Mastering Thread.sleep: Common Pitfalls to Avoid in Java
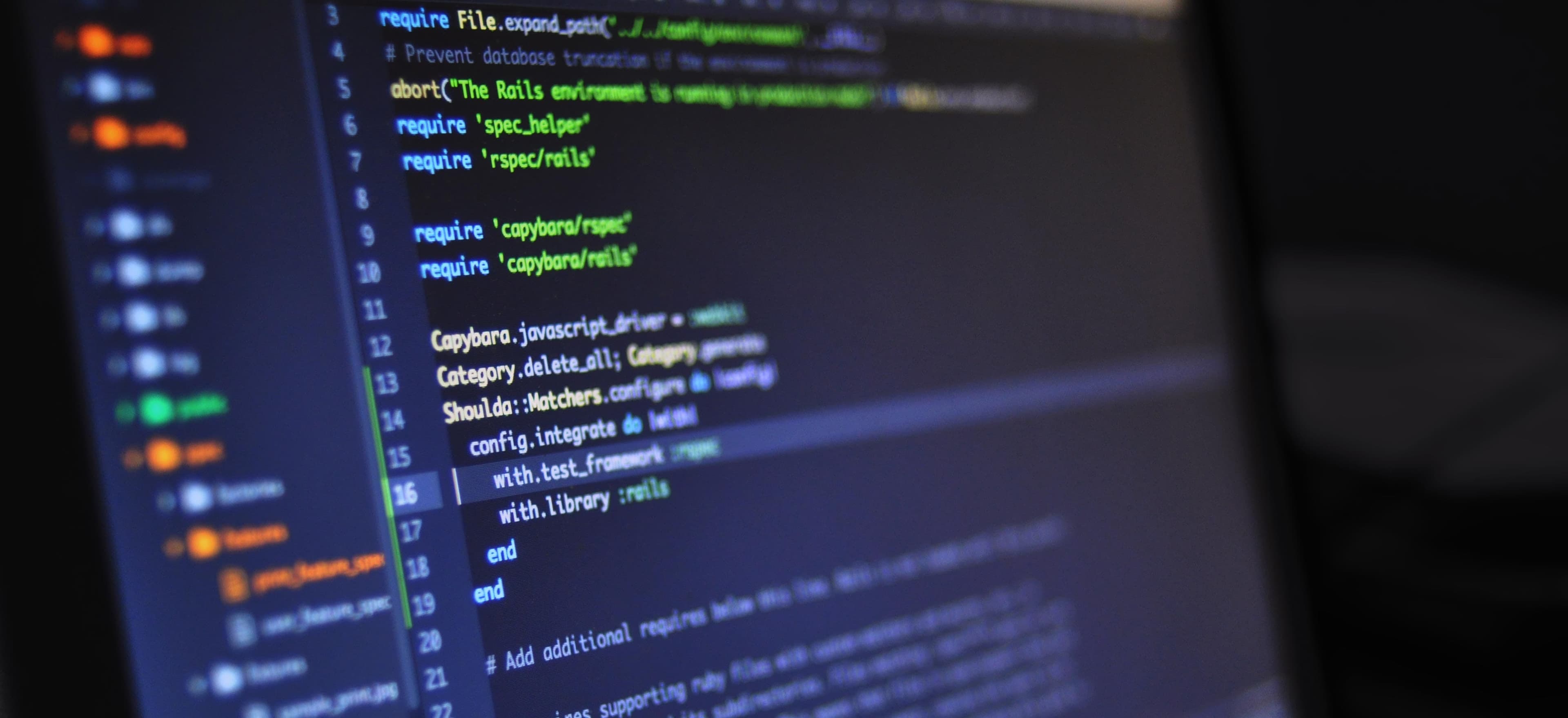
- Published on
Mastering Thread.sleep: Common Pitfalls to Avoid in Java
Java is known for its robust multithreading capabilities. However, with great power comes great responsibility. One common method that developers often use when dealing with multithreading is Thread.sleep()
. While it may seem straightforward, its misuse can lead to a range of pitfalls that can compromise application performance, reliability, and scalability. In this blog post, we'll dive into the common pitfalls associated with Thread.sleep()
and how to avoid them, while also providing practical coding examples.
What is Thread.sleep()?
The Thread.sleep(long millis)
method pauses the execution of the current thread for a specified duration (in milliseconds). This is often used to simulate delays, throttle requests, or even allow other threads to execute. However, depending on how you use it, it can lead to unwanted consequences.
Basic Syntax of Thread.sleep()
Here's a basic example of how to use Thread.sleep()
:
public class SleepExample {
public static void main(String[] args) {
System.out.println("Sleeping for 2 seconds...");
try {
Thread.sleep(2000); // Sleeps for 2000 milliseconds (2 seconds)
} catch (InterruptedException e) {
System.err.println("Sleep was interrupted!");
}
System.out.println("Awake now!");
}
}
Key Takeaway
Always wrap Thread.sleep()
in a try-catch block to handle InterruptedException
, as the sleep can be interrupted by another thread.
Pitfall #1: Overuse of Thread.sleep()
One of the most common mistakes developers make is relying too heavily on Thread.sleep()
to control the flow of the program.
Why is this a problem?
- Inefficiency: Putting a thread to sleep wastes CPU cycles since no useful work can be performed during this time.
- Unpredictable Behavior: Other threads may get blocked, leading to unpredictable delays and possibly causing performance bottlenecks in your application.
Example
Instead of using Thread.sleep()
to wait for a resource:
public class ResourceWait {
private static boolean resourceAvailable = false;
public static void main(String[] args) {
new Thread(() -> {
while (!resourceAvailable) {
try {
Thread.sleep(500); // Poll every 500 milliseconds
} catch (InterruptedException e) {
System.err.println("Interrupted!");
}
}
System.out.println("Resource is available now!");
}).start();
// Simulate resource availability after 3 seconds
try {
Thread.sleep(3000);
resourceAvailable = true;
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
Avoiding Overuse
Consider using alternative approaches such as wait/notify or java.util.concurrent constructs like CountDownLatch
or Semaphore
for more efficient thread cooperation.
Pitfall #2: Not Handling InterruptedException Properly
As noted earlier, Thread.sleep()
can throw an InterruptedException
, which you must handle. However, developers sometimes fail to provide meaningful recovery from this problem.
Why is this a problem?
- Lost Signals: When a thread is interrupted, it's generally because it should cease its operation. Ignoring this can lead to unexpected states in your application.
Example
Consider this code:
public class InterruptedSleep {
public static void main(String[] args) {
Thread task = new Thread(() -> {
try {
System.out.println("Starting task, sleeping for 5 seconds...");
Thread.sleep(5000);
} catch (InterruptedException e) {
System.out.println("Sleep interrupted!");
}
System.out.println("Task completed.");
});
task.start();
task.interrupt(); // Interrupt the sleep
}
}
Best Practice
Always check the interrupted status and respond appropriately. Instead of just printing an error message, you might want to terminate the thread gracefully or retry the operation if it's safe to do so.
if (Thread.currentThread().isInterrupted()) {
// Clean up or stop operation responsibly
}
Pitfall #3: Assuming Thread.sleep() is Accurate
Many developers mistakenly believe that Thread.sleep()
guarantees an exact delay, which is not true. The thread may not wake up precisely after the specified time due to various factors like system load or other high-priority tasks.
Why is this a problem?
- Timing Issues: Applications that rely on timing (like games or animations) may experience stutter or lag if sleep times are not handled properly.
Example
public class AccurateSleep {
public static void main(String[] args) {
long startTime = System.currentTimeMillis();
try {
Thread.sleep(500); // Sleep for 500 milliseconds
} catch (InterruptedException e) {
System.err.println("Interrupted!");
}
long endTime = System.currentTimeMillis();
System.out.println("Slept for: " + (endTime - startTime) + "ms");
}
}
Best Practice
To achieve more accurate timing, consider using a ScheduledExecutorService
or similar constructs that provide scheduling capabilities.
ScheduledExecutorService scheduler = Executors.newScheduledThreadPool(1);
scheduler.schedule(() -> {
System.out.println("Executed after a delay!");
}, 500, TimeUnit.MILLISECONDS);
Pitfall #4: Using Thread.sleep() in GUI Applications
A common mistake in GUI applications is using Thread.sleep()
on the Event Dispatch Thread (EDT). This blocks the UI, making it unresponsive.
Why is this a problem?
- Poor User Experience: The application freezes, and users cannot interact with any component until the sleep ends.
Example
public class GuiExample {
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
JButton button = new JButton("Click Me");
button.addActionListener(e -> {
try {
Thread.sleep(3000); // Freezes the UI
} catch (InterruptedException ex) {
ex.printStackTrace();
}
System.out.println("Button clicked!");
});
JFrame frame = new JFrame("GUI Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.getContentPane().add(button);
frame.setSize(200, 200);
frame.setVisible(true);
});
}
}
Best Practice
Use SwingWorker
or a separate thread for background tasks that require sleep.
SwingWorker<Void, Void> worker = new SwingWorker<>() {
@Override
protected Void doInBackground() throws Exception {
Thread.sleep(3000);
return null;
}
@Override
protected void done() {
System.out.println("Task finished!");
}
};
worker.execute();
The Bottom Line
Mastering Thread.sleep()
is not just about knowing how to use it; it involves understanding its implications and appropriate use cases. We've discussed several pitfalls to avoid, such as overuse, neglecting InterruptedException
, relying on sleep accuracy, and blocking the UI thread in GUI applications.
Always aim for efficient alternatives that promote better thread coordination and user experience. If you follow these guidelines, you will harness the power of Java's multithreading capabilities without falling into common traps.
For more in-depth resources, check out the Java documentation on Concurrency or consult the book Java Concurrency in Practice by Brian Goetz.
By understanding the nuances and being mindful of the common pitfalls, programmers can create robust, responsive, and efficient Java applications. Happy coding!
Checkout our other articles