Struggling with Runtime Config? Convert to JSON Effortlessly!
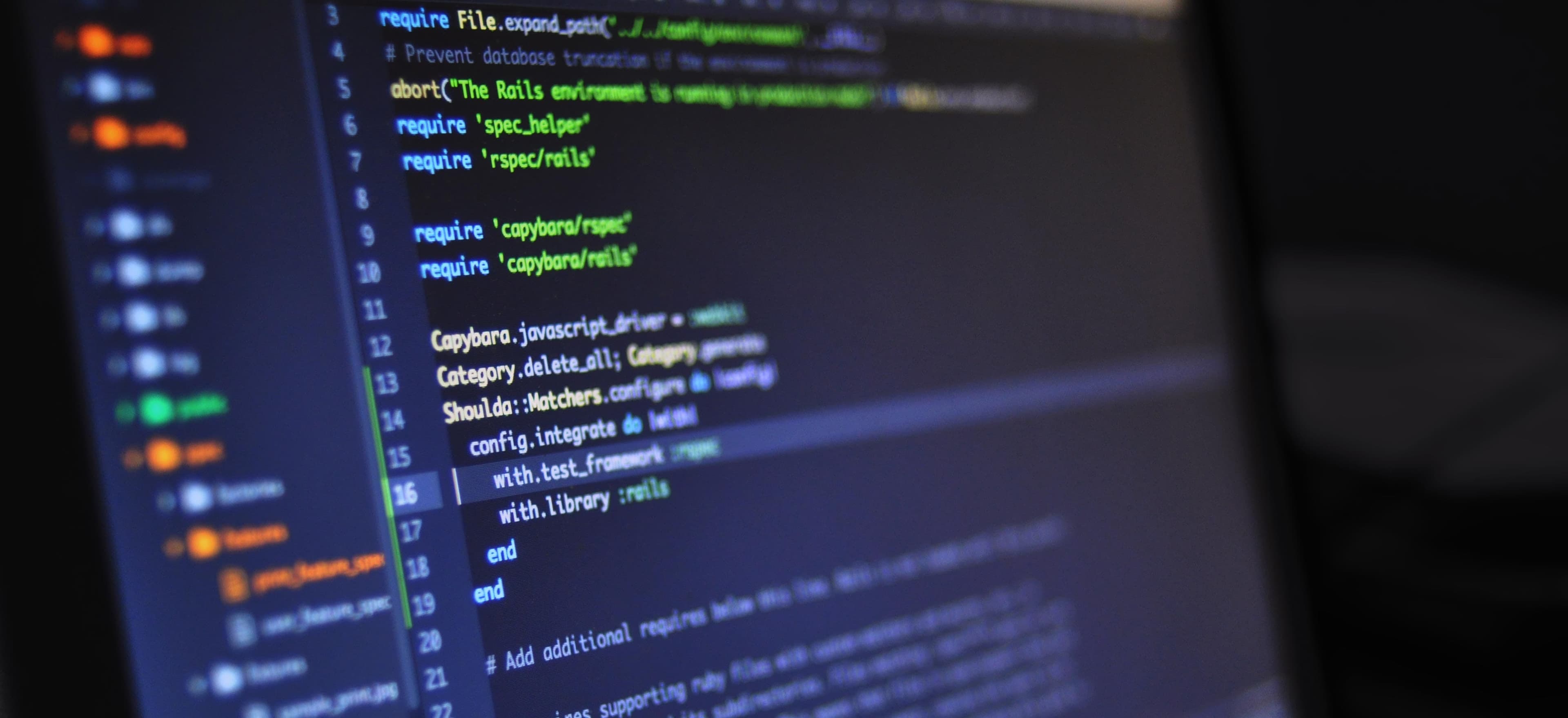
- Published on
Struggling with Runtime Config? Convert to JSON Effortlessly!
In today's rapidly evolving tech landscape, developers continuously seek efficient ways to manage configuration settings for their applications. One of the emerging tools that stands out in handling configuration parameters is Runtime Config. Despite its advantages, some developers find it challenging to implement effectively. This post will help you convert Runtime Config into JSON effortlessly, boosting your development efficiency.
Why Use Runtime Config?
Runtime Config allows dynamic configurations in applications. By managing configurations at runtime, you can avoid the need for redeploying applications whenever a change is needed in settings such as API keys, database connections, or feature toggles. Some key benefits include:
- Flexibility: Change configurations without intervention on the code level.
- Centralized Management: Manage configurations across multiple applications from a single location.
- Version Control: Easy to rollback changes in configurations.
However, configuring settings in a structured format can be complex. That's where JSON (JavaScript Object Notation) comes in. JSON is lightweight and easy for both humans and machines to parse and generate, making it an ideal format for configurations.
Converting Runtime Config to JSON
The conversion process from Runtime Config to JSON doesn't need to be daunting. Let's break it down into small, manageable steps.
Step 1: Collect Runtime Configuration
Collect your current configuration details. This might be in a key-value pair format. For instance:
APP_NAME=MyApp
API_KEY=12345
DB_HOST=localhost
DB_PORT=5432
Step 2: Define the JSON Structure
Once you have your parameters, the next step is to create a structured format for your JSON. An ideal JSON structure for the example above would look like this:
{
"app_name": "MyApp",
"api_key": "12345",
"database": {
"host": "localhost",
"port": 5432
}
}
Step 3: Use Java to Convert to JSON
To convert your collected configuration into JSON, we'll utilize the popular library Jackson. Jackson provides robust features for converting Java objects into JSON format and vice versa.
3.1. Add Jackson Dependency
If you are using Maven, you can add Jackson to your pom.xml
:
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.14.0</version>
</dependency>
3.2. Create a Configuration Class
Let's create a class that will represent our configuration:
import com.fasterxml.jackson.annotation.JsonProperty;
public class AppConfig {
@JsonProperty("app_name")
private String appName;
@JsonProperty("api_key")
private String apiKey;
private DatabaseConfiguration database;
public AppConfig(String appName, String apiKey, DatabaseConfiguration database) {
this.appName = appName;
this.apiKey = apiKey;
this.database = database;
}
}
class DatabaseConfiguration {
@JsonProperty("host")
private String host;
@JsonProperty("port")
private int port;
public DatabaseConfiguration(String host, int port) {
this.host = host;
this.port = port;
}
}
3.3. Convert the Object to JSON
Now you can easily convert an instance of AppConfig
to a JSON string. Here’s how:
import com.fasterxml.jackson.databind.ObjectMapper;
public class ConfigToJson {
public static void main(String[] args) {
try {
DatabaseConfiguration dbConfig = new DatabaseConfiguration("localhost", 5432);
AppConfig config = new AppConfig("MyApp", "12345", dbConfig);
ObjectMapper objectMapper = new ObjectMapper();
String jsonString = objectMapper.writeValueAsString(config);
System.out.println(jsonString);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Here, we instantiate the configuration classes and utilize the ObjectMapper
to convert them into a JSON string.
Why This Approach?
- Maintainability: By creating a class for configuration, you maintain a clear structure that’s easy to manage.
- Extensibility: Adding new parameters or changing existing ones becomes straightforward.
- Error Handling: Jackson handles errors internally, making it more robust.
Step 4: Integration with the Application
After converting Runtime Config to JSON, you will likely want to read it back into your application. You can effortlessly do this with Jackson as well:
String jsonInput = "{ \"app_name\": \"MyApp\", \"api_key\": \"12345\", \"database\": { \"host\": \"localhost\", \"port\": 5432 }}";
AppConfig newConfig = objectMapper.readValue(jsonInput, AppConfig.class);
Tips for Effective Configuration Management
- Environment-Specific Files: Maintain separate JSON configuration files for different environments (development, testing, production) to easily switch contexts.
- Version Control: Keep your configuration files under version control for traceability.
- Secure sensitive data: Ensure that sensitive information like API keys is encrypted or stored securely.
Lessons Learned
By utilizing JSON for your Runtime Config, you significantly improve the clarity and manageability of your application's settings. This method not only simplifies your development process but also enhances the performance and adaptability of your application.
For deeper insights into configuration management techniques and best practices, you can explore Spring Boot Configuration or check out this comprehensive guide on JSON handling with Jackson.
Recommended Tools
- Jackson Library: For JSON handling
- Spring Framework: For robust configuration management
- Git: For version control of configuration files
If you have any questions regarding the topic or need assistance with your implementation, feel free to comment below. Happy coding!
Checkout our other articles