Mastering Local Variable Type Inference: Common Pitfalls
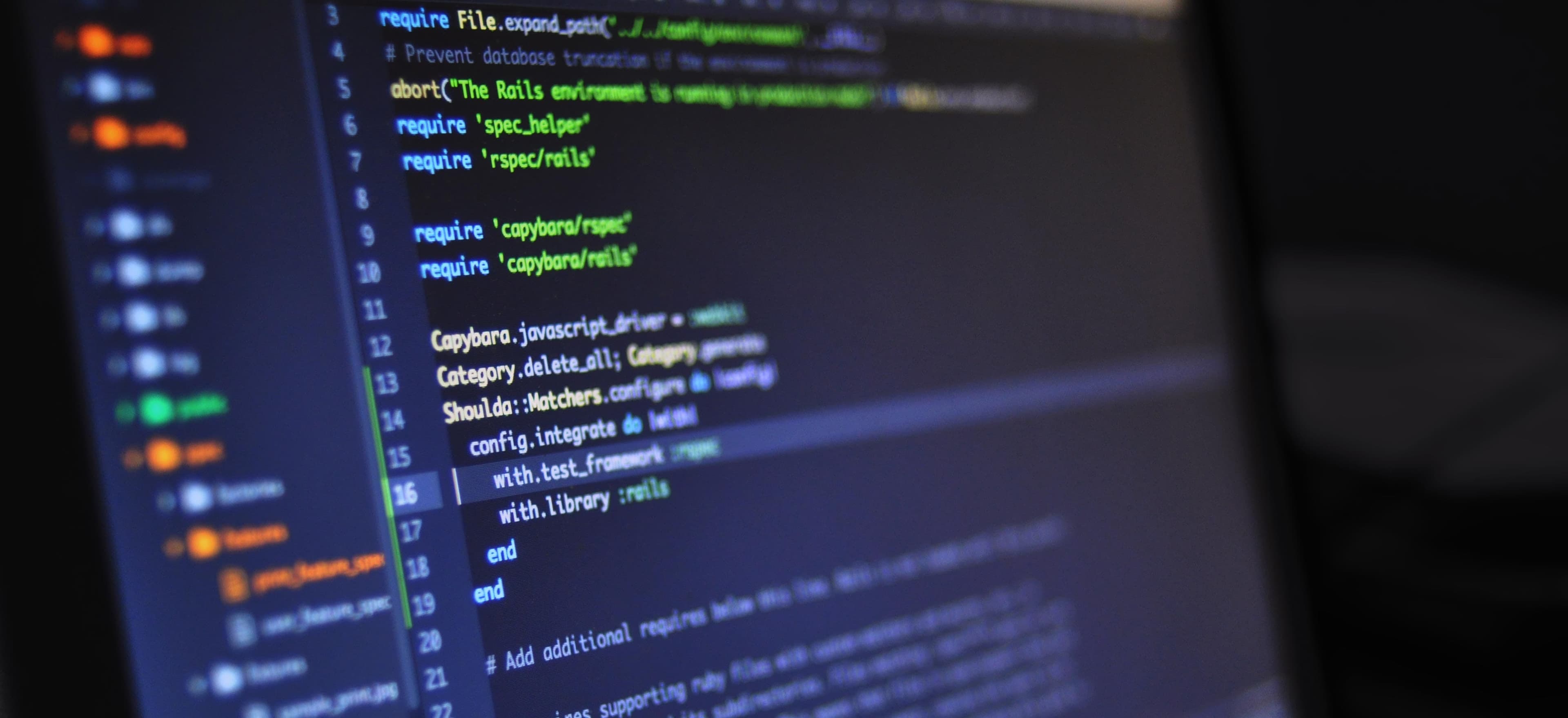
- Published on
Mastering Local Variable Type Inference: Common Pitfalls
In the ever-evolving world of programming, Java developers continually seek to enhance their coding efficiency and maintainability. One of the recent enhancements to the Java language is Local Variable Type Inference, introduced in Java 10 through the var
keyword. This feature allows developers to declare local variables without explicitly specifying their types, relying instead on the compiler to infer it.
While this new capability promotes cleaner code, it can also lead to common pitfalls if not fully understood. In this blog post, we will explore the advantages and potential traps of using local variable type inference in Java. Additionally, we will provide code snippets and commentary to illustrate best practices.
What is Local Variable Type Inference?
Local Variable Type Inference allows you to declare local variables with the var
keyword instead of specifying their type explicitly. Here is a basic example:
var message = "Hello, World!";
In this case, the compiler infers that message
is of type String
. This feature improves code readability by reducing boilerplate type declarations.
Advantages of Using var
- Conciseness: The code becomes cleaner and less verbose.
- Readability: Developers can focus on the logic instead of getting distracted by long type definitions.
- Flexibility: Helps in situations where the type is complex or verbose.
However, despite these benefits, there are pitfalls to watch out for.
Common Pitfalls
1. Loss of Clear Type Information
Using var
can result in code that is difficult to read, especially for developers unfamiliar with the codebase.
var list = new ArrayList<>(); // What type of elements?
Best Practice: Use var
judiciously. When clarity might be compromised, explicitly specify the type.
ArrayList<String> list = new ArrayList<>();
2. Ambiguity in Type Inference
The compiler infers the type based on the initializer. If the initializer involves complex expressions, it might lead to ambiguity.
var result = someMethod() ? "Success" : 100; // What type is result?
In this case, the type of result
becomes ambiguous because it can be either a String
or an Integer
. This ambiguity not only confuses readers but can also lead to compile-time errors.
Best Practice: When dealing with expressions that might lead to ambiguous types, explicitly declare the type.
String result = someMethod() ? "Success" : "Failure"; // Clear and unambiguous
3. Inability to Use var
with Null Initializers
One of the most significant restrictions of var
is that it cannot be used with a null initializer because the type cannot be inferred.
var name = null; // Compilation error: Cannot infer type
Best Practice: Always initialize var
with a non-null value.
var name = "John Doe"; // Valid initialization
4. Limited Scope of var
The scope of variables declared with var
is limited to the block they are declared in. This limitation can sometimes lead to issues when trying to access the variable outside its scope.
if (true) {
var localVariable = "I am local!";
}
// System.out.println(localVariable); // Compilation error: Cannot find symbol
Best Practice: Ensure the variable scope aligns with your intended usage, and explicitly declare it outside the block if needed.
String globalVariable;
if (true) {
globalVariable = "I am accessible!";
}
System.out.println(globalVariable); // Works as intended
5. Misuse in Loops and Streams
While var
can streamline looping constructs and stream operations, it can also lead to unexpected issues, particularly with generic types.
var iterator = list.iterator(); // What type does it return?
Using var
here can obscure the underlying type of the iterator.
Best Practice: Consider using explicit types when working with collections, especially with generics.
Iterator<String> iterator = list.iterator(); // Clear and understandable
Better Alternatives: Contextual Usage
Instead of broadly applying var
, consider it only in contexts where the intent is clear and the type of the variable is evident. Common situations include:
- Simple Records: When dealing with simple values.
var count = 42; // Clearly an int
- Lambdas: Local type inference works well with lambdas.
var action = (String input) -> input.length(); // Clearly a lambda
Bringing It All Together
Local Variable Type Inference using var
in Java is a powerful tool that, when used correctly, can make your codebase cleaner and more readable. However, it's essential to be aware of its limitations and pitfalls.
Always consider whether var
genuinely enhances clarity, and be cautious in situations where it might introduce ambiguity or confusion. Remember the best practices outlined above, and you will be well on your way to mastering local variable type inference in Java.
For further reading on this topic, check out Java SE Documentation and Java Tutorials.
By mastering local variable type inference and recognizing its pitfalls, you can significantly improve your coding practices in Java, ensuring both readability and maintainability in your projects. Happy coding!