Unlocking Insights: Troubleshooting Subversion Logs with Groovy
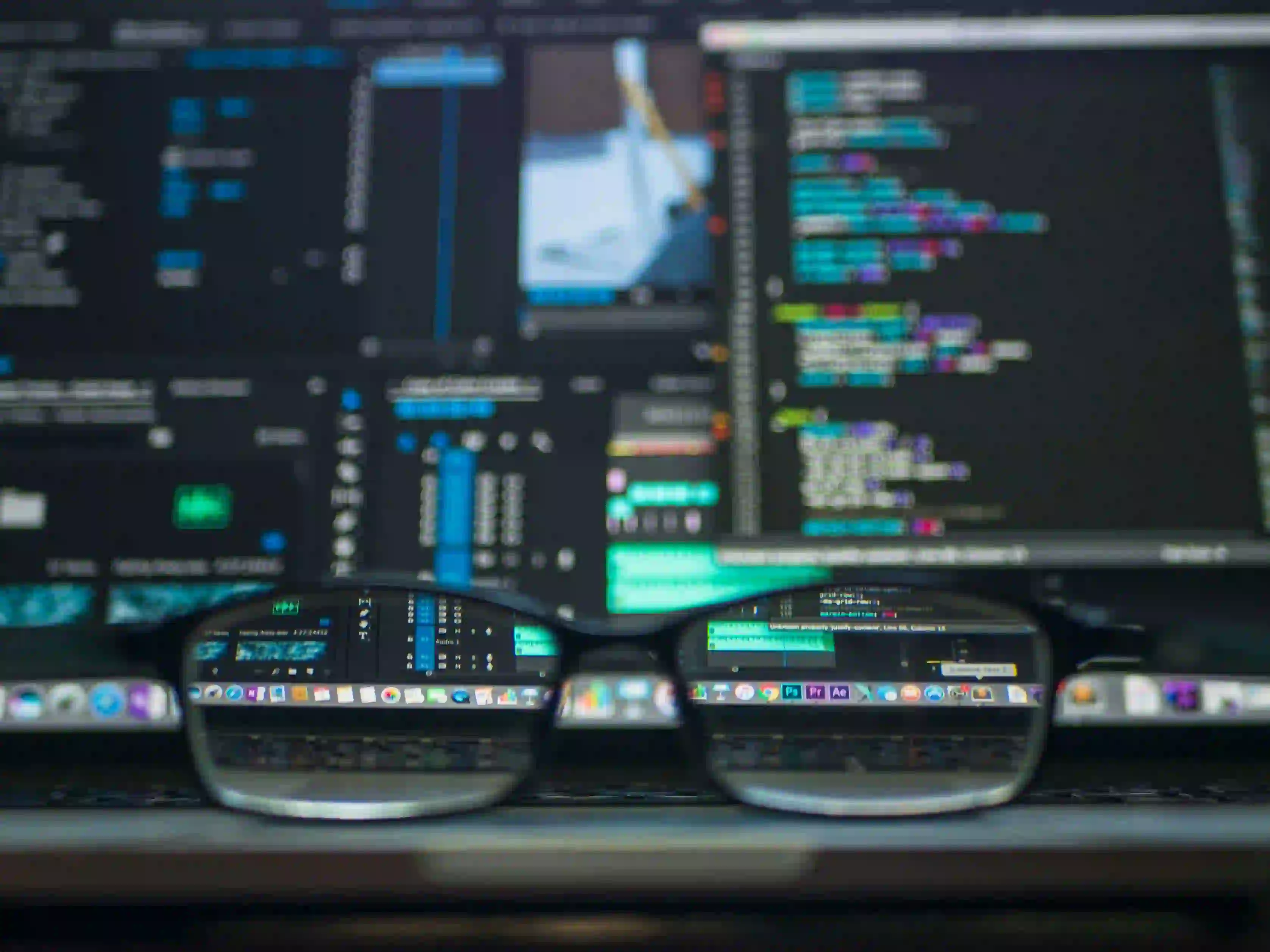
Unlocking Insights: Troubleshooting Subversion Logs with Groovy
Subversion (SVN) is a popular version control system that is widely used in software development. While it provides a robust framework for tracking changes, sometimes developers encounter issues when analyzing or troubleshooting logs. This is where Groovy steps in as a powerful scripting language that can simplify the process of parsing and analyzing SVN logs.
In this blog post, we will explore how to unlock valuable insights from SVN logs using Groovy, troubleshoot common issues, and provide you with practical examples to enhance your understanding.
Why Groovy for Subversion Logs?
Groovy is a dynamic language for the Java platform, known for its concise syntax, ease of integration with Java, and robust features. Its powerful collection handling and built-in support for regular expressions make it an ideal choice for processing text data like SVN logs.
Key Benefits of Using Groovy:
- Simplicity: Groovy's syntax is more concise than Java, which allows for quicker scripting and fewer lines of code.
- Integration: Being a part of the Java ecosystem, it can easily leverage existing Java libraries.
- Flexibility: Supports dynamic typing and functional programming paradigms, making it more adaptable for various tasks.
Now, let’s get started by exploring how to retrieve SVN logs and the basic structure of an SVN log entry.
Understanding SVN Log Structure
SVN logs are typically formatted as follows:
------------------------------------------------------------------------
r[revision number] | [author] | [date]
[commit message]
An example SVN log might look like this:
------------------------------------------------------------------------
r53 | johndoe | 2023-10-01 10:00:00 -0400 (Mon, 01 Oct 2023) | 1 line
Fixed a bug in the login module
Here, you can see key components such as the revision number, author, date, and the commit message.
Fetching SVN Logs
Before we can manipulate SVN logs, we need to fetch them. Groovy offers an easy way to execute shell commands using execute()
. Here’s how you can retrieve the logs from an SVN repository:
def getSvnLogs(String repoPath) {
def command = "svn log ${repoPath} --xml"
def process = command.execute()
process.waitFor()
return process.text
}
// Example usage
def logs = getSvnLogs('/path/to/your/repo')
println logs
In this snippet, we’re using svn log --xml
to fetch the logs in XML format, which makes it easier to parse programmatically.
Parsing SVN Logs with Groovy
Once we have the logs, we can parse them to extract meaningful insights. In the next snippet, we'll be using Groovy’s built-in XML handling to work with the logs.
Example: Parsing XML SVN Logs
Here’s how you can parse the fetched logs:
import groovy.xml.XmlSlurper
def parseSvnLogs(String xmlContent) {
def xml = new XmlSlurper().parseText(xmlContent)
xml.log.entry.each { entry ->
println "Revision: ${entry.@revision}"
println "Author: ${entry.author.text()}"
println "Date: ${entry.date.text()}"
println "Message: ${entry.msg.text()}"
println "------------"
}
}
// Call the function with the previously fetched logs
parseSvnLogs(logs)
Explanation:
XmlSlurper
: A Groovy class used for parsing XML content.- Iterate entries: The code loops through each log entry and outputs the revision, author, date, and commit message.
Parsing SVN logs allows developers to quickly identify key changes and authorship, which is vital when troubleshooting or tracking progress during development.
Common Troubleshooting Scenarios
Here are some common issues you might face when dealing with SVN:
1. Unexpected Commit Messages
Sometimes developers forget to add meaningful commit messages. We can write a Groovy script to find commits that lack messages.
def findEmptyCommitMessages(String xmlContent) {
def xml = new XmlSlurper().parseText(xmlContent)
def emptyCommits = xml.log.entry.findAll { entry ->
entry.msg.text().trim().isEmpty()
}
println "Commits without messages: ${emptyCommits.size()}"
emptyCommits.each { entry ->
println "Revision: ${entry.@revision} by ${entry.author.text()}"
}
}
// Checking for empty commit messages
findEmptyCommitMessages(logs)
2. Tracking Changes by Author
If you want to see the number of commits made by a specific author, you could expand on your parsing logic:
def countCommitsByAuthor(String xmlContent, String author) {
def xml = new XmlSlurper().parseText(xmlContent)
def commitCount = xml.log.entry.count { entry ->
entry.author.text() == author
}
println "${author} has made ${commitCount} commits."
}
// Checking commits made by a specific author
countCommitsByAuthor(logs, "johndoe")
3. Identifying Date Range Changes
In some scenarios, you might want to filter commits based on a specific date range. Here’s how to do that:
import java.text.SimpleDateFormat
def findCommitsInDateRange(String xmlContent, String startDateStr, String endDateStr) {
def startDate = new SimpleDateFormat("yyyy-MM-dd").parse(startDateStr)
def endDate = new SimpleDateFormat("yyyy-MM-dd").parse(endDateStr)
def xml = new XmlSlurper().parseText(xmlContent)
def commitsInRange = xml.log.entry.findAll { entry ->
def entryDate = Date.parse("yyyy-MM-dd'T'HH:mm:ss'Z'", entry.date.text())
entryDate >= startDate && entryDate <= endDate
}
println "Commits between ${startDateStr} and ${endDateStr}: ${commitsInRange.size()}"
commitsInRange.each { entry ->
println "Revision: ${entry.@revision} by ${entry.author.text()}"
}
}
// Check commits in a date range
findCommitsInDateRange(logs, "2023-10-01", "2023-10-31")
Explanation:
- Date parsing: The
SimpleDateFormat
is used to convert string dates intoDate
objects for comparison. - The script generates a list of commits that fall within the specified date range.
Final Considerations
In this article, we have demonstrated how to unlock insights from SVN logs using Groovy. By leveraging Groovy's features for XML processing, we can easily parse, analyze, and troubleshoot SVN logs.
Key Takeaways:
- Use Groovy for quick scripting and simplified log parsing.
- The XML format of SVN logs enables easy data manipulation.
- Common troubleshooting scenarios can be effectively addressed with Groovy scripts.
For more information on Groovy, check out the official Groovy Documentation.
Feel free to explore further and enhance your SVN troubleshooting capabilities with the power of Groovy. Happy coding!