Addressing Common Pitfalls in Java Native Memory Tracking
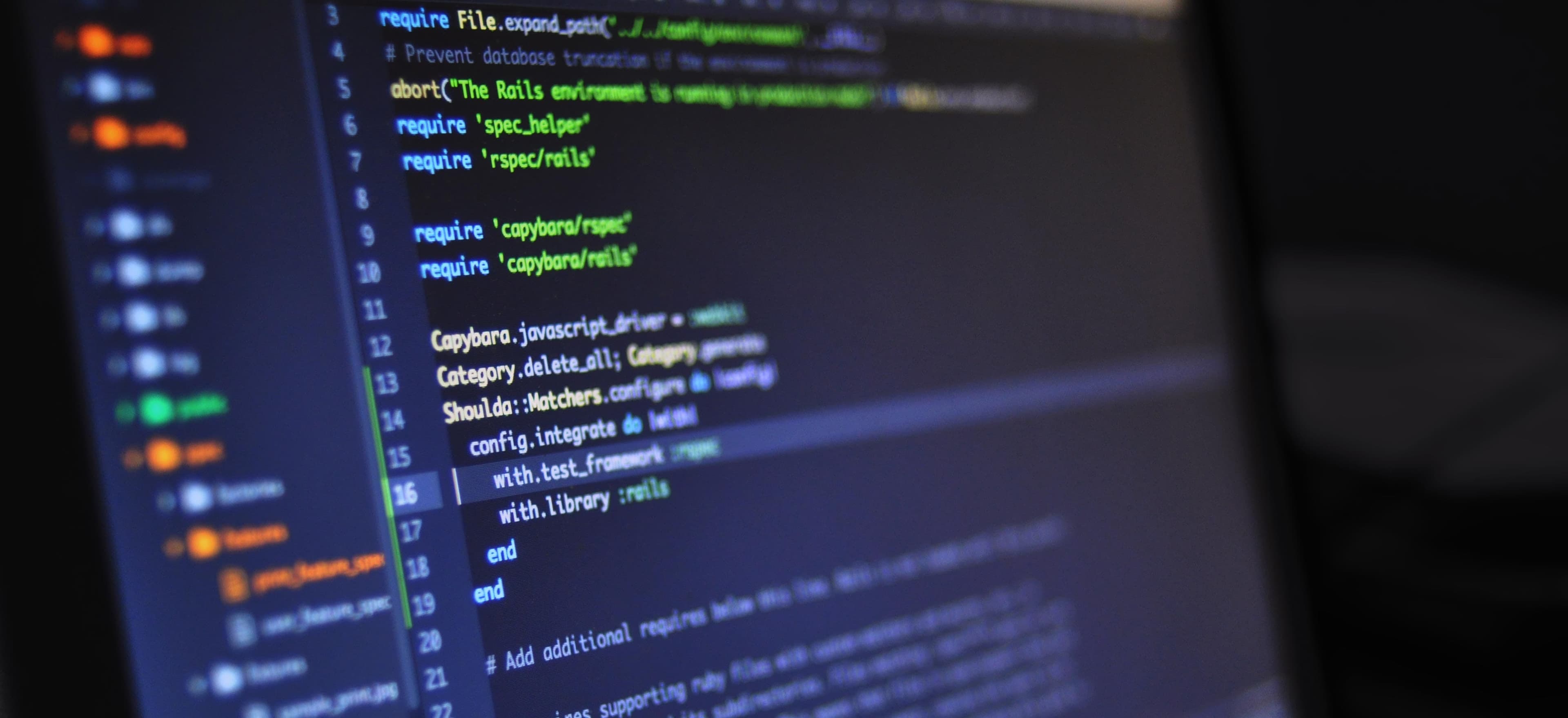
- Published on
Addressing Common Pitfalls in Java Native Memory Tracking
Java, known for its rich ecosystem and comprehensive memory management capabilities, offers developers the ability to interact with native libraries through Java Native Interface (JNI). However, with this power comes the responsibility to handle memory efficiently. This is where Java Native Memory Tracking (NMT) becomes crucial. NMT is a mechanism that helps developers track memory usage in native code. Despite its benefits, many developers encounter pitfalls when using NMT. In this article, we will explore these common pitfalls, how to avoid them, and illustrate effective usage with code examples.
What is Java Native Memory Tracking?
Java Native Memory Tracking is a feature introduced in JDK 8 that allows you to see the memory consumption of native code linked with your Java application. By enabling NMT, you can collect detailed reports on how much native memory is allocated, used, and freed by your application and the libraries it depends on.
For more technical details about NMT, you can refer to the official Java JDK Documentation.
Why is Native Memory Tracking Important?
Understanding native memory usage is essential for diagnosing performance issues and potential memory leaks caused by native code. Java applications that depend heavily on JNI or native libraries can face crashes or stability issues due to poor memory management. Hence, tracking memory usage with NMT is a proactive way to ensure reliable application performance.
Common Pitfalls in Native Memory Tracking
-
Not Enabling Native Memory Tracking
One of the most common oversights developers make is forgetting to enable NMT. NMT is not on by default and needs to be explicitly activated when starting your JVM.
How to Enable NMT: To enable NMT, you can add the following JVM argument when running your Java application:
java -XX:NativeMemoryTracking=summary -jar YourApp.jar
The
summary
option provides a high-level overview of memory usage. If you require detailed tracking, consider usingall
. However, be aware that this could affect performance. -
Failing to Analyze Reports Correctly
NMT provides a lot of data, but without proper analysis, it can be overwhelming. Developers often look at the raw numbers without understanding what they mean.
Tip: Focus on the key sections of the NMT report generated:
Native memory allocation (bytes): total: 123456 bytes committed: 78900 bytes
Understanding these values helps pinpoint where your application may have leaks or excessive memory usage. It is essential to compare allocations over time to look for trends.
-
Ignoring Native Memory Usage During Development
Another common pitfall is neglecting native memory usage during the development phase. Many developers focus solely on managing the heap memory in Garbage Collector (GC) but fail to keep track of native allocations.
Advice: Integrate NMT checks into your continuous integration (CI) pipeline. Running NMT reports as part of your build process can help catch memory issues early.
-
Overlooking Unused Native Libraries
When linking against libraries, it is essential to review if they are being used effectively. Unused libraries can consume native memory, leading to unnecessary overhead.
To analyze this, you can use the NMT report to see where different allocations relate to specific libraries. If a library is not needed, remove it from your project.
-
Failure to Account for Platform Differences
Native memory handling can vary significantly across different systems. For example, Windows and Linux may manage pointers and memory allocation differently.
Caution: Test your application on multiple platforms. Always analyze your NMT data in the context of the operating system your application is running on.
Example: Understanding Native Memory Usage with NMT
Let's consider a simple example where we have a Java application that uses JNI to call a native method. Below, we will demonstrate how to check native memory usage effectively.
Java Class with JNI
public class NativeMemoryExample {
static {
System.loadLibrary("nativeLib");
}
public native void nativeMethod();
public static void main(String[] args) {
NativeMemoryExample example = new NativeMemoryExample();
for (int i = 0; i < 1000; i++) {
example.nativeMethod();
}
System.out.println("Native methods called.");
}
}
C Code for Native Library
Below is a simple C example for our native method, which allocates memory.
#include <jni.h>
#include <stdlib.h>
JNIEXPORT void JNICALL Java_NativeMemoryExample_nativeMethod(JNIEnv *env, jobject obj) {
char *data = (char *)malloc(1024); // Allocate 1 KB
if (data == NULL) {
// Handle memory allocation failure
return;
}
// Use the allocated memory...
free(data); // Don't forget to free the memory
}
Analyzing Native Memory Usage
After compiling both the Java and C portions, run your application with NMT enabled. Figure out how much native memory is consumed with the following command:
java -XX:NativeMemoryTracking=summary -jar NativeMemoryExample.jar
You should observe something similar to:
Native memory allocation (bytes):
total: 1048576 bytes
committed: 1024000 bytes
Here, you can compare the values before and after executing your application logic to assess the memory allocation.
Closing Remarks
Navigating the complexities of Java Native Memory Tracking can significantly enhance the stability and performance of your Java applications that utilize native code. By avoiding common pitfalls such as not enabling NMT, failing to analyze reports accurately, and overlooking unused libraries, you can radically improve your code's efficiency.
Remember, NMT is not just a debugging tool; it's a crucial part of the development process when working with JNI. Incorporating it into your workflow will lead to better memory management and ultimately a more reliable application.
For further reading on Java memory management strategies, check out Effective Java by Joshua Bloch and Java Performance: The Definitive Guide.
If you have suggestions or questions regarding Native Memory Tracking, feel free to share your thoughts in the comments below!
Checkout our other articles