Overcoming Latency Issues in Lambda Architecture Microservices
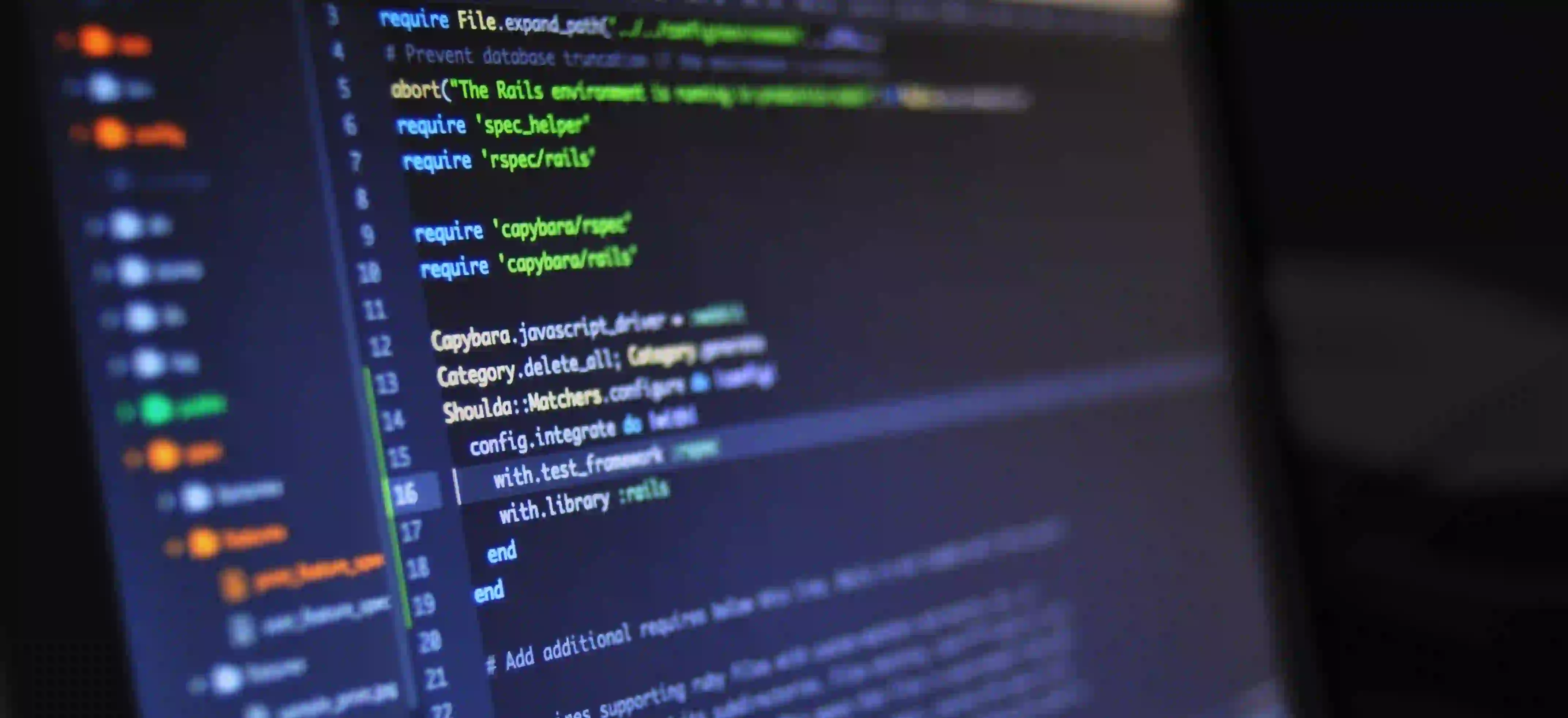
Overcoming Latency Issues in Lambda Architecture Microservices
In today's digital ecosystem, microservices have become the de facto standard for developing highly scalable and maintainable applications. However, when combined with the Lambda Architecture, achieving minimal latency can present unique challenges. In this blog post, we will explore how to tackle latency issues in Lambda Architecture microservices with a focus on Java programming.
Understanding Lambda Architecture
Before diving into latency solutions, letβs clarify what Lambda Architecture entails. Lambda Architecture aims to provide a robust framework for processing large volumes of data in real-time and batch processing. It primarily consists of three layers:
- Batch Layer: Manages the master dataset and computes batch views. This layer periodically processes large amounts of data.
- Speed Layer: Handles real-time data processing to provide low-latency views. It compensates for the delays in the batch layer.
- Serving Layer: Merges the batch and real-time views to provide a unified interface for data access.
The complexity of having multiple layers can introduce latency, especially when microservices call one another across these layers.
Common Sources of Latency in Lambda Architecture
Understanding the sources of latency is the first step in mitigation. Here are some common culprits:
- Network Latency: Occurs when microservices communicate over a network. This can be minimized but never entirely eliminated.
- Database Constraints: Slow queries or inefficient database structures can greatly impede response times.
- Cold Starts: Instances of microservices that haven't been recently accessed may take time to "warm up."
- Data Serialization/Deserialization: Converting data into a format suitable for network transmission can be resource-intensive.
Strategies for Reducing Latency in Lambda Architecture
Below, we present some effective strategies developers can use to reduce latency in Lambda Architecture microservices:
1. Optimize Network Calls
Use Asynchronous Communication
Synchronous calls can block the execution, leading to increased latency. By switching to asynchronous communication, you allow services to operate without waiting for responses.
import java.util.concurrent.CompletableFuture;
public class AsyncService {
public CompletableFuture<String> fetchData(String parameter) {
return CompletableFuture.supplyAsync(() -> {
// Simulate a network call
try {
Thread.sleep(200); // Simulated delay of 200ms
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
return "Data for " + parameter;
});
}
}
Commentary: By returning CompletableFuture
, we can perform other operations while awaiting a response, effectively reducing latency.
Load Balancing
Employ load balancers to distribute requests effectively across microservices. This optimizes resource usage and reduces the chances of individual instances becoming bottlenecks.
2. Efficient Data Management
Database Indexing
Improperly indexed databases can become a significant source of latency. Ensure that your database is appropriately indexed based on query patterns.
CREATE INDEX idx_user_email ON users(email);
Commentary: An index on the email field allows SQL queries filtering by email to execute much faster, consequently reducing response times.
Caching Strategies
Implement caching mechanisms to allow frequently accessed data to be served quickly without hitting the database for every request. You can use libraries like Ehcache or Hazelcast.
import org.ehcache.Cache;
import org.ehcache.CacheManager;
import org.ehcache.config.builders.CacheConfigurationBuilder;
import org.ehcache.config.builders.CacheManagerBuilder;
public class CacheExample {
public void setupCache() {
CacheManager cacheManager = CacheManagerBuilder.newCacheManagerBuilder()
.withCache("exampleCache",
CacheConfigurationBuilder.newCacheConfigurationBuilder(String.class, String.class)
.build()).build(true);
Cache<String, String> cache = cacheManager.getCache("exampleCache", String.class, String.class);
// Cache data
cache.put("key", "value");
}
}
Commentary: Caching can drastically reduce request times by eliminating the need to access the database for frequently fetched data.
3. Handling Cold Starts
Provisioning and Warm-Up Strategies
If using serverless architectures (e.g., AWS Lambda), cold starts can be a concern. Use provisioned concurrency for Lambda functions to keep them warm, or send periodic "ping" requests to keep instances active.
{
"Type": "AWS::Lambda::Alias",
"Properties": {
"FunctionName": "myFunction",
"FunctionVersion": "$LATEST",
"Name": "PROVISIONED_CONCURRENCY",
"ProvisionedConcurrencyConfig": {
"ProvisionedConcurrentExecutions": 2
}
}
}
Commentary: Provisioning concurrency can help eliminate latency caused by cold starts by keeping a certain number of instances initialized and ready to handle requests.
4. Data Serialization/Deserialization
Choose efficient serialization formats like Protocol Buffers or Avro, which are generally faster than JSON or XML due to their compact binary representation.
Example of Protocol Buffers
First, define your data structure:
syntax = "proto3";
message User {
string name = 1;
int32 age = 2;
}
Generate corresponding Java classes and use them like below:
import com.example.UserOuterClass.User;
public class ProtoBufExample {
public byte[] serialize() {
User user = User.newBuilder().setName("John").setAge(30).build();
return user.toByteArray(); // Efficient binary representation
}
public User deserialize(byte[] data) throws InvalidProtocolBufferException {
return User.parseFrom(data); // Fast deserialization
}
}
Commentary: Protocol Buffers are efficient in both size and speed, making them ideal for microservices communication, especially when dealing with large volumes of data.
Wrapping Up
Reducing latency in Lambda Architecture microservices requires a strategic approach that encompasses network optimization, efficient data management, handling cold starts, and seamless data serialization. By implementing the strategies outlined above, Java developers can build robust microservices that handle data effectively while minimizing latency.
For further reading on microservices and Lambda Architecture, you might find these resources helpful:
By thoughtfully applying these principles, you can ensure that your microservices operate with speed, efficiency, and reliability in a Lambda Architecture environment. Happy coding!