Debunking 5 Common Myths About Programming Skills
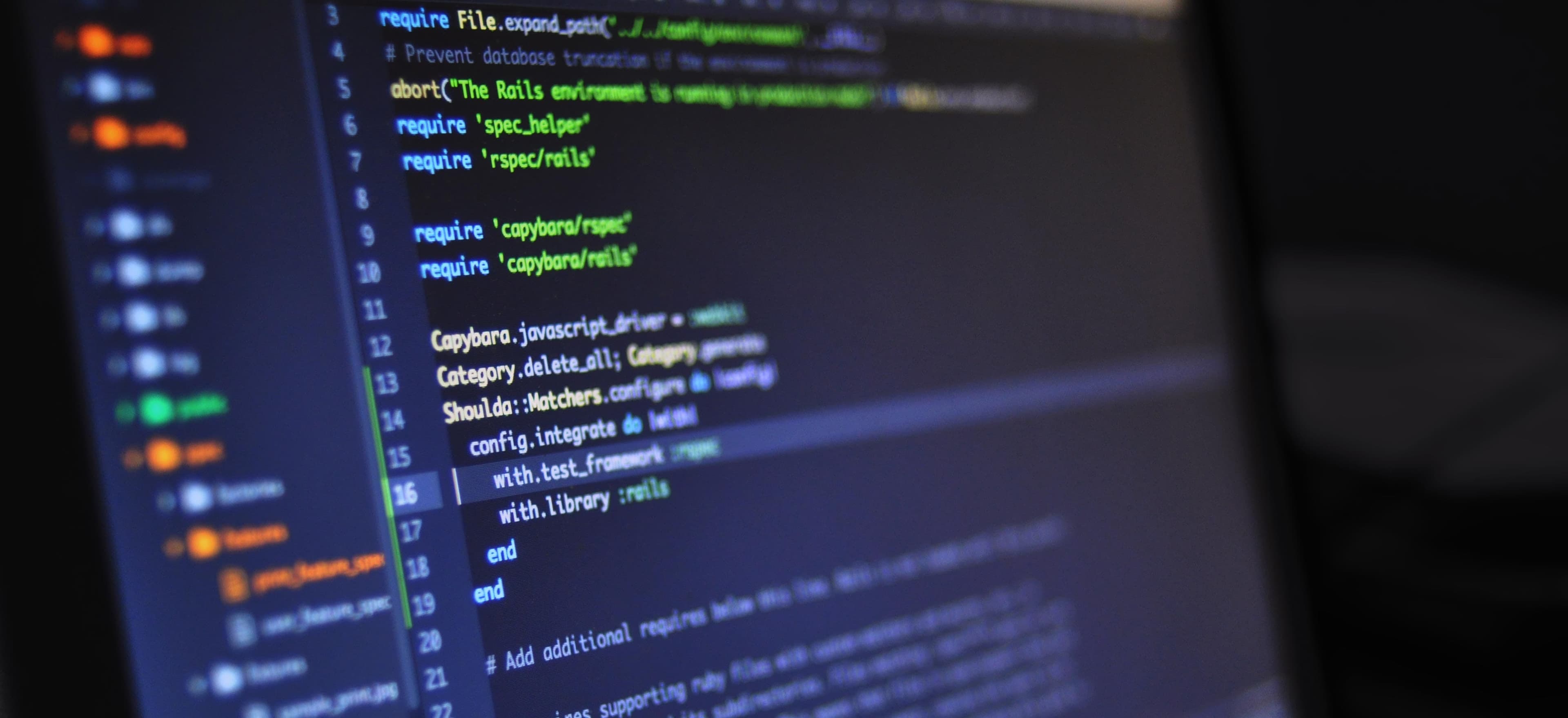
- Published on
Debunking 5 Common Myths About Programming Skills
Programming has become an essential skill in today’s technologically driven world. However, there are numerous myths surrounding the realm of programming that can perpetuate misconceptions, discourage newbies, and even hinder career advancement. In this article, we’ll debunk five common myths about programming skills, providing clarity and insights that will empower you as a budding programmer or a seasoned professional.
Myth 1: You Must Be a Math Genius to Code
The Truth
Many individuals believe that programming is solely a domain reserved for those with advanced mathematical proficiency. While understanding algorithms and logic is critical in programming, mathematics at an advanced level is not always a prerequisite. In fact, most programming tasks involve standard levels of mathematics like arithmetic or basic algebra.
Code Example
Consider a simple program that calculates the area of a rectangle. This task involves basic multiplication, a concept easily grasped without advanced math skills.
public class RectangleArea {
public static void main(String[] args) {
double length = 5.0;
double width = 3.0;
double area = calculateArea(length, width);
System.out.println("The area of the rectangle is: " + area);
}
public static double calculateArea(double length, double width) {
return length * width; // Basic multiplication operation
}
}
Why This Code Matters: This example illustrates that you can solve practical problems with fundamental arithmetic. Many programming challenges are less about exceptional math skills and more about logical problem-solving.
Myth 2: Programming is All About Knowing Multiple Languages
The Truth
A common misconception is that to be a good programmer, you must be fluent in numerous programming languages. While learning multiple languages can be beneficial, proficiency in one language—especially the one most relevant to your goals—can be far more advantageous.
Quality Over Quantity
Choosing one language, mastering it, and understanding its core concepts can provide a sturdy foundation upon which you can learn additional languages more easily later. For instance, once you understand Java, picking up Python or JavaScript will become much simpler due to shared programming concepts.
Code Example
Below is a Java snippet that demonstrates the use of an object-oriented approach, a fundamental concept in many programming languages.
class Dog {
String name;
String breed;
Dog(String name, String breed) {
this.name = name;
this.breed = breed;
}
void bark() {
System.out.println(name + " says woof!");
}
}
public class Main {
public static void main(String[] args) {
Dog myDog = new Dog("Buddy", "Golden Retriever");
myDog.bark(); // Output: Buddy says woof!
}
}
Why This Code Matters: The principles of classes and objects in this example are prevalent across many languages. By mastering Java's object-oriented programming I can transfer this knowledge when learning C#, Python, or others.
Myth 3: You Need a Computer Science Degree to Get a Programming Job
The Truth
While a Computer Science degree offers a fantastic foundation, it is not a mandatory requirement for landing a programming job. Many successful programmers do not possess formal education in computer science but have developed skills through self-study, coding boot camps, or extensive personal projects.
Skills Over Credentials
Employers increasingly value practical skills and hands-on experience over formal education. Building a strong portfolio that showcases your projects can often speak louder than a degree.
Code Example
Here’s a simple example of a web application in Java using Spring Boot, demonstrating how practical projects can showcase your skills.
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
@Bean
public CommandLineRunner demo(MyRepository repository) {
return (args) -> {
repository.save(new MyEntity("Example Data"));
System.out.println("Data saved to repository.");
};
}
}
Why This Code Matters: Skills in frameworks like Spring Boot are in-demand, and building small applications can help you build your portfolio, showing potential employers that you are ready to contribute.
Myth 4: Programmers Work Alone
The Truth
Another myth is that programming is a solitary activity. In reality, coding typically involves collaboration with other programmers, designers, project managers, and stakeholders. The ability to work as part of a team is essential in many programming roles, especially as projects grow more complex.
Team Collaboration Tools
Modern collaboration tools, like GitHub for version control, Slack for communication, and Jira for project management, enhance the collaborative nature of programming work.
Code Example
Utilizing Git for version control can be visualized with the following command:
git clone https://github.com/username/repository.git
Why This Code Matters: This command reveals how essential collaboration is in software development—essentially pulling the latest code updates from a shared repository to work on the same project as a team.
Myth 5: You Will Only Use What You Learn in Tutorials
The Truth
While tutorials are an excellent starting point, they are only the beginning of your programming journey. Real-world problems demand creative solutions adapted to specific requirements. You’ll need to think critically and often employ techniques and concepts that go beyond what is covered in tutorials.
Continuous Learning
Programming is an ever-evolving field, and continuous learning through online courses, reading documentation, and engaging in community forums is critical.
Code Example
Let's look at a real-world application: an HTTP request handling scenario, where one might need to parse JSON from a web API.
import org.json.JSONObject;
public class JsonParser {
public static void main(String[] args) {
String response = "{\"name\":\"John\", \"age\":30}";
JSONObject jsonObject = new JSONObject(response);
String name = jsonObject.getString("name");
int age = jsonObject.getInt("age");
System.out.println("Name: " + name + ", Age: " + age);
}
}
Why This Code Matters: This example demonstrates the practical application of JSON parsing, a common requirement for interacting with APIs, illustrating that learning involves adaptability and problem-solving skills beyond tutorials.
Lessons Learned
Programming is rich with opportunities and challenges, but it is important to dismantle the myths that may hinder growth or interest. Understanding that you don’t need to be a math genius, possess multiple languages, have a computer science degree, work alone, or only rely on tutorials can pave the way for lasting success.
As you embark on your programming journey, remember: skills, collaboration, continuous learning, and a passion for problem-solving are your best allies.
For further reading on computer programming skills, check out Codecademy for interactive learning and GitHub for open-source collaboration and community projects.
Whether you are just starting out or looking to deepen your knowledge, demystifying these misconceptions allows you greater freedom to explore the vast landscape of programming with confidence. Happy coding!