Challenges in Binding Non-Transactional Resources to JTA
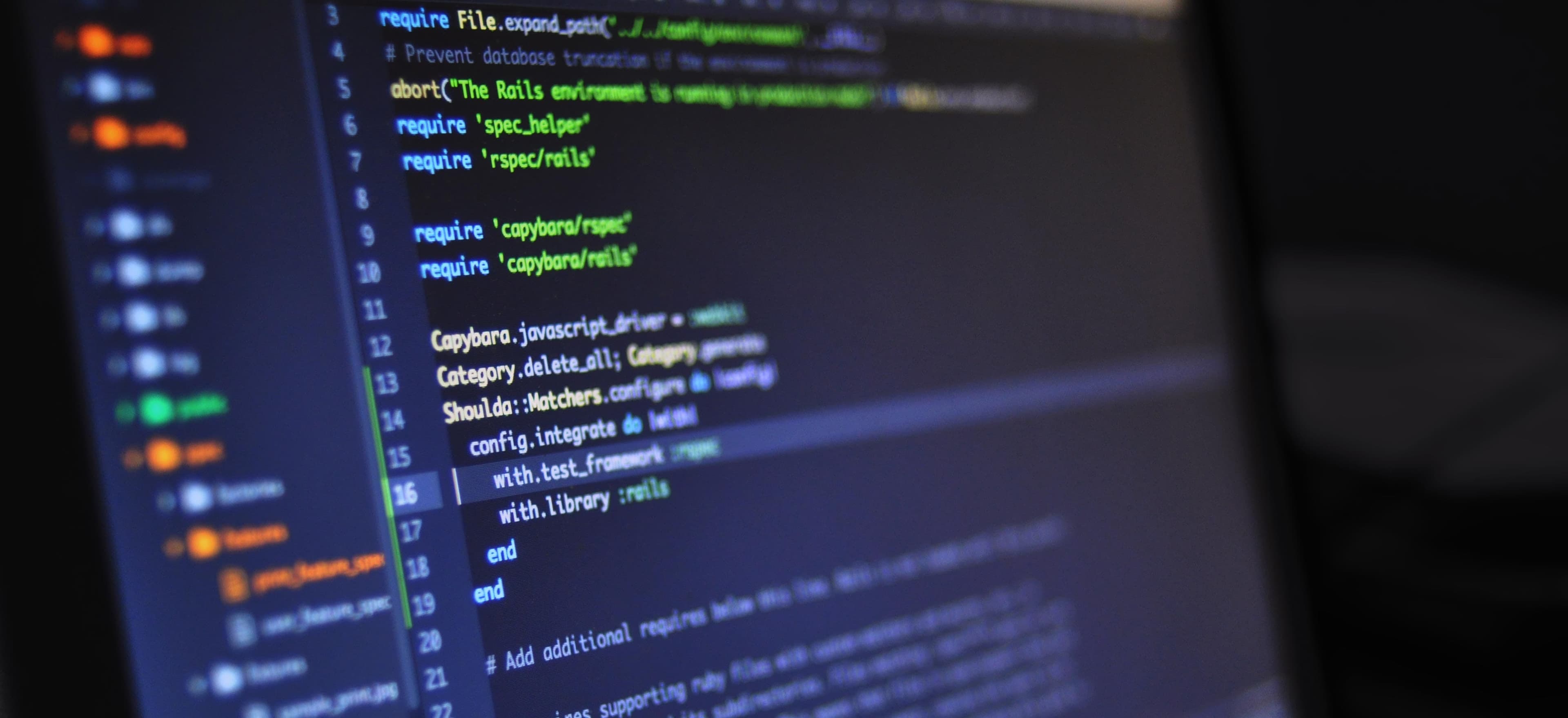
- Published on
Challenges in Binding Non-Transactional Resources to JTA
Java Transaction API (JTA) is a powerful tool for developers seeking to manage transactions across multiple resources such as databases, message queues, and other resources. However, binding non-transactional resources to JTA can present a unique set of challenges. In this blog post, we will explore these challenges, illustrate important code snippets, and discuss best practices for effectively managing non-transactional resources within a JTA context.
Understanding JTA
The Java Transaction API (JTA) is an important interface for managing transactions in Java applications. It allows for coordinate transactions across various transactional resources like databases and message queues. The real strength of JTA is in its ability to ensure the consistency and integrity of multiple interactions through a two-phase commit protocol.
However, when working with non-transactional resources—those not natively designed to participate in JTA transactions like certain APIs and file systems—additional complexities arise.
Types of Non-Transactional Resources
Understanding non-transactional resources is crucial when considering their use in conjunction with JTA. Here are some examples:
- External APIs: Services that do not provide native transactional guarantees.
- File Systems: Any local file operations that lack transactional support.
- Third-Party Libraries: Components that perform operations without integrating transactional support.
These resources, while useful, can lead to inconsistencies when they're involved in operations that require atomicity, consistency, isolation, and durability (ACID)—the four pillars of transaction integrity.
Common Challenges
1. Atomicity
Atomicity ensures that a transaction is all-or-nothing. When a transaction includes both JTA and non-JTA resources, achieving atomicity becomes problematic. If an operation on the non-transactional resource fails but the JTA transaction commits, the entire operation's effects can become inconsistent.
Example Illustration
Consider the following Java code where a JTA transaction and a REST API call are made consecutively:
import javax.transaction.UserTransaction;
import javax.naming.InitialContext;
UserTransaction utx = (UserTransaction) new InitialContext().lookup("java:comp/UserTransaction");
utx.begin();
try {
// Perform the transactional operation
orderService.createOrder(order);
// Call an external API
InventoryService.updateInventory(productId, newQuantity);
utx.commit();
} catch (Exception e) {
utx.rollback();
// Handle failure
}
Why It's Problematic: If the call to InventoryService.updateInventory
fails after the order creation has succeeded, the customer would have an order with no corresponding inventory adjustment, breaking atomicity.
2. Isolation Levels
Different sources of resources may operate under different isolation levels. JTA provides a way to define isolation levels for transactional operations, but non-transactional resources lack such guarantees. This can lead to race conditions and dirty reads.
Code Snippet for Isolation Example
import javax.transaction.Transaction;
Transaction transaction = utx.getTransaction();
transaction.setTransactionTimeout(300); // ensure timeout for JTA
Why It's Important: Managing isolation in non-transactional resources could involve implementing your logic, which increases complexity.
3. Error Handling
When a JTA transaction encounters issues, robust error handling is necessary. However, if an error arises in a non-transactional operation, tracking and responding to these issues can be challenging.
Effective Approach to Error Handling
try {
// JTA operations
} catch (Exception e) {
// Rollback if necessary
utx.rollback();
// Log the failure for external API
logError(e);
// Additional recovery strategy can be implemented
}
Why It's Necessary: Properly managing errors allows a clean recovery strategy to prevent potential data inconsistency.
4. Compensating Transactions
An alternative to rolling back transactions in certain scenarios is implementing compensating transactions on non-JTA resources. This approach is fundamental when the initial operation can't be undone, such as with external APIs.
Compensating Transaction Example
Suppose you create an order and want to cancel it later. You can create a method to handle the undo operation:
public void cancelOrder(String orderId) {
try {
inventoryService.restockOrder(orderId);
// Mark order as cancelled in order service
} catch (Exception e) {
// Handle failure
}
}
Why Use Compensating Transactions: They provide alternatives to rollback, allowing you to maintain consistency across your business operations.
5. Performance Issues
The overhead of handling transactions across different systems can impact performance. Long-running transactions involving non-transactional resources can increase the chances of contention and slow down system response times.
6. Testing Complexity
Incorporating non-transactional resources into JTA transaction tests complicates unit tests and integration tests. You often need to mock APIs or ensure the state of external services doesn’t affect the outcome.
Best Practices for Managing Non-Transactional Resources
1. Minimize Scope
Limit the use of non-transactional resources within transactional boundaries. The fewer interactions, the lower the risk of inconsistency.
2. Use Reliable Messaging Patterns
Incorporate asynchronous patterns such as message queues to ensure eventual consistency. This can help bridge the gap between transactional and non-transactional components.
3. Implement Monitoring
Employ an observability strategy to watch for failures and data inconsistencies. Log all transaction states diligently.
4. Adopt Circuit Breaker Patterns
These patterns avoid cascading failures. If a non-transactional service fails, the circuit breaker will allow the system to recover gracefully.
5. Compensating Actions
Establish clear compensating actions for critical non-transactional operations, ensuring the ability to revert to a known good state if necessary.
Final Thoughts
Binding non-transactional resources to JTA is fraught with challenges that can compromise application integrity. The complexity it introduces necessitates measured approaches and best practices, ensuring that operations remain reliable, consistent, and efficient.
As you architect your applications, take the time to understand the intricacies of JTA and non-transactional resources. For additional insights regarding Java Transaction API, consider reading Oracle’s Official JTA Documentation or Java EE Transactions.
By synthesizing these discussions, developers can navigate the complexities of binding non-transactional resources to JTA while ensuring the integrity and reliability of their applications. Happy coding!
Checkout our other articles