Common Pitfalls in Spring Cloud Service Discovery
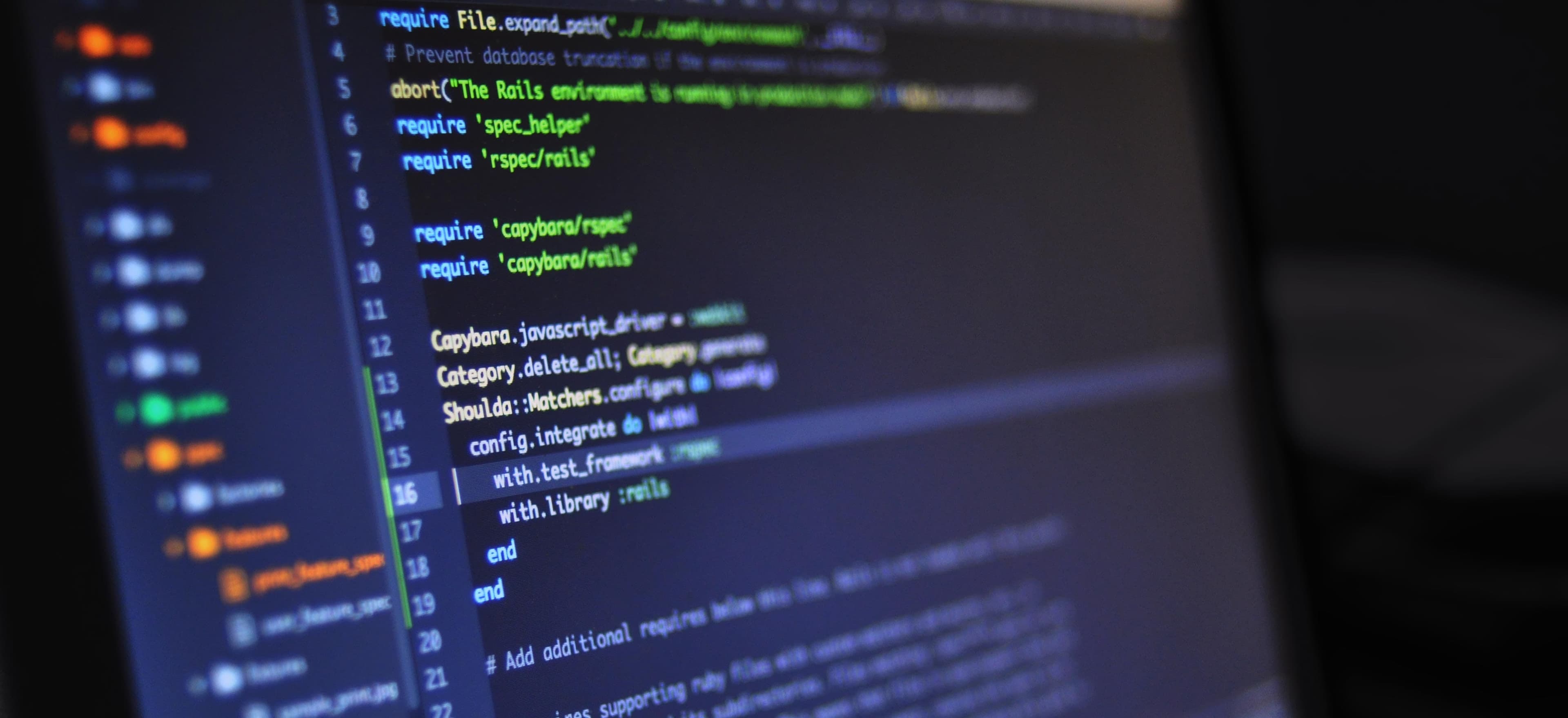
- Published on
Common Pitfalls in Spring Cloud Service Discovery
Spring Cloud Service Discovery is a vital component in cloud-native application ecosystems, facilitating efficient management of microservices by enabling them to discover and communicate with each other. However, developers often encounter pitfalls that can hinder the performance and reliability of their applications. In this blog post, we will discuss common mistakes while implementing Spring Cloud Service Discovery and how to avoid them, accompanied by insightful code snippets and explanations.
What is Spring Cloud Service Discovery?
Spring Cloud Service Discovery simplifies the process of locating service instances in a microservices architecture. It provides tools to manage and locate services through Eureka, Consul, or Zookeeper. For many applications, achieving effective service discovery is critical to ensure smooth communication and scalability in a microservices ecosystem.
Common Pitfalls
1. Overlooking Registration Configuration
One of the first steps to utilizing service discovery is configuring the service registration correctly. A common oversight is failing to set up the appropriate properties in the application configuration file. Without this, the service may not appear in the service registry.
Example Configuration:
spring:
application:
name: my-service
cloud:
discovery:
client:
simple:
instances:
my-service:
- uri: http://localhost:8080
Why This Matters:
Setting the spring.application.name
is crucial as it defines how the service will register itself in the discovery client. Failure to specify this property may lead to issues where your service cannot be found by other microservices.
2. Ignoring Health Checks
Another significant pitfall is neglecting health check configurations. Most service discovery clients provide mechanisms to check the health of services. Without proper health checks, clients may attempt to connect to unhealthy services, leading to errors in communication.
Health Check Example:
management:
health:
indicators:
diskspace:
enabled: true
uptime:
enabled: true
Why Health Checks Are Essential: Regular health checks help ensure only healthy services are part of the service registry. This not only improves system reliability but also enhances user experience by directing requests to functioning services.
3. Not Using Client-Side Load Balancing
Many developers neglect the concept of client-side load balancing offered by Spring Cloud. Relying entirely on server-side load balancing can create bottlenecks and unnecessary latency.
Example of Client Load Balancing with Ribbon:
@RestController
@RequestMapping("/api")
public class MyController {
@Autowired
private RestTemplate restTemplate;
@GetMapping("/service-data")
public String getServiceData() {
return restTemplate.getForObject("http://my-service/data", String.class);
}
}
Why This Matters: By utilizing Spring Cloud Ribbon or Spring Cloud LoadBalancer, the client can distribute requests across multiple instances of a service. This not only reduces the load on any single instance but also enhances the application's resilience.
4. Underestimating Security Concerns
In microservices architecture, security is paramount, yet it's common to see developers overlook it in service discovery configurations. If register or discover URLs are not secured, malicious actors could manipulate them, potentially harming your application's integrity.
Configuring Security:
security:
user:
name: user
password: securepassword
Why Security Is Crucial: Securing service registration prevents unauthorized access and ensures that only verified services communicate with each other. It mitigates risks associated with data leakage and increases overall system robustness.
5. Failing to Handle Service Failures Gracefully
Microservices are inherently susceptible to failures. However, handling these failures poorly can impact the availability of your application. Failing to implement fallback mechanisms when a service is unreachable can lead to significant downtime.
Example Using Circuit Breaker with Resilience4j:
@Retry(name = "serviceRetry", fallbackMethod = "fallback")
public String getServiceData() {
// Make a call to another service
}
public String fallback(Throwable t) {
return "Service is down, please try again later.";
}
Why Fallbacks Are Important: Implementing fallback methods allows you to provide a graceful degradation of service when your services are down, enhancing user satisfaction and reducing the impact of critical failures.
6. Not Understanding Deployment Strategies
Deployments play a crucial role in the reliability of service discovery. Many developers deploy services without strategic consideration of their environments and scaling needs. This can lead to service outages and impact communication between microservices.
Exploring Deployment Styles:
- Blue-Green Deployments: Keeping two identical environments (one active and one idle) facilitates nanosecond switching, ensuring zero downtime.
- Canary Releases: Deploying changes to a small subset of users minimizes risk by validating new features with real traffic.
Why Deployment Strategies Matter: Understanding and selecting the right deployment strategy can vastly improve the robustness and availability of your services. An informed approach reduces the risk of service unavailability during updates.
7. Missing Out on Centralized Configuration
For microservices managed via service discovery, maintaining a centralized configuration is often overlooked. This can lead to configuration discrepancies across multiple services, causing problems in the service interaction lifecycle.
Using Spring Cloud Config:
spring:
cloud:
config:
server:
git:
uri: https://github.com/my-org/my-repo
Why Centralized Configuration Helps: Centralized configuration ensures that all microservices can access the same settings, preventing discrepancies and reducing deployment risks. This promotes a unified approach to configuration management in your services.
Key Takeaways
In conclusion, while Spring Cloud Service Discovery offers powerful capabilities to manage microservices, several common pitfalls can lead to complications. By avoiding the mistakes outlined in this post – such as neglecting health checks, client-side load balancing, and proper security measures – you can significantly enhance the reliability and efficiency of your service discovery implementation. Monitoring deployments and leveraging centralized configuration can further streamline your microservices environment, making it an effective architecture for cloud-native applications.
For more insights into Spring Cloud Service Discovery and microservices, consider following Spring's official documentation, which offers a plethora of resources to help you navigate through these technologies effectively.
Happy coding!