Troubleshooting Buildpack Issues for Java Container Builds
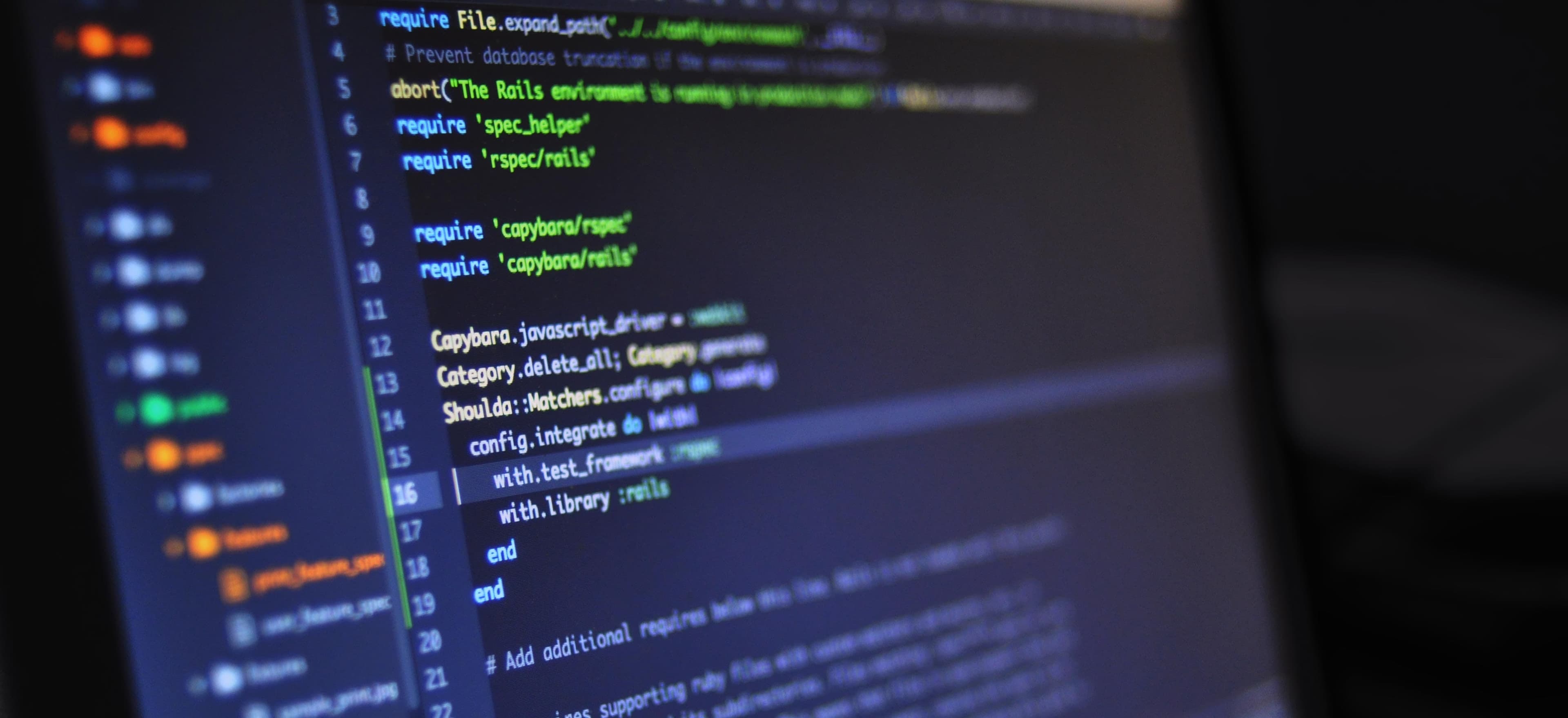
- Published on
Troubleshooting Buildpack Issues for Java Container Builds
When working with Java applications and deploying them in containerized environments, buildpacks are an important aspect of the process. Buildpacks automate the setup of the runtime environment in which your application will run, handling tasks like dependency resolution, compilation, and configuration. However, issues can arise during the build phase, causing frustrating delays and confusion. In this blog post, we will explore common buildpack issues for Java container builds, along with practical troubleshooting steps and examples.
Understanding Buildpacks
Before diving into troubleshooting, it's beneficial to understand what buildpacks are and how they function. Buildpacks are typically used in platforms such as Cloud Foundry, Heroku, and Google Cloud Run. They transform your source code into container images by layering various components:
- Frameworks: Java has various frameworks like Spring Boot, Quarkus, etc., which might require specific library dependencies.
- JDK/JRE Settings: The required version of Java needed for your application.
- Configuration Files: Properties and settings that tailor the runtime environment.
For more detailed insights on how Java buildpacks work, you can refer to the Cloud Native Buildpacks documentation.
Common Issues with Java Buildpacks
1. Missing Dependencies
One of the most prevalent issues developers face is missing dependencies. This scenario often occurs when you have defined dependencies in your pom.xml
(Maven) or build.gradle
(Gradle) files but have not included them before starting the build process.
Troubleshooting Steps
- Check Dependency Listings: Ensure all necessary dependencies are explicitly defined in your build configuration files.
- Inspect Logs: Buildpacks provide detailed log outputs. Look for messages indicating missing dependencies.
Example
Here’s a snippet from a pom.xml
file defining common dependencies:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
<version>2.5.4</version>
</dependency>
</dependencies>
Make sure you have included all required dependencies.
2. Incorrect Java Version
Another common issue is specifying the wrong Java version in the buildpack
or configuration files. This leads to compatibility issues that may manifest as runtime errors.
Troubleshooting Steps
- Specify Java Version: Use the correct Java version in your
buildpack
settings. - Testing Different Versions: Validate your application with multiple Java versions if necessary.
Example
In your Dockerfile
, you can specify the Java version like so:
FROM adoptopenjdk:11-jre-hotspot
By specifying adoptopenjdk:11
, you ensure your Java version aligns with your application's requirements.
3. Environmental Variable Issues
Environmental variables often dictate behavior in build processes, so issues can arise from incorrectly set or missing variables. For example, API keys, database URLs, or application settings are often passed as environment variables.
Troubleshooting Steps
- Check Environment Settings: Ensure your environment variables are defined correctly before building the container.
- Debugging Outputs: Print environmental variables in your logs to confirm their values during the build phase.
Example
You can define environment variables in your Dockerfile using the ENV
command:
ENV SPRING_DATASOURCE_URL=jdbc:mysql://localhost:3306/mydb
4. Buildpack Version Mismatch
Often, the buildpack version itself can lead to numerous compatibility issues. Using an outdated or incompatible version can introduce bugs or prevent your application from compiling.
Troubleshooting Steps
- Regular Updates: Frequently update and check the changelogs for your buildpacks.
- Compatibility Review: Before upgrading, review your existing setup for potential compatibility pitfalls.
Example
Here’s how you might specify a buildpack version in a Cloud Foundry manifest:
buildpacks:
- java_buildpack => 4.48
Keeping your buildpack up to date can resolve several issues.
5. Out of Memory Errors
Your container may fail to build or run if it is allocated insufficient memory. Java applications are notorious for high memory usage, which can lead to failures during the build process.
Troubleshooting Steps
- Increase Memory Limits: Override default memory limits using the container orchestrator’s settings.
- Java Memory Settings: Adjust Java options to optimize memory management.
For example, in your Dockerfile
, you might specify:
CMD ["java", "-Xmx512m", "-jar", "app.jar"]
This limits the Java Heap Memory to 512 MB.
6. File Not Found Errors
During the build process, a common error is “file not found.” This can occur if the necessary files (e.g., configuration files, binaries) aren't included or reachable within the container.
Troubleshooting Steps
- File Position Verification: Confirm that all necessary files are in the expected locations in your project.
- Docker Ignore Files: Ensure that your
.dockerignore
file isn’t excluding files inadvertently.
Example
Your .dockerignore
file should look something like this:
target/*
!target/*.jar
This ensures jar files from the target
directory are included correctly in the container build.
Closing the Chapter
While troubleshooting buildpack issues in Java container builds can be frustrating, having a clear set of strategies at your disposal will ease the process. By systematically addressing common issues, such as dependency management, configuration settings, and environment variables, you can bring clarity and resolve potential pitfalls in your build workflow.
Additional Resources
By considering the tips and examples outlined in this blog post, you’ll be better equipped to tackle the challenges that arise while building Java applications in containers efficiently. Happy coding!