Resolving Java Classloading Deadlocks in Complex Applications
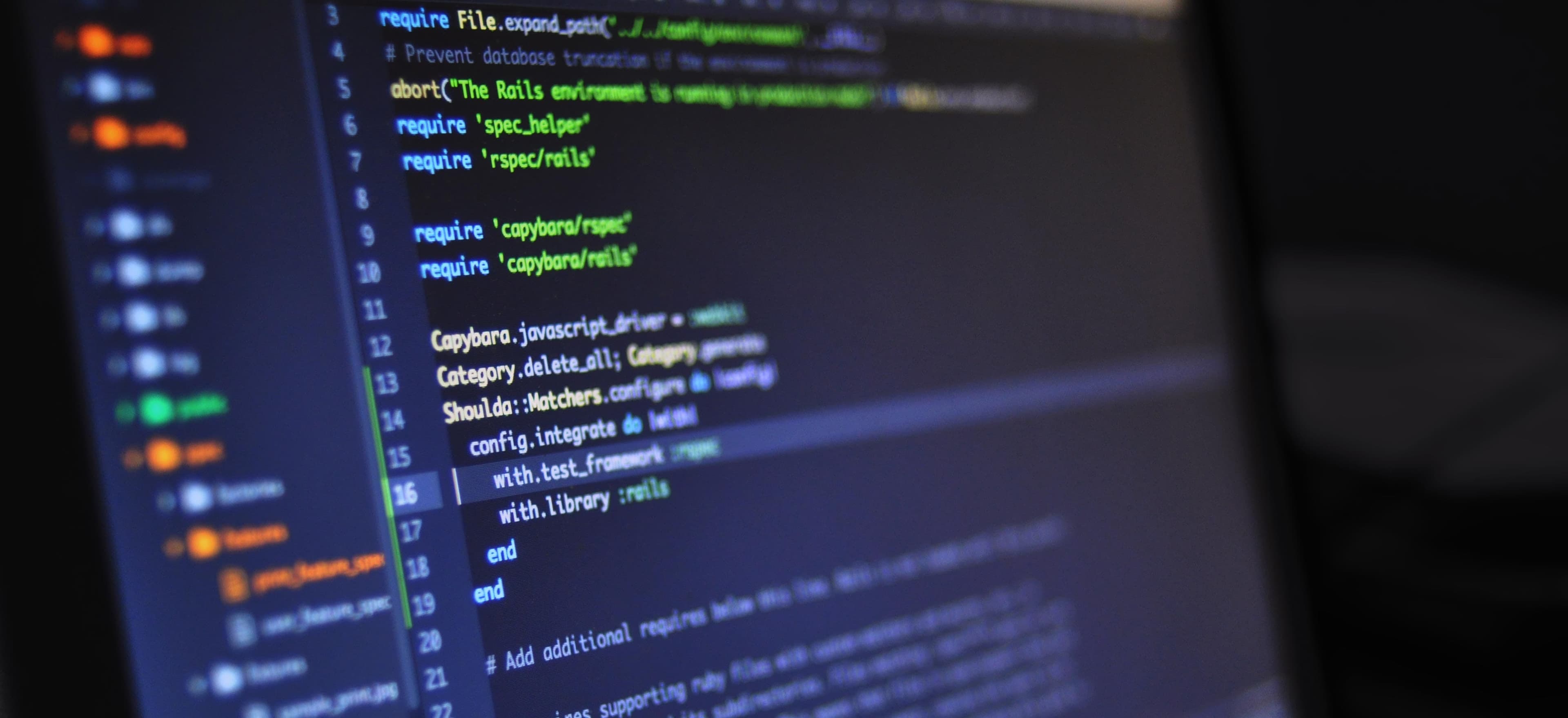
- Published on
Resolving Java Classloading Deadlocks in Complex Applications
Java is renowned for its versatility and portability, but even with these advantages, Java applications can run into complexities that lead to existential issues such as classloading deadlocks. This article delves into the mechanics behind these deadlocks and provides valuable strategies for resolving them.
Understanding Classloading
In Java, classloading refers to the process of loading class files from their binary format into memory. The Java Virtual Machine (JVM) employs a classloader hierarchy to facilitate this. Each classloader plays a critical role in loading classes and resources. When dealing with large-scale applications, particularly ones with multiple modules, these interactions can become convoluted.
Types of Classloaders
Here are the main types of classloaders in Java:
- Bootstrap ClassLoader: The parent of all classloaders, it loads fundamental Java classes from the Java Runtime Environment (JRE).
- Extension ClassLoader: Loads classes from the extension directories of the JRE.
- Application ClassLoader: Loads classes from the application's classpath.
Understanding how these classloaders interact is crucial for managing classloading dependencies effectively.
What is a Classloading Deadlock?
A classloading deadlock occurs when two or more classes are waiting for each other to load, leading the application to hang indefinitely. This typically happens when:
- Class A references Class B during its loading process.
- Class B references Class A, creating a circular dependency.
Example of a Classloading Deadlock
Consider the following classes:
public class A {
static {
// This will cause Class B to be loaded.
new B();
}
}
public class B {
static {
// This will cause Class A to be loaded.
new A();
}
}
When you attempt to load either Class A or Class B, you will encounter a deadlock. Class A's static block tries to load Class B, while Class B's static block simultaneously attempts to load Class A.
Key Takeaway: Circular dependencies among classes can lead to a deadlock and halt your application.
Identifying Classloading Deadlocks
Identifying deadlocks can be challenging. A few strategies include:
- Verbose Class Loading: Running the JVM with the
-verbose:class
flag can provide insight into which classes are being loaded and when. - Java Flight Recorder: Using the Java Flight Recorder and JVM tools can help illuminate the state of the application during runtime.
- Thread Dumps: Analyze thread dumps to identify threads engaged in classloading operations waiting on locks.
Resolving Classloading Deadlocks
Once you understand the causes and symptoms of classloading deadlocks, you can take steps to resolve them. Below are several strategies.
1. Refactor Code to Eliminate Circular Dependencies
Refactoring is often the most straightforward solution. Try to avoid circular dependencies by decomposing your classes or rethinking your design. For instance, consider using interfaces.
Example Refactored Code
public interface A {
void doSomething();
}
public class AImpl implements A {
private final B b;
public AImpl(B b) {
this.b = b;
}
public void doSomething() {
b.someMethod();
}
}
public class B {
private final A a;
public B(A a) {
this.a = a;
}
void someMethod() {
// Implementation
}
}
In this design, you eliminate direct circular dependencies. A and B rely on each other through abstraction via an interface.
2. Lazy Initialization
Instead of loading classes immediately, defer the loading of classes until they are needed. This allows you to minimize the chances of deadlocks occurring.
Example of Lazy Initialization
public class LazyLoader {
private A a;
public A getA() {
if (a == null) {
a = new A(); // A is only created here when needed
}
return a;
}
}
3. Use Dependency Injection
Utilize a dependency injection framework like Spring to manage the lifecycle and dependencies of your classes. Dependency injection containers can handle circular references more gracefully than manual instantiation.
Example Using Spring
@Configuration
public class AppConfig {
@Bean
public A a(B b) {
return new A(b);
}
@Bean
public B b(A a) {
return new B(a);
}
}
In this example, Spring manages the instantiation and resolves dependencies, significantly reducing the risk of deadlocks.
4. Custom Classloaders
In extreme cases, you may consider implementing custom classloaders. This is advanced territory and typically should be a last resort, as it can complicate your application’s architecture.
Simplified Custom ClassLoader Example
public class MyClassLoader extends ClassLoader {
@Override
protected Class<?> findClass(String name) throws ClassNotFoundException {
// Custom loading logic here
return super.findClass(name);
}
}
Caution: Custom classloaders can lead to performance issues if not implemented correctly.
5. Code Review and Best Practices
Encourage thorough code reviews focused on classloading behavior, especially during major refactors. Following best practices can help you avoid potential deadlocks.
- Modularize Your Code: Smaller, well-defined modules can reduce complexity.
- Keep Static Initializers Simple: Avoid heavy logic inside static blocks.
- Minimize Class Interdependencies: Use design patterns, such as the observer or strategy pattern, to make your classes more decoupled.
Bringing It All Together
Classloading deadlocks may be a challenging aspect of Java programming, but they can be managed through careful design and coding practices. By identifying their causes and understanding the nuances of classloading, you can steer your application clear of these deadlocks. Implementing strategies such as refactoring, lazy initialization, and dependency injection can significantly mitigate risks.
For more about Java development, consider exploring additional resources on Oracle's Class Loading Mechanism and Dependency Injection in Spring to further enhance your knowledge.
Checkout our other articles