How to Avoid Common Pitfalls in Threading Stories
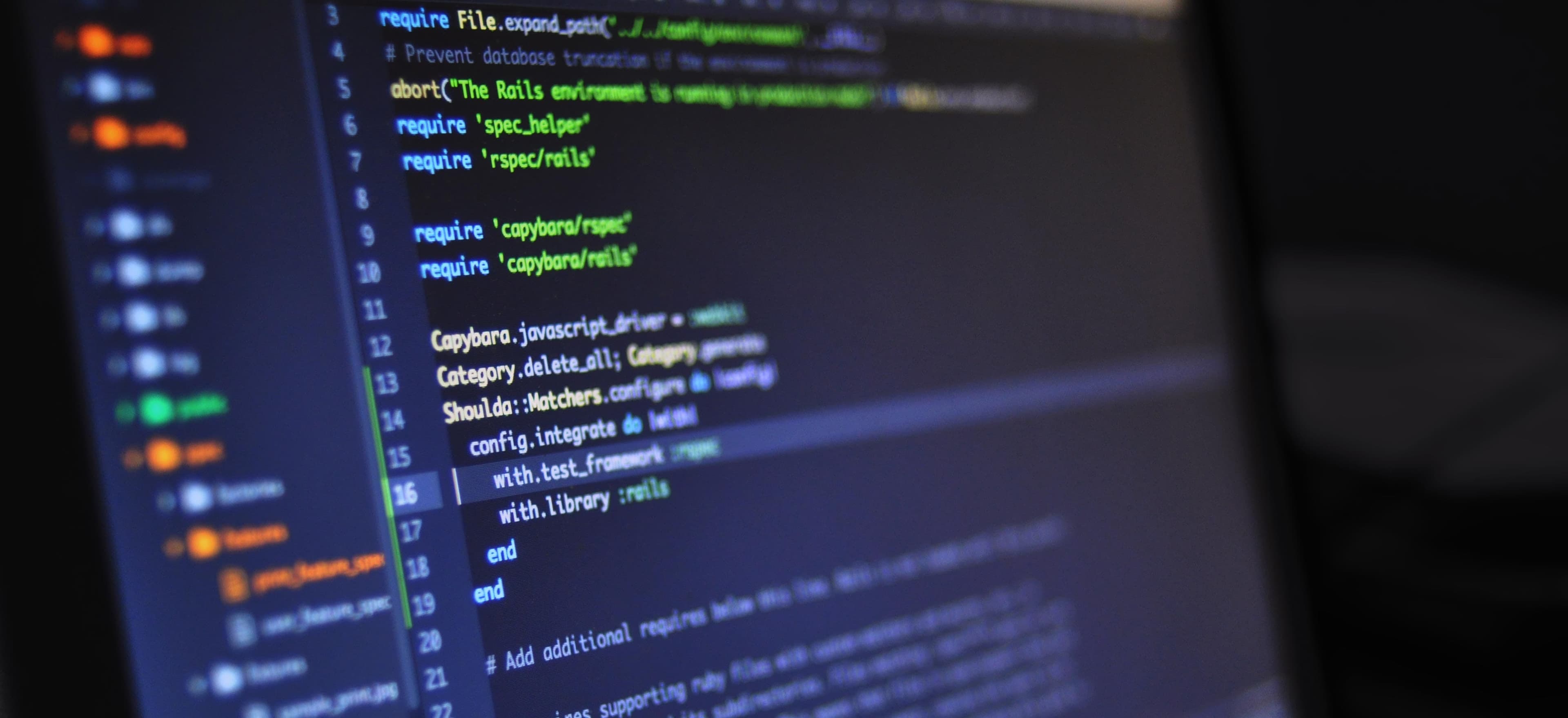
- Published on
How to Avoid Common Pitfalls in Threading Stories in Java
Threading in Java is a powerful concept that lets developers perform multiple operations simultaneously. It can significantly enhance the performance of a program; however, it isn't without its challenges. This blog post discusses common pitfalls in threading and offers strategies to navigate around them. We'll also analyze Java threading concepts through practical examples.
Understanding Threads in Java
Java threads are lightweight subprocesses. They facilitate multitasking and parallelism within a Java application. Before we dive into the pitfalls, let's briefly cover the basics of creating and managing threads.
Creating Threads
You can create threads in Java in two primary ways:
- Extending the Thread Class
- Implementing the Runnable Interface
Using the Runnable Interface
public class MyRunnable implements Runnable {
@Override
public void run() {
System.out.println("Thread is running: " + Thread.currentThread().getName());
}
}
public class ThreadExample {
public static void main(String[] args) {
Thread thread = new Thread(new MyRunnable());
thread.start();
}
}
In this example, we create a class MyRunnable
that implements Runnable
. When the run
method is executed, it displays the thread's name.
Why Use Runnable?
Using Runnable
is often preferred over extending Thread
because it allows your class to extend from another class, enhancing code reusability and design.
Common Threading Pitfalls
1. Thread Safety
Problem: When multiple threads access shared data, it can lead to inconsistencies, as seen in the example below:
public class Counter {
private int count = 0;
public void increment() {
count++;
}
public int getCount() {
return count;
}
}
Analysis: The increment
method is not thread-safe. If two threads call increment
simultaneously, they might read the same value of count
, leading to unexpected results.
Solution: Use synchronized methods or blocks to ensure that only one thread accesses the method at a time.
public synchronized void increment() {
count++;
}
2. Deadlocks
Problem: A deadlock occurs when two or more threads wait on each other to release resources, effectively halting their execution.
Example: Suppose Thread 1 locks Object A and tries to access Object B, while Thread 2 locks Object B and tries to access Object A.
Solution: Use a consistent lock ordering. Always acquire locks in the same order, for example:
public void method1() {
synchronized (objectA) {
synchronized (objectB) {
// critical section
}
}
}
public void method2() {
synchronized (objectA) {
synchronized (objectB) {
// critical section
}
}
}
3. Starvation
Problem: Thread starvation happens when a thread is perpetually denied access to resources it needs for execution, often due to other threads hogging the CPU.
Solution: Use thread prioritization wisely or consider using alternatives like java.util.concurrent
packages which have built-in mechanisms to prevent starvation.
Thread t1 = new Thread(myRunnable1);
Thread t2 = new Thread(myRunnable2);
t1.setPriority(Thread.MIN_PRIORITY);
t2.setPriority(Thread.MAX_PRIORITY);
4. Improper Use of Volatile
Problem: The volatile
keyword ensures visibility of changes to variables across threads, but it's easy to misuse. It doesn't guarantee atomicity.
Example:
public class Flag {
private volatile boolean flag = false;
public void setFlag() {
flag = true;
}
}
Analysis: While the flag
is visible to all threads immediately, modifying the value is not atomic. If you check and set flag
in one thread while another is doing the same, it may lead to errors.
Solution: Use AtomicBoolean
or synchronized methods for actions that require both reading and writing.
import java.util.concurrent.atomic.AtomicBoolean;
public class Flag {
private AtomicBoolean flag = new AtomicBoolean(false);
public void setFlag() {
flag.set(true);
}
}
5. Lack of Proper Exception Handling
Problem: Neglecting to handle exceptions in a thread can cause the entire thread to terminate, making it difficult to diagnose issues.
public class ExceptionHandledThread implements Runnable {
@Override
public void run() {
try {
// Code that may throw an exception
} catch (Exception e) {
System.err.println("Thread encountered an exception: " + e.getMessage());
}
}
}
Solution: Always catch and handle exceptions appropriately within worker threads.
Ensuring Efficient Multithreading
Utilize the ExecutorService
Instead of creating and managing threads manually, consider using the ExecutorService
framework. It simplifies thread management and enhances performance through thread pooling.
Example:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ExecutorServiceExample {
public static void main(String[] args) {
ExecutorService executorService = Executors.newFixedThreadPool(5);
for (int i = 0; i < 10; i++) {
executorService.execute(new MyRunnable());
}
executorService.shutdown();
}
}
By using ExecutorService
, we can efficiently manage multiple threads without the overhead of manual instantiation and lifecycle management.
Wrapping Up
Threading in Java is a critical skill for developers, bringing performance improvements and efficiency to applications. However, it comes with its own set of challenges. By understanding the common pitfalls outlined in this post and employing the suggested strategies, developers can create robust, thread-safe applications.
For more on threads and concurrency, the official Java documentation provides extensive resources here.
By staying informed and practicing good threading practices, you can enhance the reliability and performance of your Java applications. Happy coding!