Overcoming Common MapReduce Performance Bottlenecks
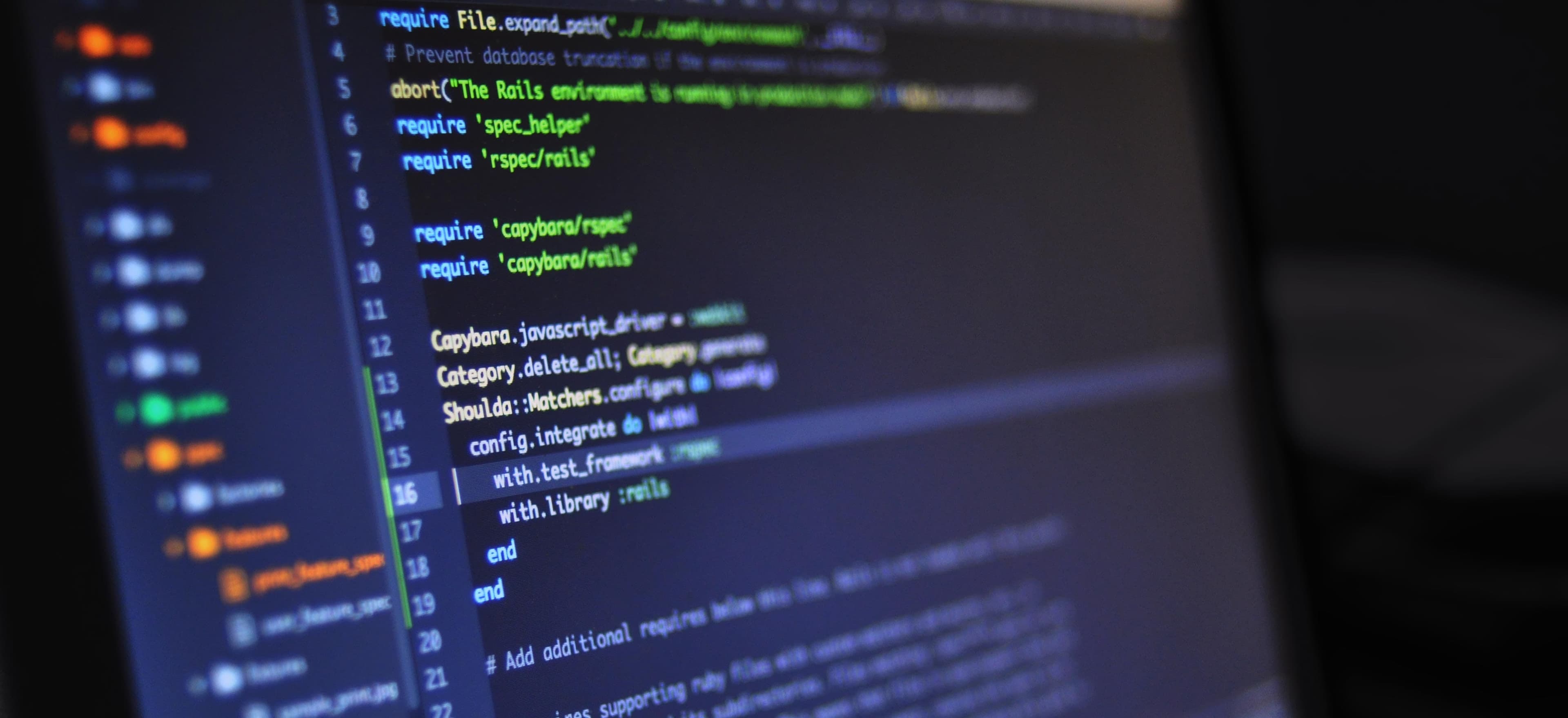
- Published on
Overcoming Common MapReduce Performance Bottlenecks
MapReduce is a powerful paradigm for processing large datasets in a distributed manner. However, as with any system, performance bottlenecks can occur that hinder the speed and efficiency of your data processing jobs. This blog post will delve into the most common performance bottlenecks in MapReduce applications and provide actionable strategies to overcome them.
Understanding the Basics of MapReduce
Before diving into performance enhancements, let’s briefly recap how MapReduce operates.
MapReduce consists of two main phases:
-
Map Phase: In this phase, the input data is split into smaller chunks, processed by the Mapper functions, and transformed into key-value pairs.
-
Reduce Phase: The key-value pairs are then shuffled, sorted, and sent to Reducers that aggregate the values associated with the same key.
This model can effectively utilize a distributed computing architecture, but it also presents multiple opportunities for inefficiencies.
Common Performance Bottlenecks
Let's discuss some of the predominant bottlenecks that occur in MapReduce performance:
1. Shuffle and Sort Phase
The shuffle and sort phase occupies the bulk of your job's runtime. During this stage, data is transferred among nodes, and if not optimized, it can lead to prolonged job durations.
Optimization Techniques
- Combiner: Use a combiner to minimize the amount of data that needs to be shuffled. The combiner acts as a mini-reducer which processes output from the mapper before it's sent across the network.
public class SumCombinator extends Reducer<Text, IntWritable, Text, IntWritable> {
public void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
int sum = 0;
for (IntWritable val : values) {
sum += val.get();
}
context.write(key, new IntWritable(sum));
}
}
Why: It reduces the data volume transferred, saving bandwidth and ultimately leading to faster execution times.
- Adjusting the Number of Partitions: By increasing the number of partitions, you can speed up the shuffle phase, but be careful not to set this too high as it can lead to unnecessary overhead.
2. Input and Output Format
The choice of input and output formats can significantly affect your job's performance. Standard text input and output formats may not be suitable for all data types.
Optimization Techniques
- Custom Input Formats: Create a custom input format that can parse data efficiently, reducing the overhead of loading large files.
public class CustomInputFormat extends FileInputFormat<LongWritable, Text> {
@Override
protected boolean isSplitable(JobContext context, Path path) {
// Customized split logic
return false; // Disable splitting for certain files
}
}
Why: By controlling how data is split and processed, you optimize reading times.
3. Running Too Many Small Map Tasks
When data is broken into tiny chunks, the overhead associated with managing many small tasks can slow down your job.
Optimization Techniques
-
Data Locality: Use data locality to your advantage by ensuring that data is processed on the node where it is stored. This can be achieved through appropriate data partitioning.
-
Input Size Management: Aim for larger input sizes. Ideally, each map task should process enough data to balance load across nodes while minimizing overhead.
4. Resource Mismanagement
Inefficient use of resources (such as CPU and memory) can also create bottlenecks.
Optimization Techniques
-
Tuning Resource Allocation: Carefully allocate memory and CPU resources based on workload profiles. Monitor jobs to find the optimal settings using tools like Hadoop's ResourceManager.
-
YARN Configuration: If using YARN, ensure that the memory and CPU settings for containers are properly configured to handle job load efficiently.
5. Inefficient Algorithms
The underlying algorithm can greatly affect the performance of your MapReduce job.
Optimization Techniques
-
Algorithm Choice: Opt for more efficient algorithms. Sometimes, a different algorithm might yield better performance without heavy modifications to your processing pipeline.
-
Data Structures: Choose the right data structures for the tasks at hand. For example, using HashMap instead of TreeMap can dramatically improve the speed of key-value data aggregation.
6. Ineffective Garbage Collection
Java's garbage collection (GC) can be an unsuspected source of delays. Long pauses during GC can stall your MapReduce jobs.
Optimization Techniques
-
JVM Tuning: Increase the heap size for your JVM to handle larger data and reduce frequent garbage collections.
-
Garbage Collector Selection: Explore different GC algorithms (like G1 or CMS) that can be better suited for the workloads your job is processing.
# Setting JVM options
export HADOOP_HEAPSIZE=2048
Why: More heap space can reduce the frequency of GC cycles, leading to smoother execution.
Lessons Learned
Even though MapReduce is a robust architecture for handling big data processing, several performance bottlenecks can arise. Understanding these potential pitfalls and implementing effective optimization strategies can significantly improve job performance.
For more in-depth tutorials and discussions, check out Hadoop Documentation, which provides a plethora of resources for better understanding and maximizing your MapReduce applications. By tackling these common bottlenecks, you'll be better equipped to harness the full power of MapReduce, ensuring efficient data processing solutions for your organization.
Further Reading
For those interested in diving deeper into MapReduce optimization, the following resources may prove insightful:
- Hadoop: The Definitive Guide by Tom White
- MapReduce Design Patterns by Donald Miner & Andrew Yu
- Optimizing Hadoop MapReduce - An article discussing advanced optimization strategies.
By employing the techniques outlined in this post, you can enhance the speed and efficiency of your MapReduce jobs, making data processing a streamlined and effective task.
Checkout our other articles