generics, always specify the type parameters. This ensures the compiler can carry out type checks and provides clarity to anyone reading your code:
GenericListExample<String> stringList = new GenericListExample<>();
2. Avoid Raw Types
Using raw types should be avoided entirely. If you encounter legacy code that uses generics improperly, consider refactoring it to embrace the type safety that Generics offer.
3. Use Bounded Type Parameters
Whenever possible, use bounded type parameters to restrict the types that can be used. This can ensure that method parameters and return types are only processed if they meet certain criteria:
public <T extends Number> void process(T number) {
// process number
}
4. Familiarize Yourself with Wildcards
Understanding the difference between ? extends
and ? super
will aid in managing collections effectively. Identifying where to use these wildcards will not only maintain type safety but also enable cleaner code.
The Last Word
Java Generics enhance code safety and efficiency by allowing developers to write flexible yet type-safe code. However, as we've discussed, they do introduce type safety concerns, particularly through the concepts of raw types, type erasure, inheritance, and wildcards.
By adhering to best practices, including always specifying type parameters, avoiding raw types, utilizing bounded type parameters, and gaining an understanding of wildcards, developers can harness the full power of Java Generics while minimizing type-related issues.
For further reading, check out the official Java documentation on Generics and explore related topics in the Java Language Specification for a deeper understanding of the foundations of Generics.
Embrace the complexities of Java Generics, and you will find yourself writing more elegant, reusable, and robust Java code. Happy coding!
Checkout our other articles