Overcoming Java's High Precision Time Measurement Challenges
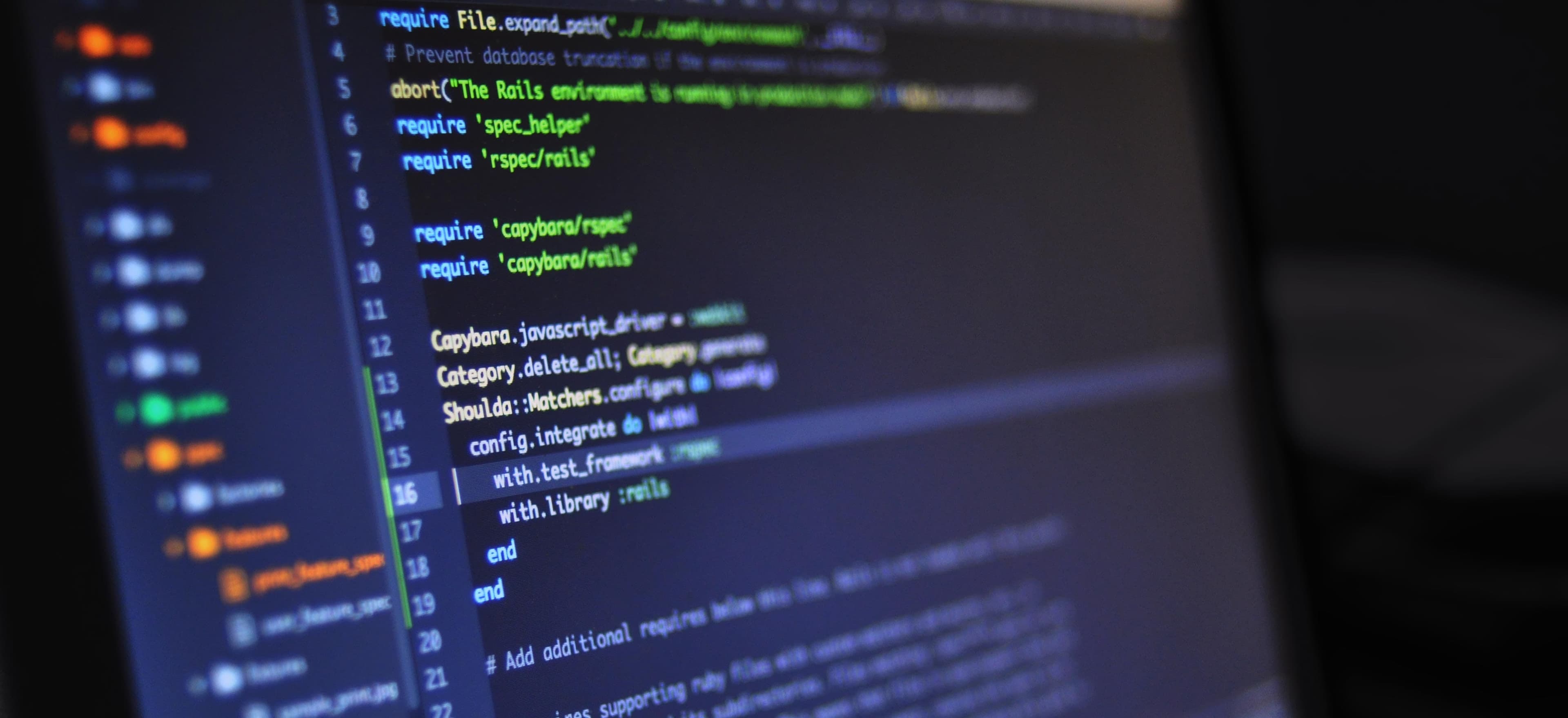
- Published on
Overcoming Java's High Precision Time Measurement Challenges
In the world of software development, accurate time measurement is crucial. Performance monitoring, benchmarking, and profiling are just a few areas that demand high precision. Java, a robust, platform-independent programming language, provides several mechanisms for time measurement. However, developers encounter challenges when seeking higher precision. In this blog post, we will explore effective strategies to overcome Java's high precision time measurement challenges, highlighting best practices along the way.
Understanding the Importance of High Precision Timing
Before diving into the specifics, it's essential to understand why high precision time measurement is important.
- Performance Testing: Accurate measurement of execution time helps identify bottlenecks in the code.
- Benchmarking: Delivering reliable benchmarks is vital for comparing different algorithms or pieces of software.
- Real-Time Systems: For systems that respond to events instantly, precise timing is non-negotiable.
Java provides multiple ways to measure time, including System.currentTimeMillis()
and System.nanoTime()
. While the former is suitable for timestamps, the latter offers nanosecond precision, crucial for performance-sensitive applications.
The Challenges Ahead
While System.nanoTime()
provides a relatively high level of precision, there are challenges, including:
- Clock Resolution: Not all hardware guarantees the same precision.
- Garbage Collection: Java's environment can introduce variability into timing measurements.
- JIT Compilation: Just-In-Time compilation can enhance performance unpredictably.
Let’s take a deeper look into overcoming these challenges.
Using System.nanoTime()
For fine-grained performance measurement, System.nanoTime()
is generally the preferred option over System.currentTimeMillis()
.
Here’s a simple code snippet to illustrate its use:
public class TimingExample {
public static void main(String[] args) {
long startTime = System.nanoTime();
// Code block to measure
performComputation();
long endTime = System.nanoTime();
long duration = endTime - startTime;
System.out.println("Execution Time: " + duration + " nanoseconds");
}
private static void performComputation() {
// Simulated computation task
for (int i = 0; i < 1_000_000; i++) {
Math.sqrt(i);
}
}
}
Why Use System.nanoTime()?
System.nanoTime()
gives you access to a high-resolution time source. Unlike currentTimeMillis()
, which is only accurate to milliseconds, nanoTime()
can measure intervals in nanoseconds, making it ideal for benchmarking.
Note on Clock Resolution
While System.nanoTime()
is more precise, the underlying clock's resolution can vary by hardware. Ensuring that your testing environment (CPU, OS, etc.) is optimized for precision is crucial. For in-depth information about the specification and behavior of nanoTime()
, check the Java documentation.
Considerations for Garbage Collection
Garbage Collection (GC) can significantly interfere with time measurements, especially for long-running processes. When GC events occur, they may skew the statistics, leading to inaccurate results. Here are some ways to mitigate these effects:
Use Warm-Up Iterations
Plan to run multiple iterations of your code block before measuring. This approach lets the JVM optimize the code and potentially run the GC ahead of time.
public class GCExample {
public static void main(String[] args) {
// Warm-up iterations
for (int i = 0; i < 10; i++) {
performComputation();
}
// Actual timing measurement
long startTime = System.nanoTime();
performComputation();
long endTime = System.nanoTime();
long duration = endTime - startTime;
System.out.println("Execution Time: " + duration + " nanoseconds");
}
private static void performComputation() {
for (int i = 0; i < 1_000_000; i++) {
Math.sqrt(i);
}
}
}
Why Warm-Up?
The JVM employs various optimization techniques, such as JIT compilation, that require some time to kick in. By executing warm-ups, you can achieve a more stable timing result, reflecting the optimized performance rather than an unoptimized state.
JIT Compilation and Its Impact
Java's Just-In-Time (JIT) compilation can introduce variability in your measurements during benchmarking. In some cases, the JIT may optimize the code after a few runs, leading to inconsistent results across subsequent executions.
Benchmarking Frameworks
To streamline the benchmarking process and mitigate the impact of JIT compilation, you can rely on established frameworks like JMH (Java Microbenchmark Harness), which is specifically designed for micro-benchmarking.
Here's a basic example of using JMH:
import org.openjdk.jmh.annotations.*;
import java.util.concurrent.TimeUnit;
@BenchmarkMode(Mode.AverageTime)
@OutputTimeUnit(TimeUnit.NANOSECONDS)
public class JMHExample {
@Benchmark
public void testComputation() {
for (int i = 0; i < 1_000_000; i++) {
Math.sqrt(i);
}
}
}
Why Use JMH?
The JMH framework handles warm-ups, iterations, and reporting, ensuring that results are more reliable and easier to interpret. For more detailed insights, read about JMH on GitHub.
Concluding Thoughts
Java provides robust mechanisms for high precision time measurement, but challenges remain. By leveraging System.nanoTime()
, being mindful of garbage collection, and utilizing benchmarking frameworks like JMH, developers can ensure more accurate and reliable performance measurements.
For those looking to delve deeper into performance tuning, consider exploring other related topics within the Java ecosystem. There is vast knowledge available on Java performance.
By understanding these nuances and implementing best practices, engineers can navigate the complexities of high precision timing in Java successfully.
By honing in on the best practices for timing and measurement, you can significantly enhance the performance monitoring capabilities of your Java applications. Let’s embrace precision and ensure our software runs efficiently!
Checkout our other articles