Improve Java Performance with Efficient Database Querying
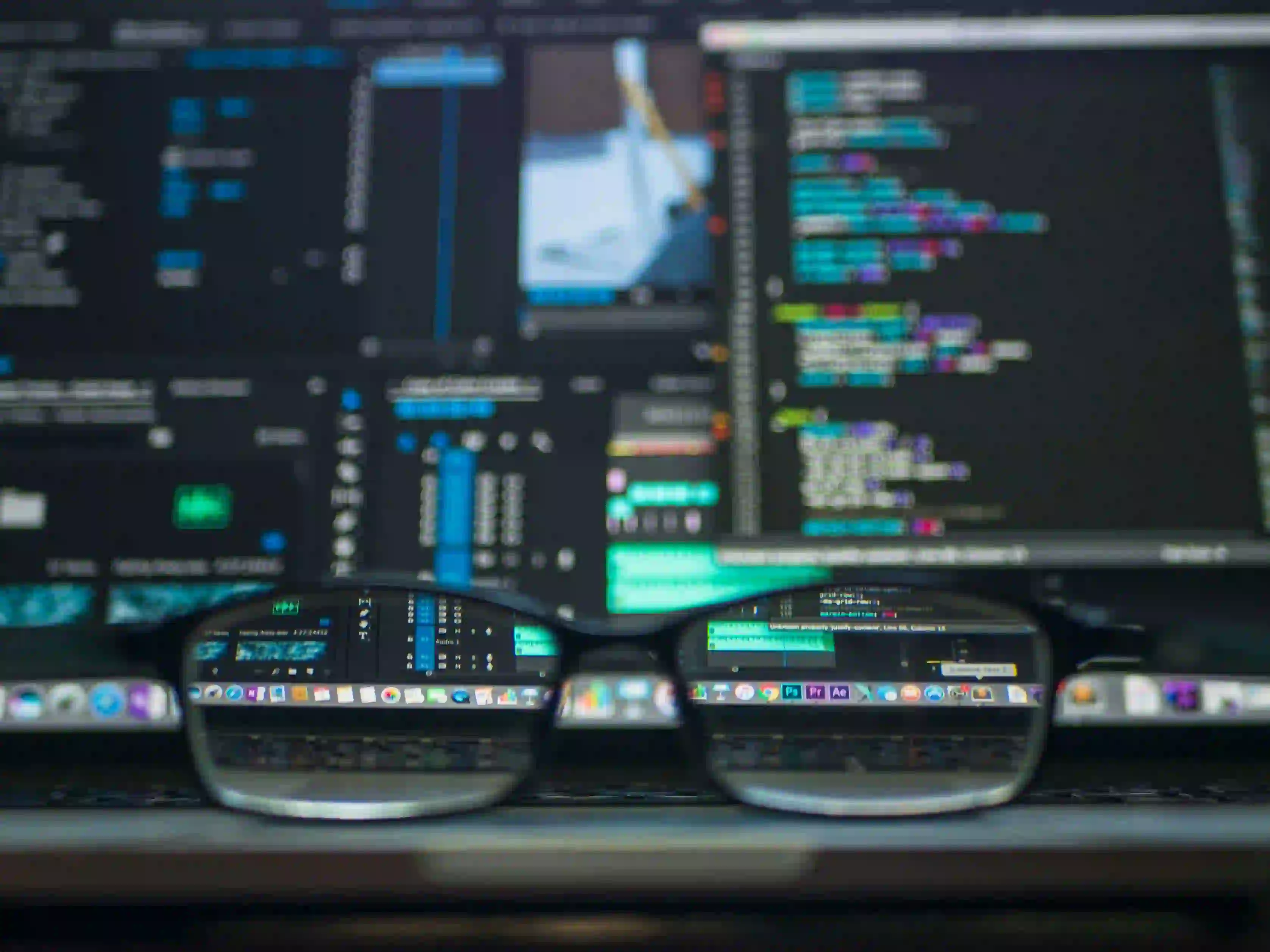
Improve Java Performance with Efficient Database Querying
In the realm of software development, an efficient application is not only about sleek code and responsive user interfaces. A significant aspect of performance hinges on effective database querying, especially when utilizing Java as your primary programming language. This blog post will explore strategies and best practices to optimize database interactions in Java applications.
Understanding the Significance of Database Querying
Databases serve as the backbone of applications. They store, retrieve, and manage data, making them essential for performance. In Java, inefficient database queries can lead to bottlenecks, significantly affecting the user experience. When queries take too long, application responsiveness is compromised.
Why Optimize Database Queries?
- User Experience: Faster queries translate to smoother application performance, resulting in happier users.
- Resource Utilization: Optimizing your queries minimizes CPU and memory usage, which can reduce costs in cloud environments.
- Scalability: Well-optimized queries can handle increased loads without requiring immediate architectural changes.
Best Practices for Efficient Database Querying in Java
1. Use Prepared Statements
Prepared statements are precompiled SQL statements that can be executed multiple times with different parameters. By using prepared statements, you can not only improve performance but also enhance security by protecting against SQL injection attacks.
String sql = "SELECT * FROM users WHERE username = ?";
try (PreparedStatement pstmt = connection.prepareStatement(sql)) {
pstmt.setString(1, "john_doe");
ResultSet rs = pstmt.executeQuery();
while (rs.next()) {
System.out.println("Username: " + rs.getString("username"));
}
} catch (SQLException e) {
e.printStackTrace();
}
Why use Prepared Statements? They reduce execution time and allow for parameterized queries, which help in maintaining the integrity and security of your application.
2. Optimize Your Queries
Not all queries are created equal. Analyzing and optimizing your SQL queries can have a dramatic impact on performance.
-
Use EXPLAIN: Use the
EXPLAIN
command in SQL to check how your database executes the query. This can help identify bottlenecks. -
Indexes: Ensure that proper indexes are created for columns frequently used in WHERE clauses.
For example, if you often search by username, consider indexing that column:
CREATE INDEX idx_username ON users(username);
Why it matters: Indexes drastically speed up data retrieval times, especially in large datasets. However, be careful—too many indexes can slow down write operations.
3. Batch Processing
When dealing with multiple inserts or updates, consider using batch processing. This reduces the number of database round trips and can significantly improve performance.
try (Statement stmt = connection.createStatement()) {
connection.setAutoCommit(false); // Turn off auto-commit
for (String user : users) {
stmt.addBatch("INSERT INTO users (username) VALUES ('" + user + "')");
}
stmt.executeBatch(); // Execute all at once
connection.commit(); // Commit the transaction
} catch (SQLException e) {
e.printStackTrace();
}
Why use batch processing? It consolidates multiple SQL operations into a single batch execution, decreasing network traffic and improving performance.
4. Connection Pooling
Managing database connections can be expensive in terms of resources. Connection pooling allows you to reuse existing connections rather than continuously opening and closing them.
Libraries such as HikariCP or Apache DBCP simplify connection pooling in Java applications.
<dependency>
<groupId>com.zaxxer</groupId>
<artifactId>HikariCP</artifactId>
<version>4.0.3</version>
</dependency>
Why use connection pooling? This approach minimizes the overhead of establishing a new connection for each database request and significantly enhances throughput.
5. Analyze and Refine Queries
Refining queries is a continuous process. Combine insights from database analytics with application performance metrics to identify slow queries and areas for improvement.
Application Performance Management (APM) tools such as New Relic, Datadog, or AppDynamics can provide invaluable information.
Here’s a simple performance monitoring code snippet:
long startTime = System.currentTimeMillis();
try (Statement stmt = connection.createStatement()) {
ResultSet rs = stmt.executeQuery("SELECT * FROM large_table");
while (rs.next()) {
// process results
}
} finally {
long endTime = System.currentTimeMillis();
System.out.println("Query executed in: " + (endTime - startTime) + " ms");
}
Why is performance monitoring essential? It helps establish a baseline for query execution times and pinpoints inefficiencies.
6. Leverage ORM Frameworks Wisely
Object-Relational Mapping (ORM) frameworks like Hibernate can simplify database access and improve development speed. However, improper use can lead to performance issues.
Examples of common pitfalls include:
- Loading too much data (N+1 select issue).
- Using inefficient fetch strategies.
Here’s an example of a correctly set up fetch strategy:
@Entity
@NamedEntityGraph(
name = "User.detail",
attributePaths = { "profile", "address" }
)
public class User {
// fields and methods
}
Why use Entity Graphs? They allow for optimized fetching of related entities when you only need a specific subset of properties.
7. Cache Frequently Accessed Data
Caching frequently accessed data reduces the number of repetitive database queries and improves application performance.
You can implement caching solutions such as Redis or Ehcache alongside your Java application.
Example Code:
Cache<String, User> userCache = CacheBuilder.newBuilder()
.expireAfterWrite(10, TimeUnit.MINUTES)
.maximumSize(1000)
.build();
User user = userCache.getIfPresent("john_doe");
if (user == null) {
user = getUserFromDatabase("john_doe");
userCache.put("john_doe", user);
}
Why caching works: It enhances data retrieval times, significantly improving application speed and reducing database load.
The Bottom Line
Optimizing database queries in Java applications is a multifaceted approach, involving careful attention to SQL syntax, connection management, and the application architecture itself. The techniques discussed—from using prepared statements to caching frequently accessed data—are foundational to improving performance.
For further insights into enhancing query performance, consider reading the article titled "Boost Your Queries: Master the Skip Scan in PostgreSQL." This article delves into advanced querying techniques that can be beneficial alongside Java optimizations.
By implementing the strategies outlined above, you can enhance the efficiency of your Java applications and provide an improved experience for your users. Remember, consistent monitoring and analysis are key to maintaining optimized performance over time.