Common Pitfalls in Dynamic Property Management with Spring
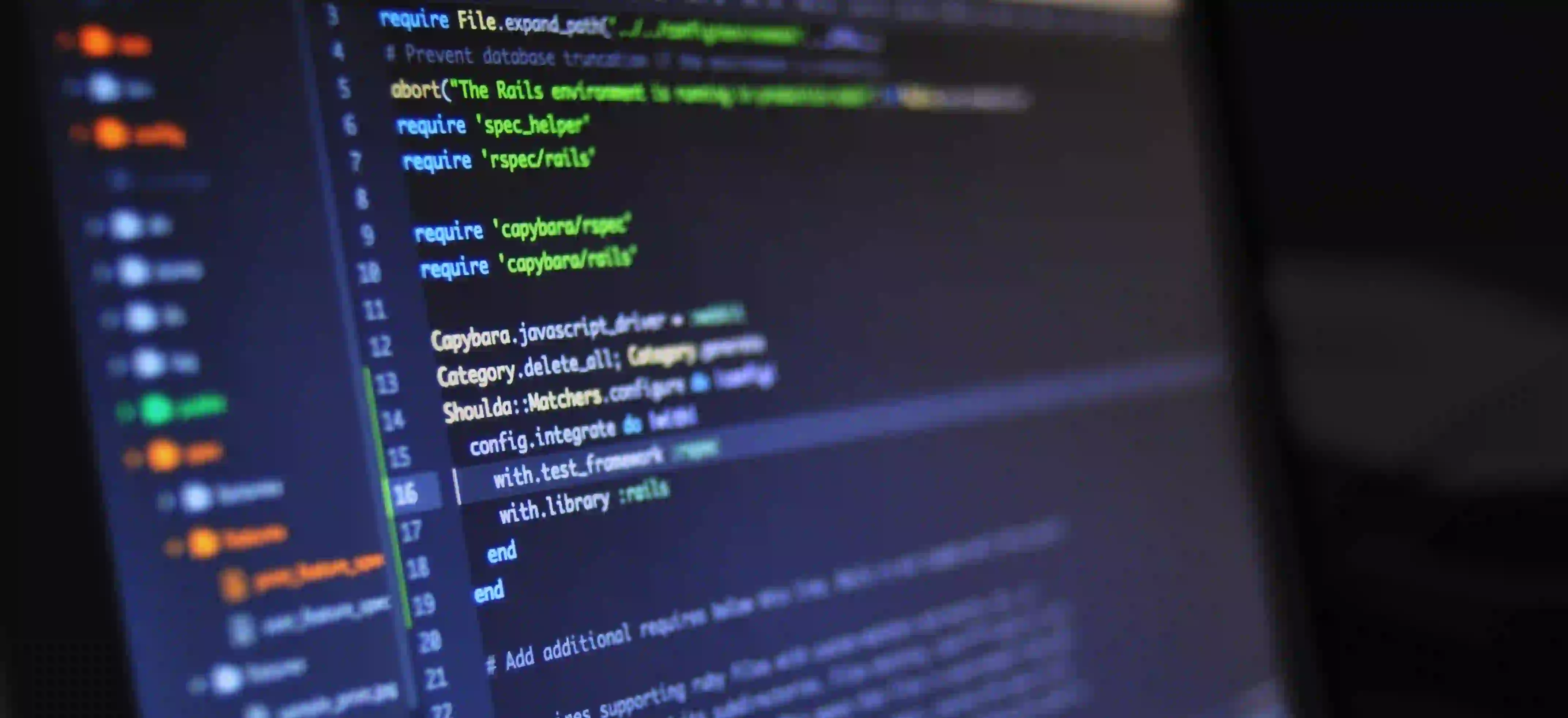
Common Pitfalls in Dynamic Property Management with Spring
Dynamic property management in Spring is a powerful feature that allows developers to externalize configuration and manage application properties flexibly. However, navigating the intricacies of this feature can lead to some common pitfalls that may hinder application performance or introduce bugs. In this blog post, we will delve into these pitfalls, provide sample code, and offer solutions to help you leverage Spring’s dynamic property management effectively.
Understanding Dynamic Property Management in Spring
Before diving into the pitfalls, it’s essential to understand what dynamic property management entails. In Spring, properties can be managed dynamically through various mechanisms such as:
- Environment abstraction
- Property sources
- Configuration properties
Spring's environment abstraction helps separate configuration from code, allowing you to create more flexible and maintainable applications. However, misusing these features can lead to complications.
Pitfall #1: Overusing Environment Variables
One of the most common pitfalls is over-reliance on environment variables. While they offer a straightforward way to externalize properties, mismanagement can lead to confusing issues.
Example
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
@Component
public class MyService {
@Value("${my.property}")
private String myProperty;
public void performAction() {
System.out.println("Property Value: " + myProperty);
}
}
Why This Matters
Using @Value
directly on a property can lead to issues where properties are not found, especially if the environment configuration changes or if the application is run in various environments (development vs. production). It’s crucial to manage these properties consistently across all environments.
Solution
Use a configuration file or class that consolidates all property values. This approach allows you to validate and transform properties centrally.
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.stereotype.Component;
@Component
@ConfigurationProperties(prefix = "my")
public class MyProperties {
private String property;
public String getProperty() {
return property;
}
public void setProperty(String property) {
this.property = property;
}
}
By adopting this method, you ensure that properties are loaded and handled in a more predictable manner.
Pitfall #2: Ignoring Default Values
Many developers forget to provide default values for properties. In Spring, if a property is missing, it may cause the application to fail or behave unpredictably.
Example
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
@Component
public class NotificationService {
@Value("${notification.timeout}")
private int timeout;
public void sendNotification() {
// Implementation
}
}
Why This Matters
If notification.timeout
is not set in the properties file or environment, it will cause our service to fail at runtime. This is not just inconvenient but also can lead to a poor user experience.
Solution
You can specify default values in your @Value
annotation:
@Value("${notification.timeout:5000}")
private int timeout; // Default value of 5000 milliseconds
With this approach, your application will fallback to the default value if no property is found, enhancing overall stability.
Pitfall #3: Not Refreshing Property Values Dynamically
Another significant issue arises from static property values. Many applications require the dynamic reloading of properties to reflect changes in real-time without restarting the application.
Example
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
@Component
public class DynamicService {
@Value("${some.dynamic.property}")
private String dynamicProperty;
public void displayProperty() {
System.out.println("Current Dynamic Property Value: " + dynamicProperty);
}
}
Why This Matters
If some.dynamic.property
is updated in the configuration file, the running instance will not reflect this change unless restarted. This can be problematic, especially in cloud-native applications where up-time is critical.
Solution
Use Spring Cloud Config or Spring Boot's support for reloading properties. For example, adding the following to your application.yml makes it possible to refresh properties.
spring:
cloud:
config:
uri: http://your-config-server
You can then leverage the /refresh
endpoint in your application to reload properties without a restart. This way, properties can be managed dynamically, providing great flexibility.
Pitfall #4: Configuration Propagation
In larger applications, it's easy to create complex interdependencies between various property sources. Changes in one area could lead to cascading failures or configuration crises in others.
Example
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Configuration;
@Configuration
public class AppConfig {
@Value("${db.url}")
private String dbUrl;
@Value("${service.url:${db.url}}") // This connects service URL to dbUrl
private String serviceUrl;
}
Why This Matters
While conditional dependencies may seem beneficial, they could lead to tight coupling, where changing one property may involve significant downstream implications.
Solution
Keep configuration at one level and avoid indirect referencing when possible. Consolidate related properties to keep them grouped, and document their intended relationships more clearly.
The Bottom Line
Dynamic property management in Spring is a powerful feature that, when used correctly, can significantly enhance your application's flexibility and maintainability. However, awareness of the common pitfalls is crucial for mitigating potential risks. By applying best practices such as using configuration classes, specifying default values, reloading properties dynamically, and observing dependencies, you can navigate the intricacies of Spring’s property management effectively.
For more detailed insights into Spring property management, check out the Spring Boot Documentation and Spring Cloud Config. Always be ready to refactor and enhance your property management strategies as your application evolves!
In this blog post, we have explored several pitfalls associated with dynamic property management in Spring and provided solutions to overcome them. Stay curious and keep experimenting!