Top Challenges in Running EJBs on Different Platforms
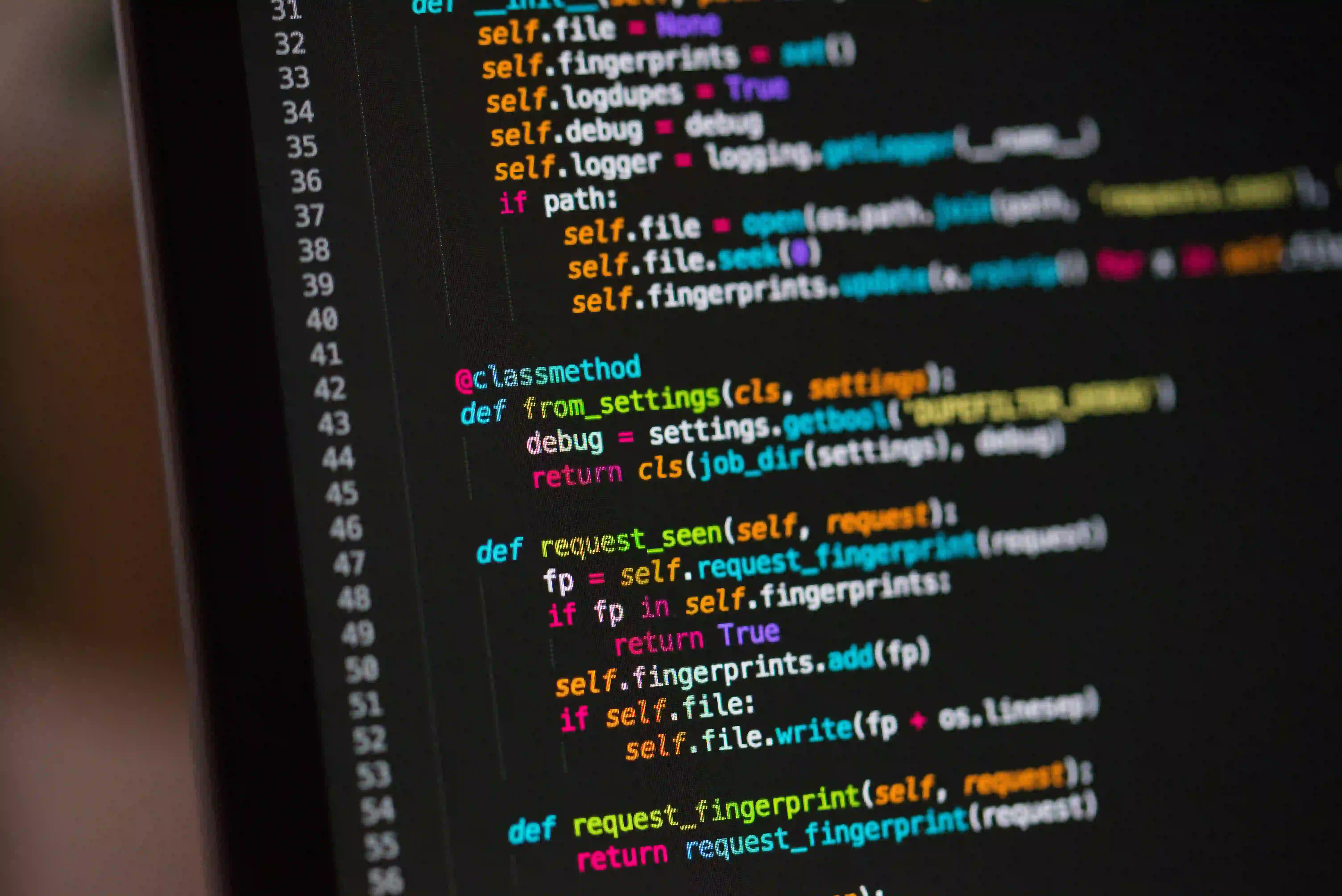
Top Challenges in Running EJBs on Different Platforms
Enterprise JavaBeans (EJB) technology has become a cornerstone for building large-scale enterprise applications. While the power of EJBs is undeniable, running them across different platforms presents unique challenges. In this blog post, we will explore these challenges in detail and understand how to overcome them.
1. Understanding EJB Architecture
Before diving into the challenges, it's critical to grasp the basics of EJB architecture. An Enterprise Java Bean is a server-side component that encapsulates business logic of an application. EJBs can be categorized into three types:
- Session Beans: Maintain a conversational state with clients.
- Entity Beans: Represent persistent data.
- Message-driven Beans: Handle asynchronous processing.
Each type serves specific use cases, making EJBs versatile but also complex.
2. Deployment Complexity
One of the most significant challenges in running EJBs on different platforms is dealing with deployment complexities. Each application server (like JBoss, WebLogic, or GlassFish) may have its own set of deployment descriptors, which could lead to compatibility issues.
Solution:
To mitigate deployment challenges, adhere to standardized API use and convention over configuration principles. By following published standards, you can ensure that your EJBs remain portable.
// An example of a simple stateless session bean
import javax.ejb.Stateless;
@Stateless
public class MyService {
public String greet(String name) {
return "Hello, " + name;
}
}
In the code snippet above, the @Stateless
annotation makes the EJB portable across compliant servers.
3. Database Compatibility
When EJBs need to connect to different databases, discrepancies in SQL dialects can cause issues. A feature that works in one database may not be applicable in another.
Solution:
Utilize Java Persistence API (JPA) to abstract database interactions. This approach allows developers to focus on entity relationships rather than database specifics.
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
@Entity
public class User {
@Id
@GeneratedValue
private Long id;
private String name;
// Getters and setters
}
In the above code, the @Entity
annotation defines a JPA entity. JPA streamlines database management, allowing automatic translation of SQL queries based on the underlying database.
4. Application Server Variability
Each application server implements the EJB specification differently. This variability can lead to features being unsupported or implemented in ways that create additional overhead.
Solution:
Always reference the official EJB specifications and utilize testing to ensure compatibility across servers.
5. Performance Issues
EJBs may introduce overhead due to their nature of remote calls and transactional management. Performance can become a concern, particularly when the application is not optimized for the server environment.
Solution:
To optimize performance:
- Choose the right bean type: For short-lived operations, prefer stateless session beans.
- Limit remote method calls: Minimize the number of remote calls by batching operations whenever possible.
@EJB
private MyService myService;
public void performOperation() {
String greeting = myService.greet("John");
// Process greeting...
}
In this example, the @EJB
annotation is used to inject an EJB, but it is crucial to consider performance—too many remote calls can degrade application performance.
6. Security Models
Different platforms provide varying levels of security configurations, which can lead to inconsistent behaviors.
Solution:
Use Java EE's built-in security features to handle authentication and authorization. Implementing role-based access control through annotations can help maintain consistent security practices.
import javax.annotation.security.RolesAllowed;
@RolesAllowed("admin")
public void adminMethod() {
// Admin specific logic
}
This code ensures that only users with the "admin" role can invoke adminMethod()
, providing a simple yet effective way to handle security.
7. Testing Challenges
Testing EJBs can be a double-edged sword. While JUnit and Arquillian provide useful tools, testing in diverse environments raises concerns about consistency and results.
Solution:
Incorporate integration testing into your build pipeline. Use Arquillian, a testing platform for Java EE, which allows you to run tests inside containers.
@RunWith(Arquillian.class)
public class MyServiceTest {
@Inject
private MyService myService;
@Test
public void testGreet() {
String result = myService.greet("Alice");
assertEquals("Hello, Alice", result);
}
}
The use of Arquillian in the code snippet sets up a testing environment where EJB business logic can be validated consistently across environments.
8. Logging and Monitoring
Monitoring EJB applications can be difficult, particularly when logging across multiple platforms. Incorrectly configured logging can lead to performance bottlenecks and inefficient debugging.
Solution:
Adopt a centralized logging framework. Tools like Log4j or SLF4J can unify logging practices.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@Stateless
public class MyService {
private static final Logger logger = LoggerFactory.getLogger(MyService.class);
public String greet(String name) {
logger.info("Greeting user {}", name);
return "Hello, " + name;
}
}
In this example, we use SLF4J to log messages, which can be configured to send logs to a central server for monitoring.
The Bottom Line
Running EJBs on different platforms presents various challenges, but these can be addressed using standard practices, utilizing appropriate technologies, and embracing effective development methodologies. By employing the strategies outlined in this post, you can ensure that your EJB applications remain robust, efficient, and scalable no matter the environment.
For further reading, you may refer to Oracle's EJB Documentation and Java EE 8: Application Development for a deeper understanding of enterprise application development.
Whether you're just getting started with EJBs or are facing compatibility issues in a diverse deployment landscape, remember that the strategies to navigate these many challenges are just as important as the underlying technology itself. Happy coding!