Common Docker Misconfigurations in Java EE Apps
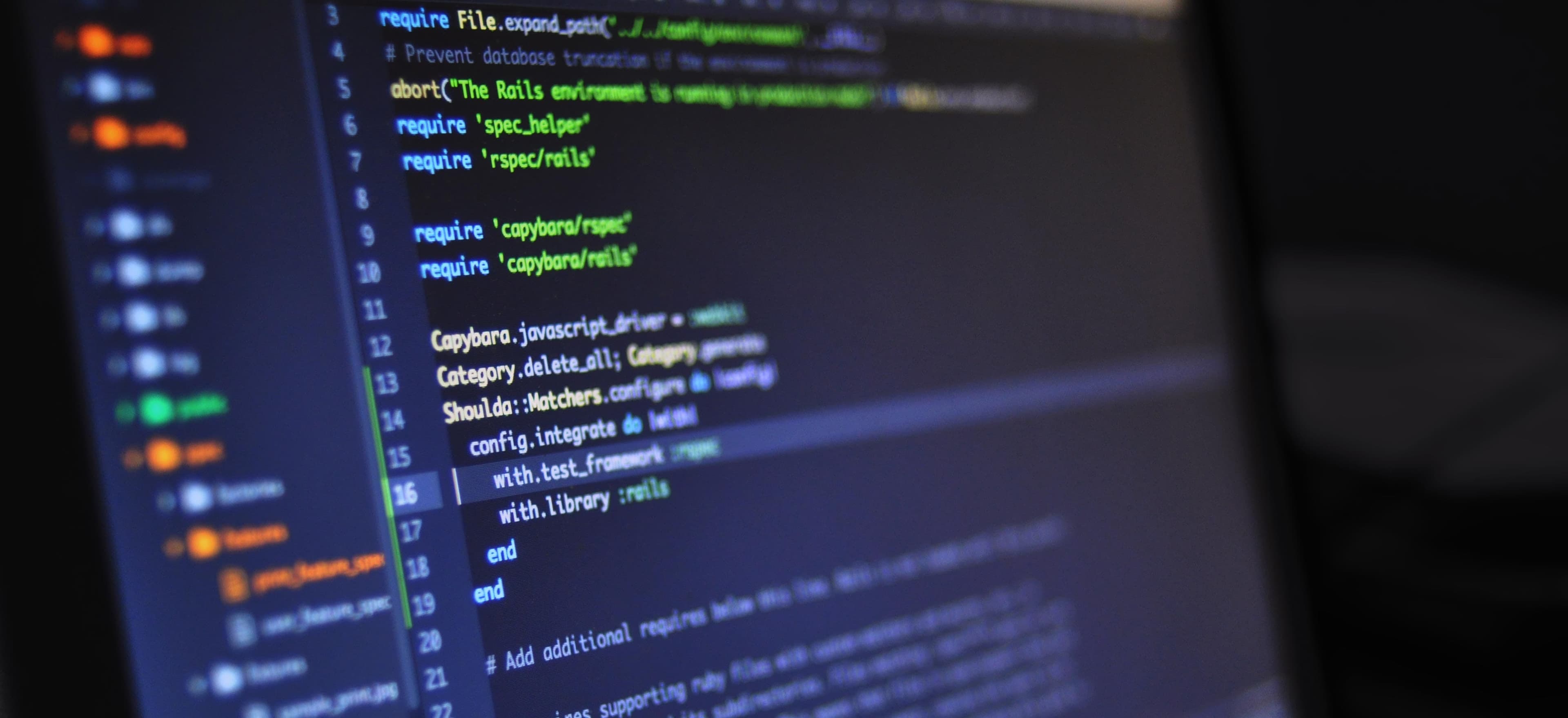
- Published on
Common Docker Misconfigurations in Java EE Apps
Containerization has revolutionized the way we deploy applications. For Java EE developers, deploying their applications using Docker can lead to increased efficiency and scalability. However, common misconfigurations can hinder performance and security. This post will explore some typical Docker misconfigurations in Java EE applications, how to identify them, and best practices to avoid these issues.
Understanding Docker and Java EE
Before diving into misconfigurations, it's essential to understand the two technologies at play here. Docker is a platform for developing, shipping, and running applications in containers. Java EE, now Jakarta EE, is an enterprise-level framework that allows developers to build scalable, reliable, and secure applications.
Combining these technologies can enhance your application's portability, but it also brings its own set of challenges. Docker images should be optimized to ensure smooth deployment and adherence to best practices.
Common Docker Misconfigurations
1. Exposing Unnecessary Ports
One of the first misconfigurations developers encounter is exposing unnecessary ports. By default, Docker containers expose all ports defined in the application. If your Java EE application uses various microservices, it may expose multiple ports.
What to do: Always specify only the ports necessary for your application. For example, if your application runs on port 8080, update your Dockerfile
like this:
EXPOSE 8080
This will ensure that no sensitive ports are unintentionally exposed to the outside world, reducing the attack surface area.
2. Using the Latest Base Image
Using the latest version of a Docker image might seem like a good practice. However, it can introduce breaking changes unexpectedly.
Why this is an issue: When you use a "latest" tag, your build might break if a new version introduces updates that are incompatible with your application. This unpredictability can lead to deployment challenges.
Best Practice: Always specify a specific version of the base image in your Dockerfile:
FROM openjdk:11-jre-slim
Doing this will ensure that your builds are consistent and predictable.
3. Not Following Layer Optimization
Docker layers are caching mechanisms that help speed up builds. However, if you do not optimize the structure of your Dockerfile, it can lead to larger image sizes and slower build times.
Example of potential misconfiguration:
COPY . /app
RUN mvn clean package
In this case, every time you change a source file, all layers following it will be rebuilt.
Optimization Tip: Separate the dependencies from the application code. Use multi-stage builds in your Dockerfile:
# Stage 1: Build the application
FROM maven:3.6.3-jdk-11 AS build
COPY pom.xml /app/
COPY src /app/src
WORKDIR /app
RUN mvn clean package -DskipTests
# Stage 2: Run the application
FROM openjdk:11-jre-slim
COPY --from=build /app/target/myapp.jar /app/myapp.jar
ENTRYPOINT ["java", "-jar", "/app/myapp.jar"]
This way, layer invalidation is minimized, thus improving efficiency when you change your application code but not your dependencies.
4. Ignoring Healthchecks
Health checks are critical for managing Docker containers. Ignoring them may lead to faulty containers running in production without detection.
By default, Docker does not check whether your application is running properly. If your Java EE application crashes or enters an unhealthy state, you need a mechanism to identify this.
Implement a Health Check: Add a healthcheck to your Dockerfile:
HEALTHCHECK CMD curl --fail http://localhost:8080/health || exit 1
This command will periodically ping your application and ensure it is running as expected, allowing you to automatically restart unhealthy containers.
5. Container Size Considerations
Large container images can slow down processing, deployment, and scaling. It's crucial to monitor the size of the images you are using.
Decode the files in your image. You'll want to keep it minimal.
Strategies for reducing size:
- Use multi-stage builds as previously mentioned.
- Remove unnecessary tools and packages.
- Use a slim base image.
Dockerfile Example:
FROM openjdk:11-jre-slim
WORKDIR /app
COPY myapp.jar .
ENTRYPOINT ["java", "-jar", "myapp.jar"]
This snippet illustrates using a base image that is already reduced, preventing unnecessary bloat.
6. Not Managing Environment Variables
Environment variables are perfect for configuration in Java EE applications. Mismanaging them can lead to hard-coded values in your application or sensitive data exposure.
Best practices for environment management:
- Use
.env
files to store configurations. - Refer to these environment variables in your application startup.
Here's how to set environment variables in your docker-compose.yml
:
version: '3'
services:
myapp:
image: myapp:latest
env_file:
- .env
Now you can easily manage environment variables without hardcoding them into your application.
Closing the Chapter
Dockerizing your Java EE application offers many benefits, but misconfigurations can severely impact performance and security. From exposing unnecessary ports to mismanaging environment variables, these pitfalls can be avoided with best practices.
Implement strategies like specifying versions in your Docker images, optimizing layers, and setting up health checks to fortify your Docker setup. By adhering to these guidelines, you can ensure a smoother deployment process and better overall performance of your Java EE applications running in Docker containers.
For more insights on Docker best practices, check out the official Docker documentation. For Java EE development guidance, refer to the Jakarta EE documentation.
Happy coding!
Checkout our other articles