Common API Security Flaws and How to Avoid Them
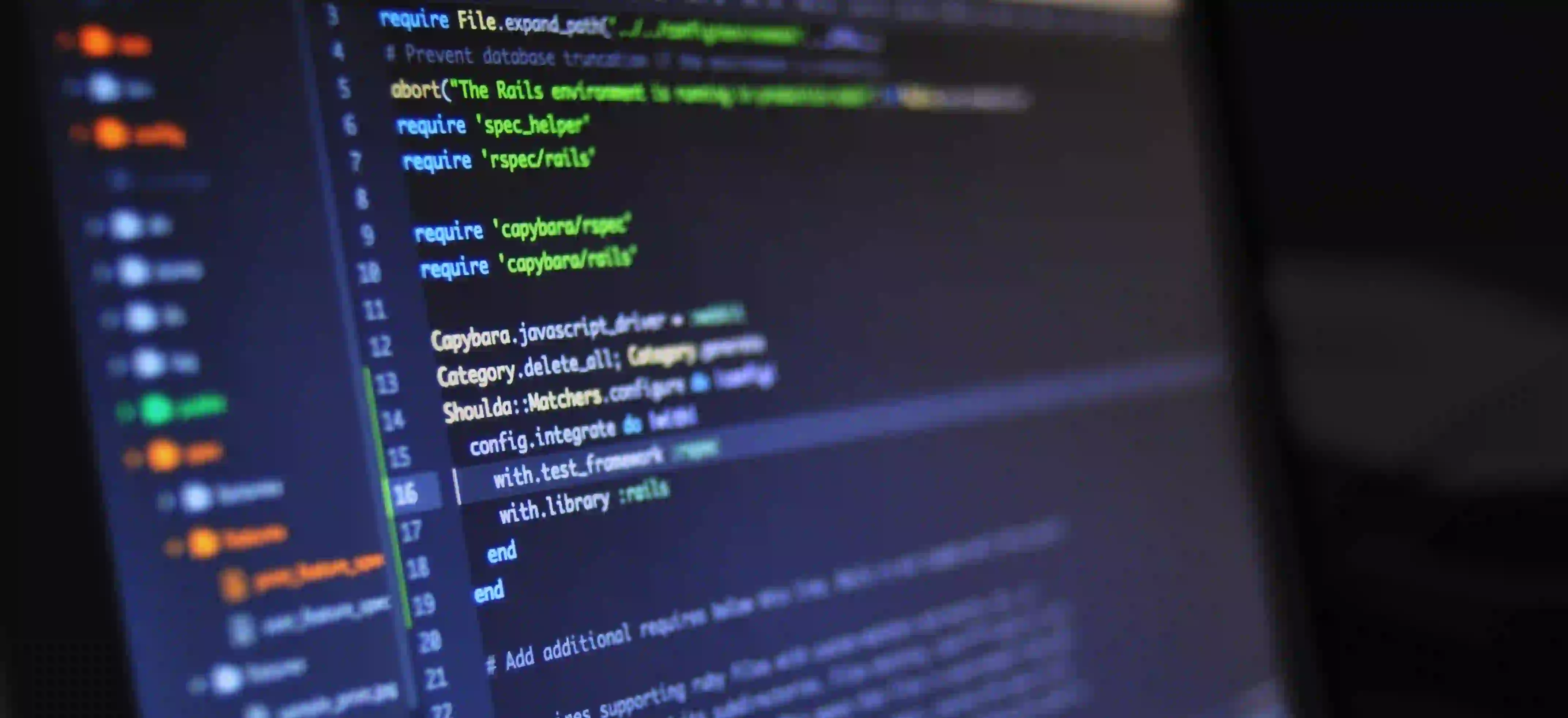
Common API Security Flaws and How to Avoid Them
In today’s digital landscape, APIs (Application Programming Interfaces) serve as pivotal components in software architecture. They empower seamless communication between different software parts, and inevitably, with this seamless integration comes the responsibility of safeguarding sensitive data. Due to their central role, APIs are frequently targeted by malicious actors. This blog delves into the common API security flaws, providing insight into how to identify and mitigate these vulnerabilities.
Understanding API Security
API security pertains to the methods and strategies employed to protect APIs from data breaches, misuse, or other unauthorized access. A robust API security strategy considers various aspects including authentication, authorization, encryption, and logging. Recognizing the importance of these concepts is crucial for any developer working with APIs.
Common API Security Flaws
Let’s explore some of the most prevalent vulnerabilities in APIs.
1. Lack of Authentication
Why it's an issue: Authentication is the first line of defense against unauthorized access. A lack of proper authentication mechanisms can expose sensitive API endpoints to malicious users.
How to Avoid
Implement strong authentication methods such as OAuth 2.0 or API tokens. For example, using OAuth allows third-party applications to access user information without revealing passwords.
// Example of implementing OAuth 2.0 in Java
import org.springframework.security.oauth2.config.annotation.web.configuration.EnableResourceServer;
import org.springframework.security.oauth2.config.annotation.web.configuration.ResourceServerConfigurerAdapter;
@EnableResourceServer
public class ResourceServerConfig extends ResourceServerConfigurerAdapter {
@Override
public void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/api/public").permitAll() // Public API
.anyRequest().authenticated(); // Require authentication for all other requests
}
}
This snippet sets up a basic resource server configuration, allowing public access to specified endpoints while securing others.
2. Insufficient Authorization
Why it's an issue: Once authenticated, users must be authorized to access specific endpoints. Insufficient authorization can allow users to access data or functions they shouldn’t have access to.
How to Avoid
Implement role-based access control (RBAC) or attribute-based access control (ABAC) to stringently define what authenticated users can and cannot do.
// Example of implementing role-based access control in Java
@PreAuthorize("hasRole('ADMIN')")
public ResponseEntity<List<User>> getAllUsers() {
// Only accessible by users with the ADMIN role
return ResponseEntity.ok(userService.findAll());
}
By using the @PreAuthorize annotation, we restrict access to specific actions based on user roles, effectively safeguarding endpoints.
3. Lack of Input Validation
Why it's an issue: APIs need to validate the data they receive. Ignoring input validation can lead to SQL injection, cross-site scripting (XSS), and other forms of attacks that exploit untrusted data.
How to Avoid
Always validate and sanitize input data, especially from untrusted sources. You can do this using frameworks like Hibernate Validator or by implementing custom validators.
// Java example of a simple validation check
import javax.validation.constraints.NotBlank;
public class User {
@NotBlank(message = "Username cannot be empty")
private String username;
// ... other fields and methods
}
By employing Java’s built-in validation constraints, we ensure that user input must meet the specified criteria before processing.
4. Insecure Data Transmission
Why it's an issue: Transmitting sensitive data over unsecured channels can lead to data interception by attackers. Without proper encryption, sensitive information is vulnerable during transit.
How to Avoid
Always use HTTPS to encrypt data in transit. Implement SSL/TLS certificates to secure API endpoints.
// Java example using Spring Boot to enforce HTTPS
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.EnableWebMvc;
@Configuration
@EnableWebMvc
public class WebConfig {
@Bean
public ServletWebServerFactory servletWebServerFactory() {
TomcatServletWebServerFactory factory = new TomcatServletWebServerFactory();
factory.addContextCustomizers(context -> {
context.setSecure(true);
context.setUseHttpOnly(true);
});
return factory;
}
}
This snippet configures an embedded Tomcat server to ensure that it operates securely, enforcing HTTPS across the board.
5. Lack of Rate Limiting
Why it's an issue: Without rate limiting or throttling, APIs can become susceptible to denial-of-service (DoS) attacks, where an attacker overwhelms the API with requests.
How to Avoid
Implement rate limiting using tools or libraries like Bucket4J or Spring Cloud Gateway.
// Example of implementing rate limiting in a Spring application
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class RateLimiterConfig {
@Bean
public RateLimiter rateLimiter() {
return RateLimiter.create(10); // 10 requests per second
}
}
This configuration creates a rate limiter that permits a maximum of 10 requests per second, safeguarding against abuse.
6. Inadequate Logging and Monitoring
Why it's an issue: Without sufficient logging, it’s challenging to trace malicious activities or breaches. This can delay the identification and resolution of security issues.
How to Avoid
Implement comprehensive logging for all API requests and responses, including details about authentication and errors. Tools like Logstash or ELK stack can help in monitoring and analyzing logs.
// Example of logging API requests in Spring Boot
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.web.bind.annotation.*;
@RestController
public class UserController {
private static final Logger logger = LoggerFactory.getLogger(UserController.class);
@GetMapping("/api/users/{id}")
public ResponseEntity<User> getUser(@PathVariable Long id) {
logger.info("Request made to fetch user with ID: {}", id);
// Actual logic to fetch user
}
}
Logging the API requests provides a trail that can be analyzed later to investigate suspicious activities.
The Bottom Line
API security is paramount in preserving the integrity and confidentiality of data. By understanding and addressing common vulnerabilities, developers can create secure APIs that withstand potential threats. It’s crucial to implement robust authentication methods, enforce strict authorization measures, validate user inputs, ensure data transmission security, enforce rate limits, and maintain comprehensive logging.
For a more in-depth understanding of API security best practices, consider checking out resources such as OWASP API Security Top 10 and Crafting an API Security Strategy.
Further Steps
Stay updated with the latest security frameworks and practices to keep your APIs protected as the threat landscape evolves. Adopting a proactive approach to API security not only safeguards data but also fortifies the reputation of your applications.