Understanding TCP vs UDP: The Key Differences Explained
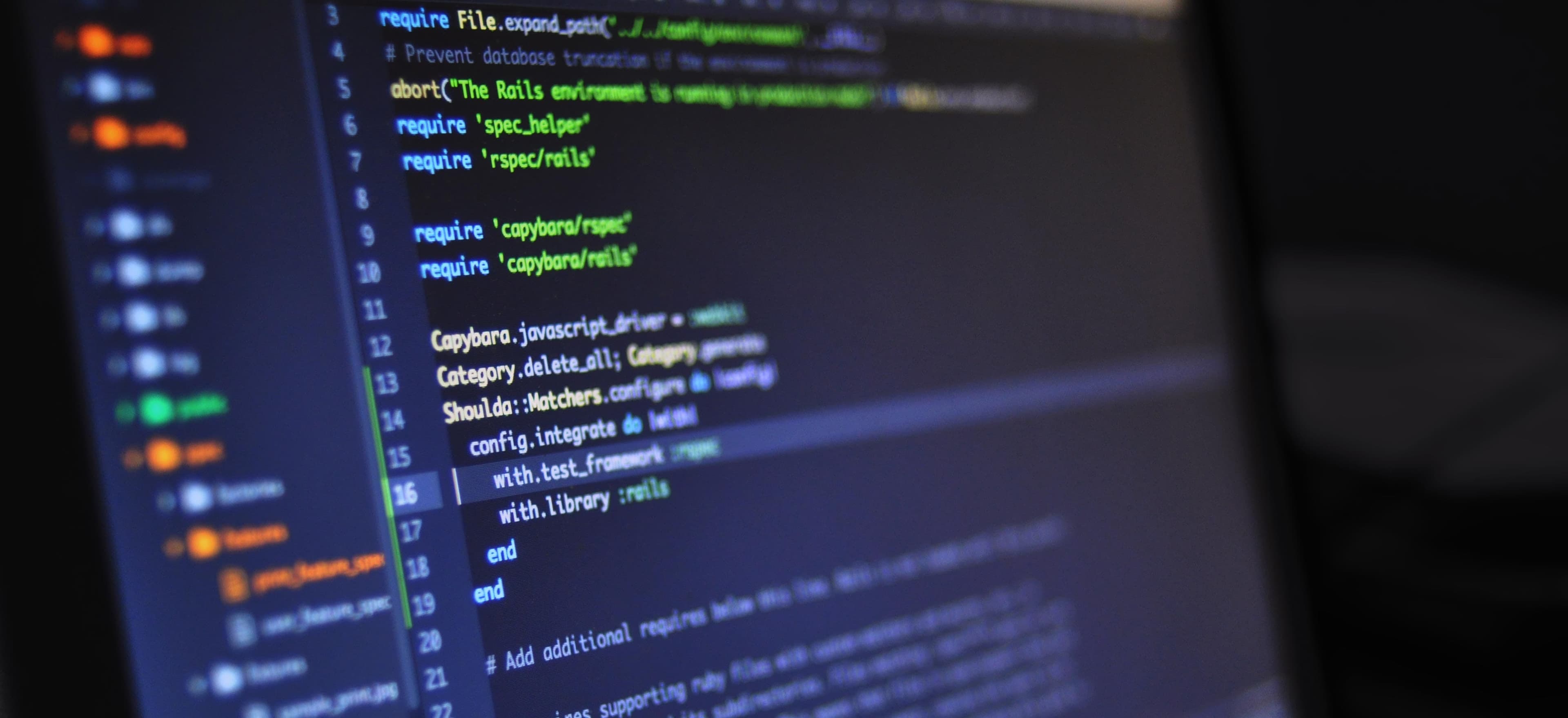
- Published on
Understanding TCP vs UDP: The Key Differences Explained
In the world of networking, two fundamental protocols reign supreme: Transmission Control Protocol (TCP) and User Datagram Protocol (UDP). Both are essential for data transmission over the internet, yet they serve different purposes and have distinct characteristics. Understanding these differences can help developers choose the right protocol for their specific needs.
In this post, we’ll explore the characteristics, advantages, and disadvantages of both TCP and UDP, and provide relevant code snippets to illustrate their differences.
1. What is TCP?
1.1 Overview
TCP, or Transmission Control Protocol, is a connection-oriented protocol designed to ensure reliable communication between devices. It establishes a dedicated end-to-end connection before data transmission, ensuring that packets arrive correctly and in order.
1.2 Key Features
- Connection-Oriented: TCP establishes a connection using a three-way handshake before data is sent.
- Reliability: It guarantees the delivery of data packets through error checking and retransmission.
- Data Integrity: TCP will reassemble packets in the correct order and account for any loss.
- Flow Control: It uses sliding window mechanisms to prevent the receiver from being overwhelmed with too much data at once.
1.3 TCP Connection Example
import java.io.*;
import java.net.*;
public class TCPClient {
public static void main(String[] args) {
String serverAddress = "localhost"; // address of the server
int port = 12345; // port number on which the server listens
try (Socket socket = new Socket(serverAddress, port);
PrintWriter out = new PrintWriter(socket.getOutputStream(), true);
BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()))) {
out.println("Hello Server!"); // sending a message
String response = in.readLine(); // waiting for the server's response
System.out.println("Server: " + response); // print the response
} catch (IOException e) {
e.printStackTrace(); // handle potential errors
}
}
}
Explanation: In this example, a TCP client connects to a server. The client uses a Socket object to establish a connection with the server's IP address and port. It sends a message and waits for the server’s response. The PrintWriter and BufferedReader handle sending and receiving data, ensuring reliable communication.
2. What is UDP?
2.1 Overview
UDP, or User Datagram Protocol, is a connectionless protocol that emphasizes speed and efficiency over reliability. While it does not guarantee delivery like TCP, it is ideal for applications where speed is critical, and dropped packets can be tolerated.
2.2 Key Features
- Connectionless: There is no prior connection established. Data is sent without any handshake.
- Speed: UDP is faster due to less overhead; it transmits data quickly without waiting for acknowledgment.
- No Reliability: UDP does not guarantee packet delivery or order. Lost packets are simply discarded.
- Simple Error Checking: Uses checksum for error detection but does not handle retransmissions.
2.3 UDP Connection Example
import java.io.*;
import java.net.*;
public class UDPClient {
public static void main(String[] args) {
String serverAddress = "localhost"; // server address
int port = 12345; // port number
try (DatagramSocket socket = new DatagramSocket()) {
String message = "Hello Server!";
byte[] buffer = message.getBytes();
DatagramPacket packet = new DatagramPacket(buffer, buffer.length, InetAddress.getByName(serverAddress), port);
socket.send(packet); // send the packet
System.out.println("Packet sent: " + message);
} catch (IOException e) {
e.printStackTrace(); // handle potential errors
}
}
}
Explanation: This code demonstrates a UDP client that sends a message to a server. The DatagramSocket is created for communication, and a DatagramPacket is formed with the message. Unlike TCP, no acknowledgment is awaited, allowing for faster operations.
3. TCP vs UDP: Key Differences
Here’s a concise table summarizing the fundamental differences between TCP and UDP:
| Feature | TCP | UDP | |------------------------|---------------------------------|--------------------------| | Connection Type | Connection-oriented | Connectionless | | Reliability | Reliable (guaranteed delivery) | Unreliable (no delivery guarantee) | | Order of Delivery | Ordered | No guaranteed order | | Speed | Slower due to overhead | Faster due to less overhead | | Use Cases | Web browsing, file transfers | Streaming, gaming |
4. When to Use TCP vs UDP
4.1 When to Use TCP
- Web Applications: Applications that require reliable delivery of data, such as web pages and file downloads.
- Email: Protocols like SMTP and IMAP rely on TCP for ensuring messages are sent and received correctly.
- File Transfer Protocols (FTP): TCP ensures all parts of a file are received correctly for accurate transfers.
4.2 When to Use UDP
- Online Gaming: The speed is more critical than reliability, and users can tolerate occasional packet loss.
- Voice over IP (VoIP): Real-time communication is prioritized over data integrity.
- Live Streaming: Continual playback with slight adjustments outweighs waiting for lost packets to be resent.
5. Conclusion
In networking, the choice between TCP and UDP is dictated by the specific requirements of the application. TCP is best suited for applications where reliability is crucial, while UDP is appropriate for scenarios demanding speed and efficiency over complete accuracy.
Understanding these protocols will empower developers to make informed decisions and optimize their applications for performance and user experience.
Additional Resources
Here are some helpful links for deeper understanding:
- TCP/IP Protocol Suite - GeeksforGeeks
- User Datagram Protocol Explained - Microsoft Docs
- Understanding TCP and UDP - Network World
By grasping the essence of TCP and UDP, developers can not only enhance application performance but also tailor the user experience based on the needs of their target audience. Happy coding!
Checkout our other articles