Common Debugging Mistakes in Selenium Test Scripts
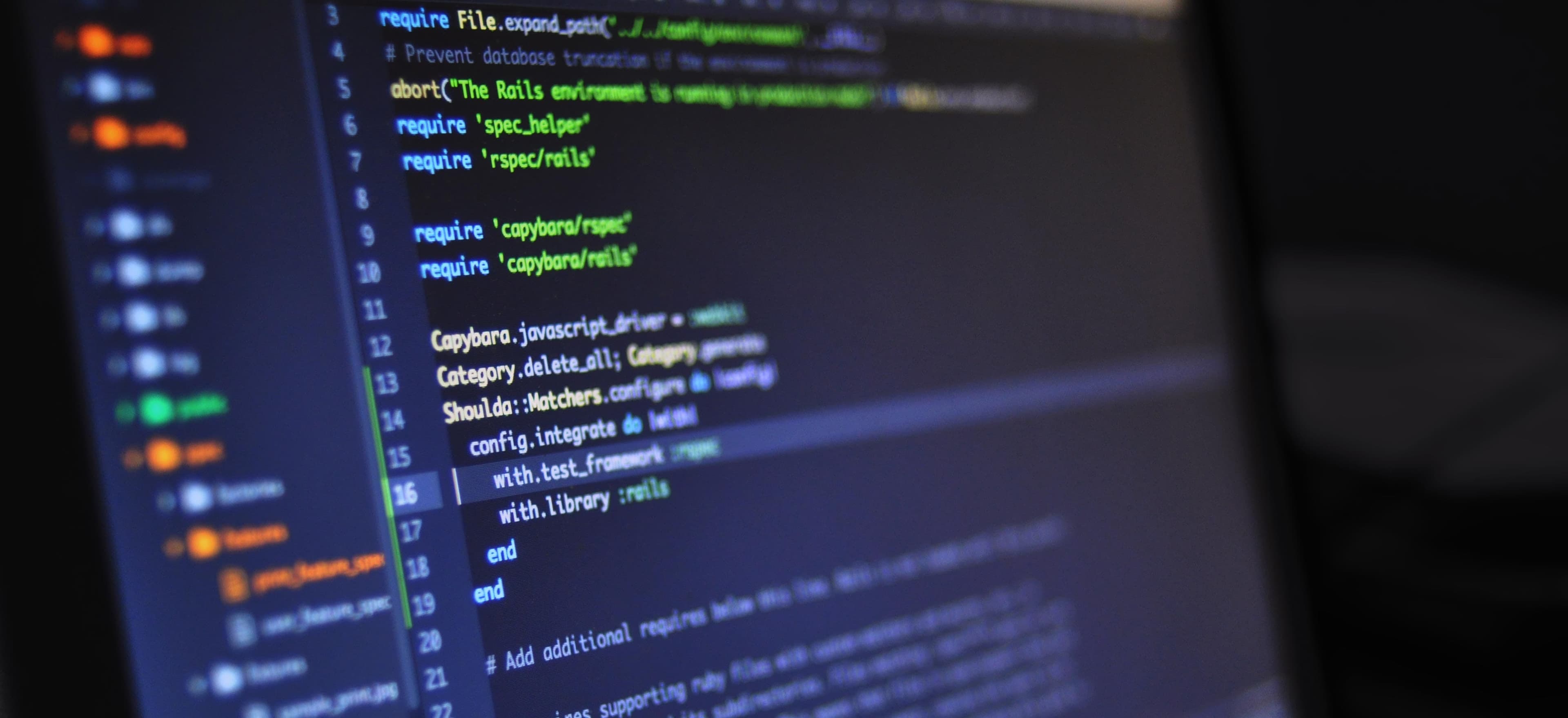
- Published on
Common Debugging Mistakes in Selenium Test Scripts
Debugging is a critical skill for any developer or QA engineer, especially when working with automated testing frameworks like Selenium. As you write and execute your test scripts, you may face a variety of challenges. This blog post will explore the most common debugging mistakes in Selenium test scripts and offer insight on how to avoid them.
Whether you are a beginner or an experienced user, understanding these pitfalls will help you develop more robust and reliable test scripts.
The Importance of Debugging in Selenium
Selenium is a powerful tool that automates browsers. However, like any software, it is susceptible to bugs and errors. Debugging is the process of identifying and correcting those errors. Getting it right is essential in ensuring that your tests perform as intended, providing confidence in your application's quality.
Debugging is not just about fixing issues; it’s about understanding what went wrong. By taking the time to debug effectively, you can improve your overall testing process and minimize frustration.
1. Ignoring Browser-Specific Issues
Mistake
One of the most common mistakes in Selenium debugging is ignoring browser-specific issues. Each browser has its quirks and behaviors, and a script that works perfectly in one browser may fail spectacularly in another.
Solution
To avoid this pitfall, you should:
- Test on multiple browsers. Make it a habit to test your scripts on all supported browsers regularly.
- Use browser developer tools. Familiarize yourself with browser-specific console messages and network issues.
// Example: Using WebDriverManager to manage different browsers
import io.github.bonigarcia.wdm.WebDriverManager;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class BrowserTest {
public static void main(String[] args) {
WebDriverManager.chromedriver().setup();
WebDriver driver = new ChromeDriver();
driver.get("http://example.com");
// Verify that the page title is correct
assert driver.getTitle().equals("Expected Title") : "Title mismatch!";
driver.quit();
}
}
In the code above, we are using WebDriverManager to set up the ChromeDriver. This minimizes configuration errors, ensuring that we're using the correct browser version.
2. Not Waiting for Page Loads and Element Visibility
Mistake
Forgetting to wait for page loads or element visibility is a classic mistake. If your script attempts to interact with an element before it's available, you'll face errors.
Solution
Implement explicit waits to wait for elements or conditions before interacting with them.
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class WaitExample {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("http://example.com");
// Using explicit wait
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("uniqueId")));
element.click(); // Click action after ensuring the element is visible
driver.quit();
}
}
In this example, the WebDriver waits up to 10 seconds until the element with the specified ID becomes visible. This simple modification prevents errors and allows your scripts to run reliably, regardless of load times.
3. Hardcoding Values
Mistake
Hardcoding values may seem convenient but can lead to maintenance nightmares. If your application's UI changes, you will likely be in for extensive fixes.
Solution
Utilize variables or configuration files for your test data. This allows easy modification without altering the script structure.
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class ConfigExample {
public static void main(String[] args) {
String URL = "http://example.com"; // Store URL in a variable
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get(URL); // Use variable instead of hardcoded URL
// Additional test steps...
driver.quit();
}
}
By storing the URL in a variable, we streamline any potential future updates. It simplifies the script and adheres to good development practices.
4. Using Implicit Waits Only
Mistake
Implicit waits are useful but can have unintended consequences. When mixed with explicit waits, they can cause confusion and lead to longer-than-necessary wait times.
Solution
Choose to use either explicit waits or implicit waits, but not both. This helps avoid complexities.
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.WebDriverWait;
import org.openqa.selenium.support.ui.ExpectedConditions;
public class ClickExample {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(5)); // Avoid using this with explicit waits
driver.get("http://example.com");
// Explicit wait
WebDriverWait wait = new WebDriverWait(driver, 10);
wait.until(ExpectedConditions.elementToBeClickable(By.id("buttonId"))).click(); // Ensure clickable before action
driver.quit();
}
}
In this code, we've shown how to implement implicit waits. Although it will work in certain scenarios, consider whether it might be more advantageous to rely solely on explicit waits.
5. Lack of Logging and Reporting
Mistake
Insufficient logging and reporting can make it challenging to analyze failures. Without clear messages in your logs, tracking down issues can be an arduous process.
Solution
Integrate logging frameworks into your tests to capture useful messages. Use allure or log4j for this purpose.
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class LoggingExample {
private static final Logger logger = LogManager.getLogger(LoggingExample.class);
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
logger.info("Setting up ChromeDriver.");
WebDriver driver = new ChromeDriver();
logger.info("Navigating to the website.");
driver.get("http://example.com");
// Further steps...
driver.quit();
logger.info("Test completed.");
}
}
In this example, we use log4j
for logging. Clear log statements help track each stage of the script execution, making it easier to diagnose issues when they arise.
My Closing Thoughts on the Matter
Debugging Selenium test scripts can be daunting, but by adhering to best practices and avoiding common mistakes, you can ensure more reliable and manageable code. Remember, the devil is in the details. Taking the time to understand and correct these mistakes not only improves your scripts but also enhances your overall testing strategy.
For further reading on Selenium best practices, consider exploring the official Selenium documentation or resources at Guru99's Selenium Tutorials. These links provide valuable insights and deeper learning about effective debugging approaches in Selenium.
Happy testing!
Checkout our other articles