Optimize Java Applications with Effective Database Querying
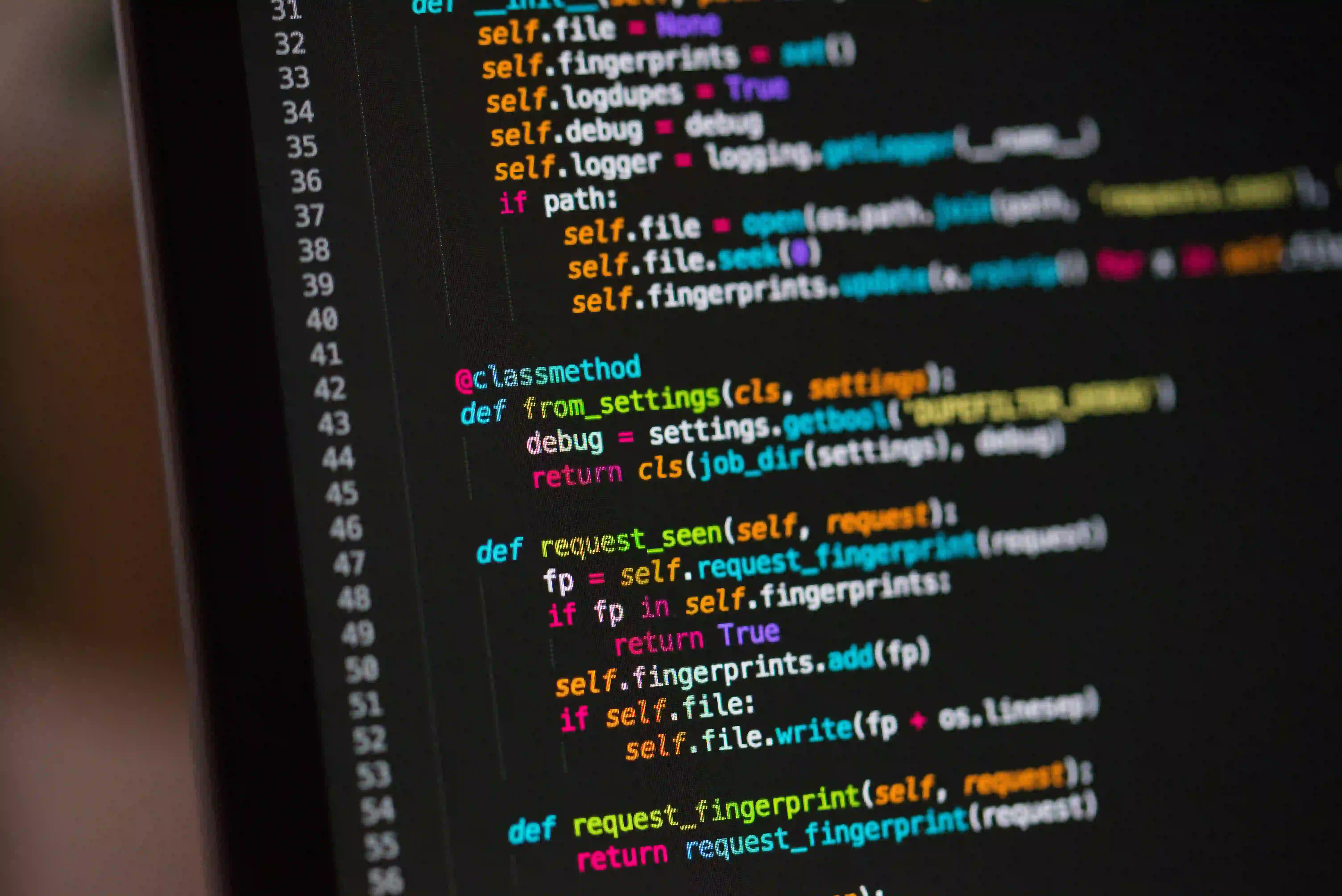
Optimize Java Applications with Effective Database Querying
In today's digital landscape, data is at the heart of application performance. For Java developers, understanding how to interact with databases efficiently is crucial. Poor database querying can lead to sluggish applications, frustrated users, and ultimately a loss of business opportunities. In contrast, efficient database queries can significantly enhance application performance and scalability.
Understanding the Basics of Database Querying
Before diving into optimization techniques, let's clarify what database querying entails. In essence, a database query is a request for data from a database. This is often done using SQL (Structured Query Language) in relational databases like PostgreSQL, MySQL, or Oracle.
Why Optimize Database Queries?
- Performance Enhancement: Faster queries mean quicker data retrieval and improved application responsiveness.
- Reduced Load on Database Server: Efficient queries minimize the strain on the database server, ensuring it can handle more requests.
- Cost Savings: Reduced resource utilization can lead to lower operational costs, especially with cloud-based databases.
A recent guide on Boost Your Queries: Master the Skip Scan in PostgreSQL delves into advanced querying techniques that can further enhance performance in PostgreSQL. While the article focuses on PostgreSQL, the principles discussed can broadly apply to Java applications interfacing with any relational database.
Key Strategies for Optimizing Database Queries in Java
1. Use Prepared Statements
Prepared statements not only enhance security by preventing SQL injection attacks, but they also improve performance for repeated queries.
String sql = "SELECT * FROM users WHERE username = ?";
try (PreparedStatement pstmt = connection.prepareStatement(sql)) {
pstmt.setString(1, "john_doe");
ResultSet rs = pstmt.executeQuery();
// Process the ResultSet
}
Why Use Prepared Statements?
- Efficiency: The database can cache the query plan, speeding up execution.
- Security: They help prevent SQL injection by separating code from data.
2. Optimize Your SQL Queries
Inefficient SQL queries can lead to poor application performance. Review your SQL codes and ensure you are adhering to best practices, such as:
- Using indexed columns in the WHERE clause.
- Avoiding SELECT * and fetching only necessary columns.
SELECT id, username FROM users WHERE status = 'active';
Why This Matters?
- Reducing the amount of data fetched decreases network latency and speeds up the response time.
3. Proper Indexing
Indexing is a powerful tool for speeding up data retrieval. When used correctly, indexes can drastically reduce search time.
CREATE INDEX idx_username ON users(username);
Why Is Indexing Important?
- It enhances query performance by allowing the database to find rows faster.
- However, over-indexing can have the opposite effect, slowing down write operations.
4. Connection Pooling
Managing database connections efficiently is vital for performance. Connection pooling allows you to reuse a pool of connections rather than opening and closing connections frequently.
Here's a simple code example using HikariCP for connection pooling:
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:postgresql://localhost:5432/mydatabase");
config.setUsername("user");
config.setPassword("password");
HikariDataSource dataSource = new HikariDataSource(config);
// Use the data source to get a connection
try (Connection conn = dataSource.getConnection()) {
// Use the connection
}
Why Connection Pooling?
- Allows applications to be more efficient in managing database connections, particularly under high load.
- Reduces overhead from establishing and tearing down connections.
5. Batch Processing
Batch processing reduces the number of round trips made to the database. Instead of executing individual queries, batch multiple operations into a single call.
String sql = "INSERT INTO users (username, password) VALUES (?, ?)";
try (PreparedStatement pstmt = connection.prepareStatement(sql)) {
for (User user : usersList) {
pstmt.setString(1, user.getUsername());
pstmt.setString(2, user.getPassword());
pstmt.addBatch();
}
pstmt.executeBatch(); // Execute all inserts at once
}
Why Batch Processing?
- It significantly minimizes the overhead of multiple network round trips, leading to faster performance.
6. Analyze Query Performance
Regular analysis of your SQL queries can surface performance bottlenecks. Most databases come equipped with tools to analyze query execution plans.
For example, in PostgreSQL, you can use:
EXPLAIN ANALYZE SELECT * FROM users WHERE username = 'john_doe';
What To Look For?
- Check for sequential scans or inefficient joins which can indicate that the query could be optimized.
7. Consider Using ORM Frameworks
Using Object-Relational Mapping (ORM) frameworks like Hibernate can simplify data manipulation and improve performance when configured correctly.
User user = session.get(User.class, userId);
Why ORM Frameworks?
- Abstract the complexities of direct SQL usage, making data operations easier to implement.
- When configured properly, ORMs can leverage advanced features like caching, which enhances performance.
Final Thoughts
Optimizing database querying in Java applications is not just about writing complex, elaborate queries. It’s about implementing best practices that facilitate smooth interaction with the database, improve performance, and provide a better overall user experience. By using prepared statements, optimizing SQL queries, properly indexing, utilizing connection pooling, batch processing, analyzing query performance, and considering ORM frameworks, developers can create highly efficient applications.
For diving deeper into the world of database optimization, the article "Boost Your Queries: Master the Skip Scan in PostgreSQL" provides compelling insights worth exploring. Remember, the better your database performance, the better your Java application will serve its users. Happy coding!