Understanding Thread Visibility in Java Concurrency
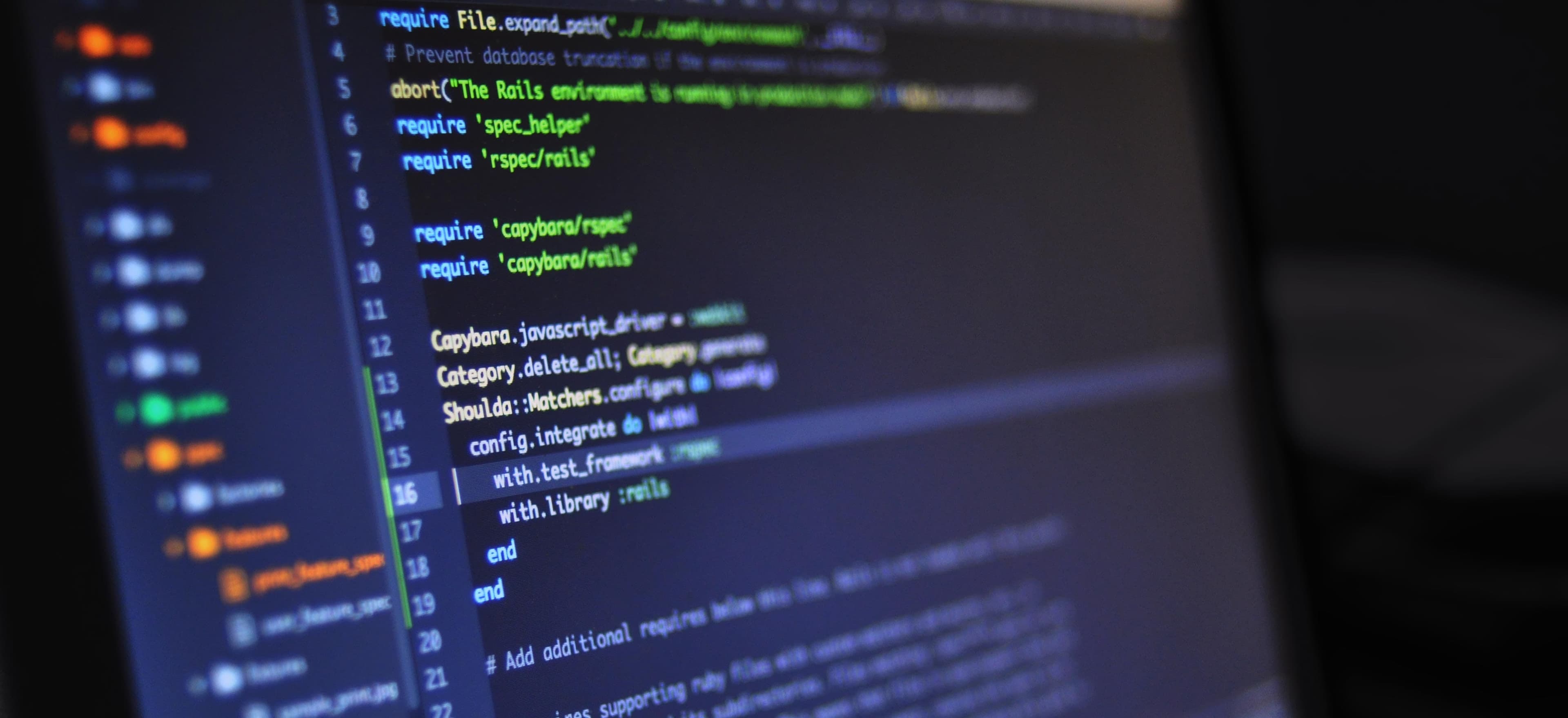
- Published on
Understanding Thread Visibility in Java Concurrency
Java concurrency is a vast field, allowing developers to write efficient multi-threaded applications. One of the key concepts in Java concurrency is thread visibility. This involves ensuring that changes made to shared variables in one thread are visible to other threads. In this blog post, we'll explore how thread visibility works, the issues tied to it, the role of synchronization, and some practical code examples to gracefully handle this complexity.
What is Thread Visibility?
Thread visibility refers to the guarantee that when one thread modifies a shared variable, all other threads will see the latest modification. Without appropriate synchronization, threads may cache values locally or not see changes made by others.
The Java Memory Model
To understand thread visibility, you need to be familiar with the Java Memory Model (JMM). The JMM specifies how threads interact through memory and what behaviors are allowed, particularly concerning reading and writing shared variables.
When a variable is made visible across threads, it ensures that:
- Changes made in one thread are immediately reflected in other threads.
- Memory reads/writes follow a defined order, thus preventing subtle bugs.
The Problem: Cache and Local Copies
Threads in Java can create local copies of shared variables stored in CPU caches. Suppose one thread updates a shared variable:
public class Counter {
private int count = 0;
public void increment() {
count++; // Non-atomic operation
}
public int getCount() {
return count;
}
}
In the example above, if multiple threads call increment()
, they might not see the updated count due to caching. This could lead to erroneous behavior, where some increments are lost.
Solutions to Ensure Visibility
1. Using the volatile
Keyword
The easiest way to ensure thread visibility is through the volatile
keyword. When a variable is declared as volatile, it guarantees that any read of that variable will return the most recent write by any thread.
Here’s how you can apply the volatile
keyword in our previous example:
public class VolatileCounter {
private volatile int count = 0;
public void increment() {
count++; // Still not atomic but ensures visibility
}
public int getCount() {
return count;
}
}
Using volatile
ensures that when one thread modifies count
, the change becomes immediately visible to other threads. However, keep in mind that volatile
does not make operations atomic. If you need atomicity for operations, use synchronization or other constructs.
2. Synchronized Blocks
Another way to ensure thread visibility is with synchronized blocks or methods. When a thread enters a synchronized block, it obtains a lock, preventing other threads from executing any synchronized block that shares the same lock.
Here’s a revised version using synchronized methods:
public class SynchronizedCounter {
private int count = 0;
public synchronized void increment() {
count++;
}
public synchronized int getCount() {
return count;
}
}
Using synchronized
ensures that once a thread modifies count
, the value is flushed to main memory, making it visible to other threads as they acquire the lock.
3. Atomic Classes
If you require atomic operations along with visibility guarantees, consider using classes provided in the java.util.concurrent.atomic
package, such as AtomicInteger
.
Here’s an example:
import java.util.concurrent.atomic.AtomicInteger;
public class AtomicCounter {
private AtomicInteger count = new AtomicInteger(0);
public void increment() {
count.incrementAndGet(); // Atomic operation
}
public int getCount() {
return count.get();
}
}
Using AtomicInteger
, we get both atomicity and visibility guarantees without the need for explicit synchronization blocks.
Performance Considerations
When designing a multi-threaded application, performance matters. Overusing synchronization can lead to contention and performance bottlenecks. Benchmarking different approaches is essential to determine the right balance for your application.
- Volatile: Best for simple visibility and not for complex operations.
- Synchronized blocks: Good for encapsulating complex logic while maintaining thread safety.
- Atomic classes: Optimal for operations requiring atomicity without heavy locking.
Example: Comparing Approaches
Let’s delve into an example that employs the aforementioned techniques in a simple multi-threaded incrementing scenario:
public class ThreadVisibilityExample {
private static final int NUM_THREADS = 10;
private static final int NUM_INCREMENTS = 1000;
public static void main(String[] args) throws InterruptedException {
final SynchronizedCounter syncCounter = new SynchronizedCounter();
final AtomicCounter atomicCounter = new AtomicCounter();
// Using synchronized counter
runIncrementThreads(syncCounter);
// Using atomic counter
runIncrementThreads(atomicCounter);
}
private static void runIncrementThreads(SynchronizedCounter counter) throws InterruptedException {
Thread[] threads = new Thread[NUM_THREADS];
for (int i = 0; i < NUM_THREADS; i++) {
threads[i] = new Thread(() -> {
for (int j = 0; j < NUM_INCREMENTS; j++) {
counter.increment();
}
});
threads[i].start();
}
for (Thread thread : threads) {
thread.join();
}
System.out.println("Final count with SynchronizedCounter: " + counter.getCount());
}
private static void runIncrementThreads(AtomicCounter counter) throws InterruptedException {
Thread[] threads = new Thread[NUM_THREADS];
for (int i = 0; i < NUM_THREADS; i++) {
threads[i] = new Thread(() -> {
for (int j = 0; j < NUM_INCREMENTS; j++) {
counter.increment();
}
});
threads[i].start();
}
for (Thread thread : threads) {
thread.join();
}
System.out.println("Final count with AtomicCounter: " + counter.getCount());
}
}
In the above implementation, we create two counters and run multiple threads incrementing each. The results show how methods differ in terms of performance when dealing with threading.
To Wrap Things Up
Thread visibility is a foundational aspect of Java concurrency that can significantly impact application behavior. By understanding how the Java Memory Model works and utilizing mechanisms like volatile
, synchronization, and atomic classes, developers can avoid common pitfalls associated with multi-threaded programming.
For further reading on Java concurrency, consider checking out these resources:
- Java Concurrency in Practice
- The Java Memory Model - Official Oracle Documentation
Arming yourself with this knowledge will enable you to write more efficient, safe, and high-performing multi-threaded applications. Embrace the power of concurrency and let your Java code run smoothly in a multi-threaded world!
Checkout our other articles