ReentrantLock vs Synchronized: Choosing the Right Tool
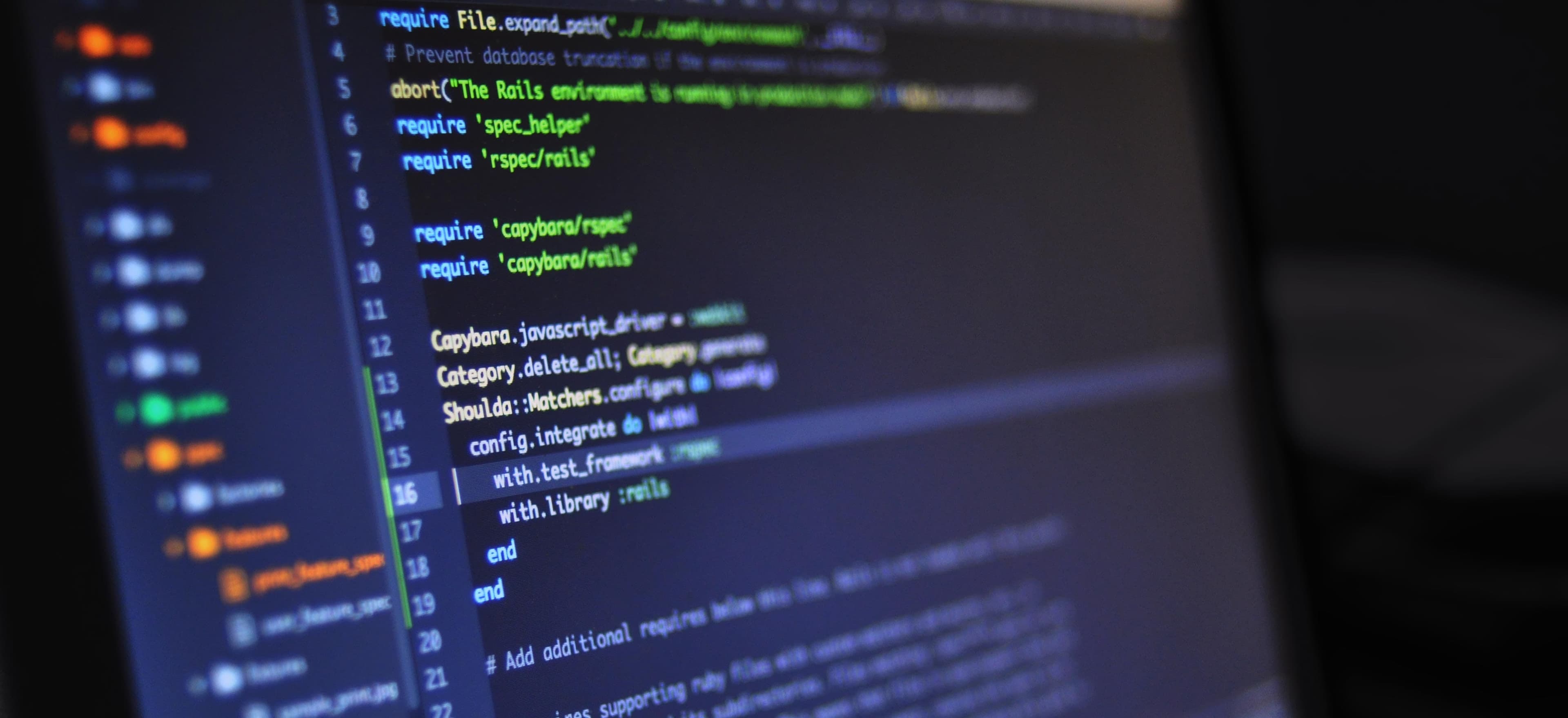
- Published on
ReentrantLock vs Synchronized: Choosing the Right Tool in Java
Concurrency is a fundamental aspect of modern programming that allows for multiple threads to execute simultaneously. In Java, two primary mechanisms facilitate thread synchronization: the synchronized
keyword and the ReentrantLock
class. Understanding the differences between these two tools can greatly enhance the performance and maintainability of your multithreaded applications. This article is a comprehensive guide that will help you choose the right tool for your synchronization needs.
Table of Contents
- Understanding Synchronization
- Synchronized: The Basic Locking Mechanism
- ReentrantLock: An Advanced Synchronization Tool
- Key Differences Between Synchronized and ReentrantLock
- When to Use Which Tool
- Conclusion
Understanding Synchronization
Synchronization refers to the coordination between threads to ensure that shared resources are accessed safely. Without synchronization mechanisms, multithreading can lead to inconsistent data, race conditions, and even application crashes.
Java provides built-in support for thread synchronization that ensures only one thread can access a block of code or an object at a time. The two most commonly used synchronization mechanisms in Java are synchronized
and ReentrantLock
.
Synchronized: The Basic Locking Mechanism
The synchronized
keyword in Java is the simplest way to control access to a block of code or an object. When you declare a method or a block as synchronized, a thread obtains a lock for the object on which it is synchronized.
Example of Synchronized Method
public class Counter {
private int count = 0;
public synchronized void increment() {
count++;
}
public synchronized int getCount() {
return count;
}
}
Why Use Synchronized?
In the example above, the increment
and getCount
methods are synchronized, ensuring that only one thread can execute either method at any given time. This simplicity makes synchronized
a convenient choice for basic locking needs.
However, one of the significant drawbacks of synchronized
is its lack of flexibility. Once a thread holds the lock, it cannot be interrupted, and it results in poor performance under contention scenarios.
ReentrantLock: An Advanced Synchronization Tool
ReentrantLock
is part of the java.util.concurrent.locks
package and provides a more sophisticated way to manage synchronization. Unlike synchronized
, ReentrantLock
offers a number of advanced features, including:
- Fairness: You can create a fair lock where the longest-waiting thread gets the access.
- Interruptible Lock Acquisition: Threads can attempt to acquire the lock without blocking indefinitely.
- Try Lock: You can attempt to acquire the lock without blocking by using a timeout.
Example of ReentrantLock
import java.util.concurrent.locks.ReentrantLock;
public class Counter {
private int count = 0;
private final ReentrantLock lock = new ReentrantLock();
public void increment() {
lock.lock(); // Acquire the lock
try {
count++;
} finally {
lock.unlock(); // Always unlock in finally block
}
}
public int getCount() {
lock.lock();
try {
return count;
} finally {
lock.unlock();
}
}
}
Why Use ReentrantLock?
In this example, the increment
and getCount
methods utilize ReentrantLock
. The use of try
...finally
ensures that the lock is always released, preventing deadlocks and resource leaks. Additionally, if you want to implement fair locking:
private final ReentrantLock lock = new ReentrantLock(true); // Fair lock
By passing true
to the constructor, threads are granted lock access in the order they requested it, promoting fairness.
Key Differences Between Synchronized and ReentrantLock
While both synchronized
and ReentrantLock
serve the purpose of controlling access to shared resources, they come with their own sets of advantages and disadvantages:
Ease of Use
- Synchronized: Easier to implement, as it can be used as a simple keyword.
- ReentrantLock: Requires additional boilerplate to acquire and release locks.
Flexibility
- Synchronized: Limited functionality; it cannot be interrupted and does not support try-lock behavior.
- ReentrantLock: Offers advanced features such as fairness, try-lock, and interruptible locking.
Performance
- Synchronized: Has a lower overhead in simple use cases due to JVM optimizations.
- ReentrantLock: May be faster in scenarios with high contention, but usually, the performance gain is negligible when compared to
synchronized
for simpler locking.
Deadlock Prevention
Both mechanisms avoid deadlocks if used properly; however, ReentrantLock
offers more flexibility with lock management.
When to Use Which Tool
Use Synchronized When:
- You require a simple and easy-to-understand locking mechanism.
- You don’t need advanced features such as fairness or interruptible locks.
- You are working in a scenario where performance is not a critical factor.
Use ReentrantLock When:
- You require advanced locking mechanisms like fairness.
- You need to acquire a lock in a way that can be interrupted.
- You want to implement a try-lock mechanism to avoid blocking indefinitely.
- You need to perform more complex thread signaling using Condition variables.
For more comprehensive guidance on multithreading in Java, consider checking Java Concurrency in Practice by Brian Goetz and others.
Closing Remarks
In the choice between synchronized
and ReentrantLock
, your requirements will dictate the appropriate tool. For straightforward synchronization needs, synchronized
often suffices. If your application requires more control and flexibility, then ReentrantLock
may be the better option.
Ultimately, understanding how these two mechanisms interact with Java's memory model and their impact on performance is key to effective multithreading. By choosing the right synchronization tool, you significantly improve your application's reliability and efficiency.
Whichever path you choose, implementing thread-safe code is a critical skill for Java developers, and knowing when to apply each technique will serve you well in your programming endeavors.
Checkout our other articles