Seamlessly Integrating Java Animations for Web UI Enhancements
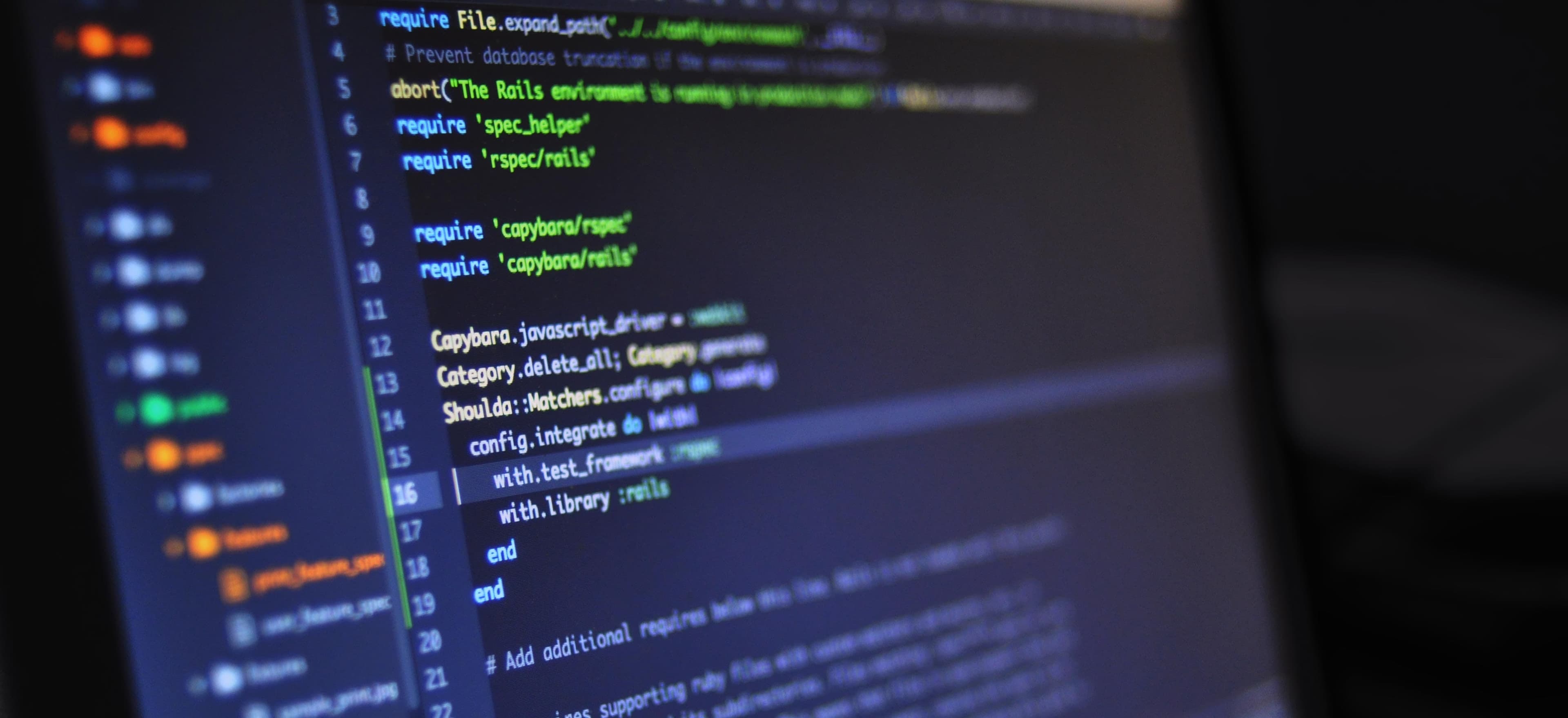
- Published on
Seamlessly Integrating Java Animations for Web UI Enhancements
In the ever-evolving landscape of web development, enhancing user experience (UX) is paramount. One effective method to achieve this is through the use of animations. Java, primarily known for its robustness in backend development, can also play a significant role in creating engaging animations on the web. This blog post will delve into how you can integrate Java animations into your web applications for improved UI experience, offering code snippets and useful insights along the way.
Why Use Animations in Web UI?
Animations serve various purposes in a web interface, including:
- Grabbing attention: They can highlight features or guide users through processes, making it easier for them to navigate the UI.
- Providing feedback: Animations help users understand that an action has been successfully completed (like form submissions).
- Creating a smoother experience: Subtle animations can ease transitions between different states of the application.
For a more thorough exploration of design principles around animations, check out Mastering Smooth Sliding Animations in Web Design.
Setting Up Java for Web Animations
Before we dive into specific examples, it's essential to ensure that you have the right environment. You'll need:
- JDK (Java Development Kit): Make sure you have the latest version installed.
- Web framework: You can use frameworks like Spring Boot to create REST APIs or JavaServer Faces (JSF) for full-fledged web applications.
- Frontend integrations: Libraries like jQuery or frameworks like React can manage animations by complementing Java on the backend.
Example 1: Simple Fade-In Animation with Java and CSS
This code snippet demonstrates a simple fade-in animation. Although most animations are handled in CSS on the client side, we will initiate the animation using Java for clarity.
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.*;
public class FadeInServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html><head><style>");
out.println("body { opacity: 0; transition: opacity 2s; }");
out.println("body.loaded { opacity: 1; }");
out.println("</style></head>");
out.println("<body onload=\"document.body.classList.add('loaded');\">");
out.println("<h1>Welcome to Our Web Application!</h1>");
out.println("</body></html>");
}
}
Commentary
-
CSS Animation: The fade-in effect is defined in the
<style>
section, setting the initial opacity to 0 and defining a transition effect for when it changes to 1. -
Java Servlet: Here, the servlet generates HTML content and injects a unique script that adds the
loaded
class to the<body>
element when the page is fully loaded. This triggers the CSS transition to fade in the content smoothly. -
Why it works: This approach separates concerns, allowing CSS to handle the animation seamlessly while Java can manage server-side logic and content rendering.
Example 2: Loading Spinner Using Java and JavaScript
For dynamic interfaces, displaying a loading spinner can significantly improve user perception of performance. This example shows how you can implement a spinner through Java backend integration with JavaScript for the UI.
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.*;
public class LoadingSpinnerServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html><head><style>");
out.println(".spinner { border: 4px solid #f3f3f3; border-top: 4px solid #3498db; border-radius: 50%; width: 50px; height: 50px; animation: spin 1s linear infinite; }");
out.println("@keyframes spin { 0% { transform: rotate(0deg); } 100% { transform: rotate(360deg); } }");
out.println("</style></head>");
out.println("<body onload=\"loadContent()\">");
out.println("<div class='spinner'></div>");
out.println("<div id='content' style='display:none;'><h1>Content Loaded!</h1></div>");
out.println("<script>");
out.println("function loadContent() { setTimeout(function() { document.querySelector('.spinner').style.display = 'none'; document.getElementById('content').style.display = 'block'; }, 3000); }");
out.println("</script>");
out.println("</body></html>");
}
}
Commentary
-
CSS for Spinner: The
.spinner
class defines a loading animation. The keyframes create a rotation effect for the spinner element. -
Java Servlet Logic: Initially displays the spinner, simulating a content load process for 3 seconds before revealing the main content.
-
JavaScript for Behavior: JavaScript controls when to hide the spinner and show the actual content. This method is straightforward and beneficial for asynchronous loading in modern web applications.
Integrating with Frontend Frameworks
While the examples above demonstrate vanilla Java and HTML integration, consider using frontend frameworks like Angular or React for more complex animations. Java can serve the backend APIs, while frontend frameworks handle user interaction and state management, leading to a smoother animation experience.
Using Java with React
Here is an example of how to manage animations using a Java backend with a React frontend:
Java REST API Example:
@RestController
@RequestMapping("/api")
public class AnimationController {
@GetMapping("/data")
public ResponseEntity<String> getData() {
try {
// Simulate data fetch.
Thread.sleep(2000);
return ResponseEntity.ok("Data fetched successfully!");
} catch (InterruptedException e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).build();
}
}
}
React Example:
import React, { useEffect, useState } from 'react';
import './App.css';
function App() {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
useEffect(() => {
fetch('/api/data')
.then(response => response.text())
.then(data => {
setData(data);
setLoading(false);
});
}, []);
return (
<div>
{loading ? <div className="spinner"></div> : <h1>{data}</h1>}
</div>
);
}
export default App;
Commentary
In this React component, when the component mounts:
- It fetches data from the Java backend.
- A spinner is displayed until the data is loaded.
- Once loaded, the data is rendered, making for a fluid user experience.
Using libraries like Framer Motion or React Spring alongside your React application can enhance animations, facilitating more complex interactions.
Final Thoughts
Integrating animations in your web UI using Java is not only feasible but also enhances user experience significantly. From subtle fade-ins to complex loading spinners, Java provides the backend architecture while CSS and JavaScript handle frontend manipulations efficiently.
As we continue to strive for intuitive web applications, understanding these integrations will elevate your development projects. For additional insights into animation design principles, revisit Mastering Smooth Sliding Animations in Web Design and improve your web UI further!
Feel free to leave comments or ask questions below – let’s create beautifully engaging web experiences together!
Checkout our other articles