Mastering Java NIO: Overcoming Common Blocking Pitfalls
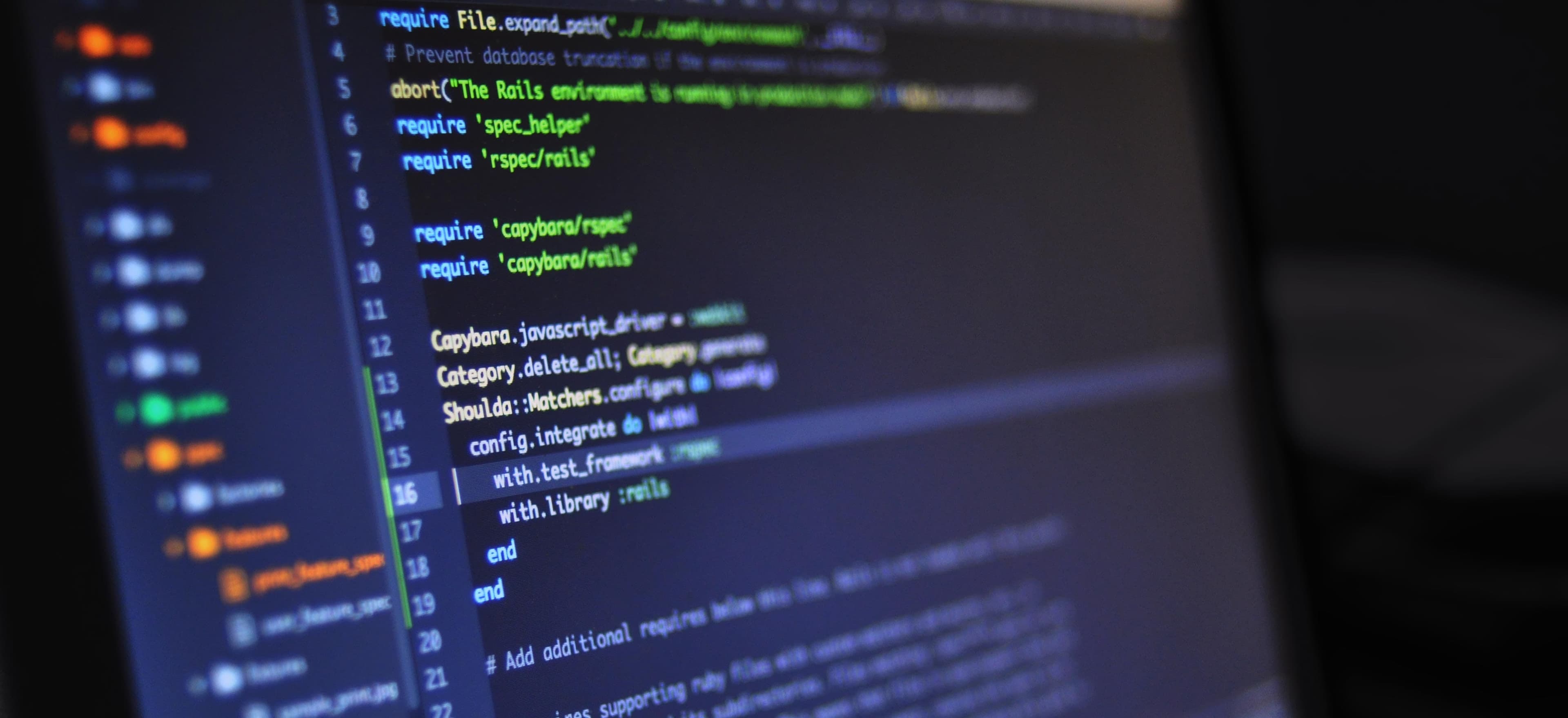
- Published on
Mastering Java NIO: Overcoming Common Blocking Pitfalls
Java's New Input/Output (NIO) package is a powerful tool for handling I/O operations with a much more scalable and efficient approach. Compared to the traditional I/O, NIO enhances performance, especially when dealing with multiple input/output channels. However, new users may encounter common pitfalls, especially related to blocking operations. This blog post aims to explore these pitfalls and provide effective strategies to overcome them, while also demonstrating practical examples along the way.
Understanding Java NIO
Java NIO, introduced in Java 1.4, brings several key features:
- Non-Blocking I/O: Allows applications to continue processing while waiting for I/O operations to complete.
- Selectors: Enable a single thread to monitor multiple channels for readiness to perform reading or writing.
- Buffers: Facilitate the management of data that is read from or written to channels.
For a deeper understanding of the NIO architecture, check out the official Java documentation: Java NIO Documentation.
The Problem with Blocking I/O
In traditional Java I/O, each I/O call can block the executing thread, waiting for data to be available. This blocking can lead to performance bottlenecks, especially in high-load scenarios where many threads are trying to access shared resources.
Common Pitfalls with Blocking
-
Thread Management Overhead:
- Creating large numbers of threads can lead to increased CPU context switching and thread management overhead.
-
Limited Scalability:
- Blocking I/O handles one request per thread, which means if you have 1000 concurrent users, you might end up creating 1000 threads. This is inefficient and limits scalability.
-
Latency Issues:
- The time taken to switch between threads can add significant latency, especially under high load.
-
Complexity of Handling Errors:
- Blocking code can lead to more complex error-handling paths, especially when dealing with timeouts or unavailable resources.
Leveraging Java NIO to Overcome Blocking Pitfalls
1. Use Non-Blocking Channels
Instead of using standard InputStream
or OutputStream
, utilize non-blocking channels provided by NIO.
import java.nio.channels.*;
import java.nio.*;
import java.net.*;
public class NonBlockingServer {
public static void main(String[] args) throws Exception {
Selector selector = Selector.open();
ServerSocketChannel serverSocket = ServerSocketChannel.open();
serverSocket.bind(new InetSocketAddress(1234));
serverSocket.configureBlocking(false);
serverSocket.register(selector, SelectionKey.OP_ACCEPT);
while (true) {
selector.select();
for (SelectionKey key : selector.selectedKeys()) {
if (key.isAcceptable()) {
SocketChannel client = serverSocket.accept();
client.configureBlocking(false);
client.register(selector, SelectionKey.OP_READ);
}
if (key.isReadable()) {
SocketChannel client = (SocketChannel) key.channel();
// Handle reading from the client...
}
}
selector.selectedKeys().clear();
}
}
}
Why this code works:
- By setting the server socket to non-blocking mode, you can handle multiple connections simultaneously without blocking the main thread.
- This not only improves the application's performance but also significantly reduces the resource overhead associated with thread management.
2. Use Selectors
Selectors are the heart of the NIO non-blocking architecture. They allow a single thread to manage multiple channels, enabling the efficient utilization of resources.
// Inside the while loop of NonBlockingServer
selector.select();
Why use a Selector?
- They enable multiplexing I/O operations. One thread can effectively monitor multiple channels, which reduces the overall number of threads needed and enhances scalability.
3. Handle Read and Write in Separate Methods
To maintain clean code and prevent blocking, handle I/O operations in separate methods. This method ensures you aren’t stuck waiting for a read or write operation to complete.
private void handleRead(SocketChannel client) {
ByteBuffer buffer = ByteBuffer.allocate(256);
int bytesRead = client.read(buffer);
// Continue processing...
}
Why this structure?
- Keeping read and write logic isolated makes your code more manageable and allows for more precise error handling. Each method can gracefully handle potential exceptions that may occur during I/O operations.
4. Implement Asynchronous File I/O
Java NIO also offers asynchronous file channels, allowing file read/write operations to be performed without blocking.
import java.nio.channels.AsynchronousFileChannel;
import java.nio.file.Paths;
AsynchronousFileChannel channel = AsynchronousFileChannel.open(Paths.get("file.txt"));
channel.read(buffer, 0, null, new CompletionHandler<Integer, Object>() {
@Override
public void completed(Integer result, Object attachment) {
// Handle successful read
}
@Override
public void failed(Throwable exc, Object attachment) {
exc.printStackTrace();
}
});
Why use asynchronous file I/O?
- Using asynchronous operations allows applications to continue processing while waiting for file operations to complete, which dramatically improves performance, especially for I/O-bound applications.
5. Manage Resource Cleanup Properly
Failure to close channels and buffers can lead to resource leaks. Always ensure that resources are cleaned up properly.
try {
// Perform I/O operations
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (channel != null) channel.close();
if (buffer != null) buffer.clear();
} catch (IOException e) {
e.printStackTrace();
}
}
Why resource management is crucial:
- Proper resource management ensures the application runs smoothly without exhausting system resources. Memory leaks can be hard to trace and lead to unpredictable application behavior.
To Wrap Things Up
Understanding and embracing the power of Java NIO can significantly elevate the performance and scalability of your applications. By transitioning away from blocking I/O practices, implementing non-blocking channels, utilizing selectors effectively, and managing resources appropriately, you can sidestep many common pitfalls and build efficient Java applications.
For more extensive insights into Java NIO and how to leverage its capabilities, do check out the in-depth resources available on Baeldung or JavaTpoint.
By mastering these techniques, you can ensure that your applications remain responsive and performant, even under heavy load. Begin implementing these strategies today, and watch your Java applications reach new heights.
Checkout our other articles