Avoiding Premature Optimization: Know When to Optimize
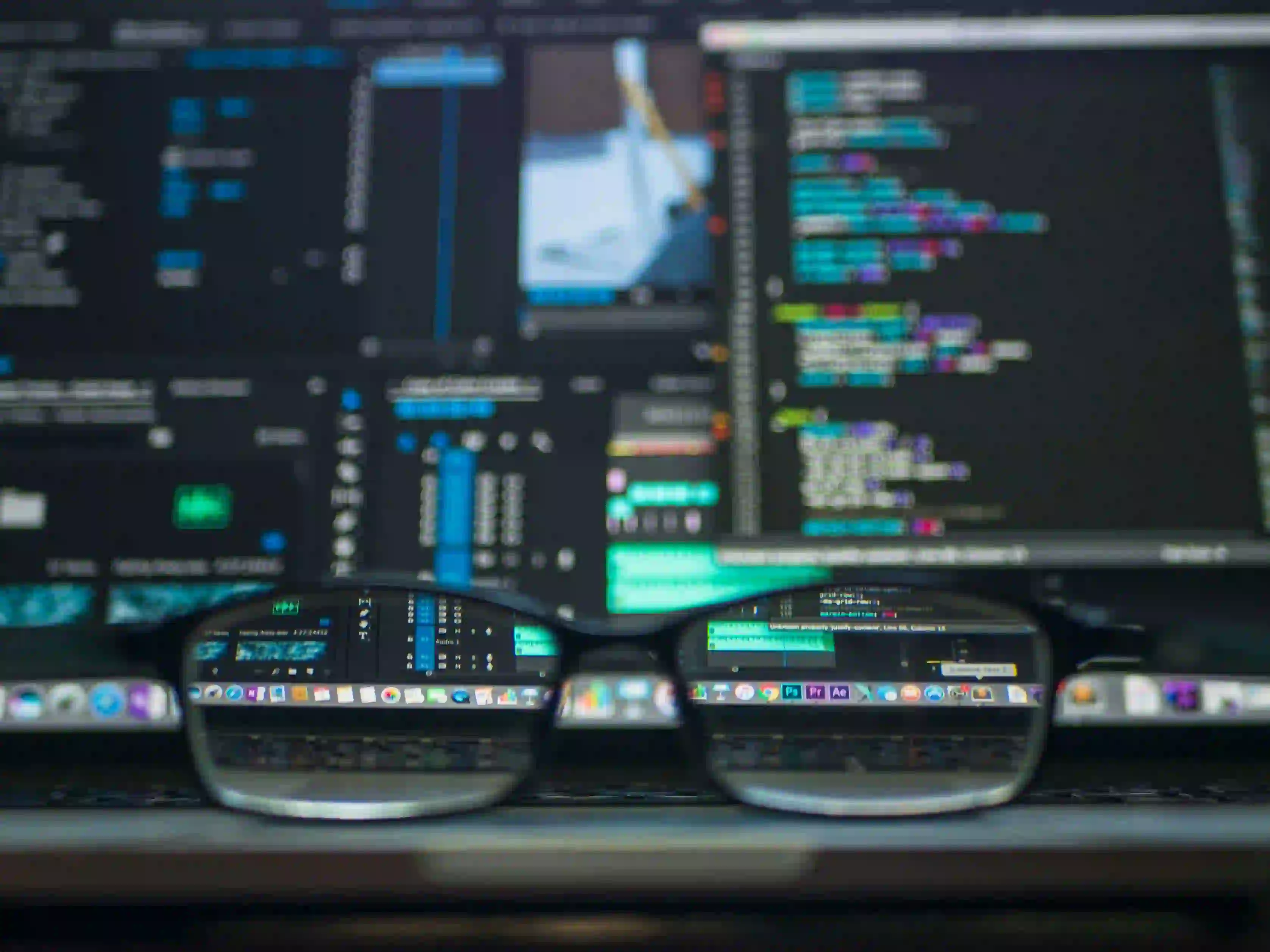
Avoiding Premature Optimization: Know When to Optimize
In the realm of software development, the phrase "premature optimization is the root of all evil" — coined by Donald Knuth — resonates deeply with many experienced developers. It serves as a cautionary reminder that optimizing code before understanding its functionality and bottlenecks can lead to unnecessary complexity and wasted resources. In this blog post, we will explore the concept of premature optimization, delve into its implications, and provide practical guidelines on when to optimize your Java code.
What is Premature Optimization?
Premature optimization refers to the act of making certain code paths highly efficient without a clear understanding of their performance impact. Often, developers focus on micro-optimizations, thinking they will improve overall performance, but this may distract them from building a well-structured application.
Effects of Premature Optimization
- Complexity: Adding unnecessary complexity can make the codebase harder to maintain and understand.
- Runtime Costs: Time spent on optimization could be better spent on features or fixing bugs.
- Technical Debt: Premature optimizations can introduce inconsistencies in the code, leading to increased technical debt.
The Right Time to Optimize
Understanding when to optimize your Java code is crucial. It hinges on two critical principles: Profiling and Measuring Performance.
Step 1: Identify Bottlenecks with Profiling
Profiling helps identify sections of your code where performance lags. Java provides profiling tools like VisualVM and JProfiler that can help visualize memory consumption and CPU usage.
// Example: Using VisualVM for Profiling
public class ImageProcessor {
public void processImages(List<Image> images) {
for (Image img : images) {
img.compress(); // Assume compress() is a costly operation
}
}
}
Using a profiler on processImages
, you might find that the compress()
method is consuming most of the CPU time.
Why Profiling is Important
- It identifies genuine performance hotspots.
- It allows you to focus your optimization efforts on areas that matter.
- It provides a benchmark to measure the impact of your optimizations later.
Step 2: Measure Performance
Using built-in tools like JMH (Java Microbenchmark Harness), you can measure the performance of specific code sections accurately.
import org.openjdk.jmh.annotations.*;
@State(Scope.Thread)
public class BenchmarkExample {
@Benchmark
public void testMethod() {
// Code to measure
for (int i = 0; i < 1000; i++) {
process(i);
}
}
private void process(int i) {
// Simulates a process
}
}
Importance of Measurement
- Accurate time tracking allows you to compare before and after states.
- Engaging in micro-benchmarking can lead to understanding the impact of different implementations.
Common Mistakes and How to Avoid Them
While learning when to optimize is essential, knowing common mistakes can also aid in avoiding premature optimization. Here are a few pitfalls to watch out for:
1. Over-engineering
Adding unnecessary abstractions and enhancements before they’re justified can lead to a confusing and bloated codebase.
Example: Unnecessary Use of Design Patterns
In many cases, developers jump to use design patterns without understanding whether they are needed.
// Over-engineered Singleton example
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton(); // Performance impact in multi-threading
}
return instance;
}
}
2. Ignoring Readability
Optimization shouldn’t come at the cost of code clarity. Always choose code that is easier to understand over the most optimized code.
3. Premature Data Structure Decisions
Choosing data structures early in the development cycle without a solid understanding of usage patterns can result in suboptimal choices.
// Using an ArrayList when a LinkedList is required
List<String> list = new ArrayList<>();
// If frequent insertions/removals are needed
Best Practices for Optimization
Now that we’ve established when not to optimize, let’s move on to best practices that ensure efficient and maintainable code when the time for optimization comes.
1. Optimize After Measuring
Always measure performance first, optimize second. The aforementioned profiling tools will help you decide which areas to enhance.
2. Prioritize Readability
Refactor code for optimization while keeping readability intact. Write comments explaining why certain optimizations were made.
3. Use Caching Wisely
Implement caching judiciously. It can significantly speed up applications but may lead to stale data if not managed properly.
// Simple caching example
public class Cache {
private Map<String, CachedObject> cache = new HashMap<>();
public CachedObject fetch(String key) {
return cache.computeIfAbsent(key, k -> expensiveOperation());
}
private CachedObject expensiveOperation() {
// Simulate a time-consuming process
}
}
4. Favor Built-in Libraries
Leverage Java’s built-in libraries that are often optimized. Java Collections Framework, for instance, provides various data structures that are efficient for common tasks.
5. Keep Learning and Adapting
Technology constantly evolves. Keeping up-to-date with the latest best practices through resources like Oracle's Java Documentation or Java Performance Tuning Guides can offer new insights into when and how to optimize.
Lessons Learned
Avoiding premature optimization is about making informed decisions that balance performance gains with code maintainability. By firmly establishing when to optimize—through profiling and measuring performance—you can ensure that your efforts yield substantial benefits without introducing unnecessary complexity into your codebase.
Always remember: the goal of optimization is to enhance user experience without compromising the code's integrity. When your application is consistently performing well, and your users are satisfied, you've struck the right balance.
Optimization, when done right, can be a powerful ally. Now go forth and optimize wisely!