Solving Common Integration Issues with Spring, Velocity, and Tiles
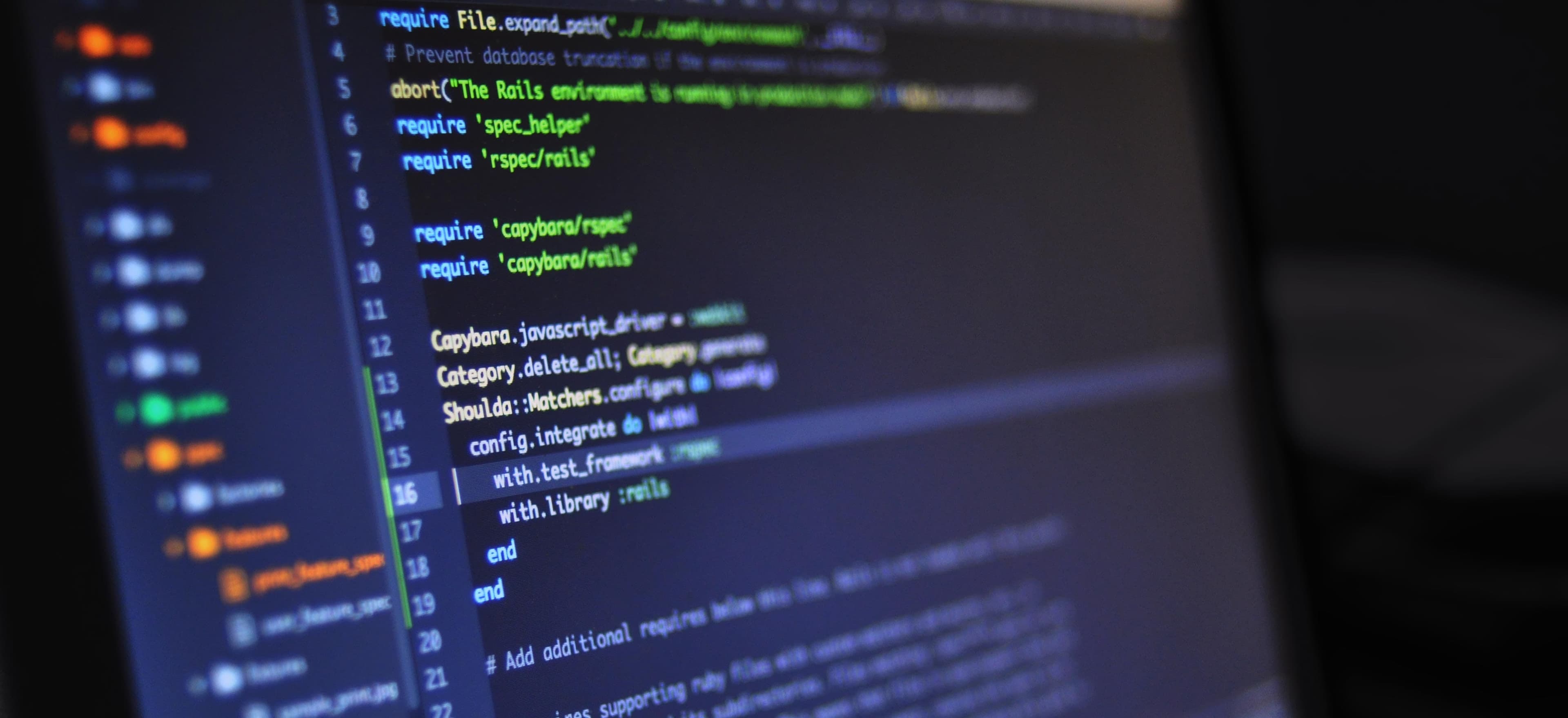
- Published on
Solving Common Integration Issues with Spring, Velocity, and Tiles
When building web applications in Java, combining frameworks efficiently can be a daunting endeavor. Spring, Velocity, and Tiles are a trio that can work hand-in-hand to create powerful, maintainable applications. However, integrating these frameworks presents unique challenges. In this blog post, we will explore common integration issues developers face and how to effectively resolve them.
Understanding the Frameworks
Spring Framework
Spring is a powerful framework that offers comprehensive infrastructure support for Java applications. By providing dependency injection, transaction management, and various modules, Spring alleviates boilerplate code and promotes better design patterns (like MVC).
Velocity Template Engine
Velocity is a Java-based template engine that allows developers to create web pages by separating business logic from presentation logic. This separation enhances ease of maintenance and promotes cleaner code.
Tiles
Apache Tiles is a templating framework that provides a way to define reusable page layout templates. It works as an abstraction layer over different views (JSPs, for example), enabling the reuse of common elements like headers and footers.
Integration Challenges
Here, we’ll explore common issues you may encounter when integrating Spring, Velocity, and Tiles, along with practical solutions.
1. Configuration Complexity
The Issue
Integrating these three frameworks can lead to a complex configuration setup. Without a clear structure, developers often find it challenging to manage the interdependencies.
The Solution
Utilizing Spring's XML or Java-based configuration can greatly ease this transition. Here’s a simple XML setup for integrating these three frameworks:
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="viewResolver" class="org.springframework.web.servlet.view.UrlBasedViewResolver">
<property name="prefix" value="/WEB-INF/views/"/>
<property name="suffix" value=".vm"/>
</bean>
<bean id="tilesConfigurer" class="org.apache.tiles.impl.basic.BasicTilesConfiguration">
<property name="definitions">
<list>
<value>/WEB-INF/tiles-defs.xml</value>
</list>
</property>
</bean>
<bean id="tileViewResolver" class="org.springframework.web.servlet.view.tiles2.TilesViewResolver"/>
</beans>
Why This Works: By declaring view resolvers and the Tiles configuration bean, you create a cohesive environment that Spring can manage. This allows Spring to resolve views effectively, providing a smooth interface between your controllers, Velocity templates, and Tiles layouts.
2. Page Layout Incompatibility
The Issue
When using Tiles and Velocity together, you may encounter issues with page layout and content rendering. For example, occasionally, a component may not display as expected.
The Solution
Ensure that you are configuring your Tiles definitions correctly. Here is an example of how to define a Tiles layout:
<tiles-definitions>
<definition name="baseLayout" template="/WEB-INF/layouts/base.vm">
<put-attribute name="header" value="/WEB-INF/includes/header.vm"/>
<put-attribute name="footer" value="/WEB-INF/includes/footer.vm"/>
<put-attribute name="content" value="/WEB-INF/views/content.vm"/>
</definition>
</tiles-definitions>
Why This Works: This layout definition serves as a base for all your views, allowing a common header and footer to be reused. This makes maintenance simpler, especially when it comes time to apply changes across multiple views.
3. Controller Mappings
The Issue
Using Spring’s @Controller
annotations to map your requests can sometimes lead to confusion when rendering views. Without adhering to a consistent naming convention, it can be easy to overlook where a view is being served from.
The Solution
Stick to consistent naming conventions. Here’s an example of how to define a controller that returns a view:
@Controller
public class HomeController {
@RequestMapping(value = "/home", method = RequestMethod.GET)
public String showHome(Model model) {
model.addAttribute("message", "Welcome to our site!");
return "home"; // Name of the view without the suffix
}
}
Why This Works: This code snippet adheres to clear conventions. It clearly states which view to render based on the return string. In a complex app, where multiple controllers exist, this can prevent mix-ups associated with view names.
4. Common Dependency Errors
The Issue
While integrating frameworks, you might encounter dependency issues, often seen in the form of ClassNotFoundException or NoClassDefFoundError.
The Solution
Use Dependency Management tools like Maven or Gradle effectively. Here’s an example using Maven to add required dependencies:
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.3.6</version>
</dependency>
<dependency>
<groupId>org.apache.velocity</groupId>
<artifactId>velocity-engine-core</artifactId>
<version>1.7</version>
</dependency>
<dependency>
<groupId>org.apache.tiles</groupId>
<artifactId>tiles-core</artifactId>
<version>3.0.8</version>
</dependency>
</dependencies>
Why This Works: This ensures each framework’s functionality is present in your application context. Inter-framework dependencies often lead to runtime errors if specific versions are not consistently aligned.
5. Testing Integrated Frameworks
The Issue
Testing components of your application can be cumbersome when additional layers of rendering are introduced by Velocity and Tiles.
The Solution
Mock the components using frameworks like Mockito or Spring Test. Here’s a small test example demonstrating this approach:
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(classes = {WebConfig.class})
public class HomeControllerTest {
@Autowired
private WebApplicationContext webApplicationContext;
private MockMvc mockMvc;
@Before
public void setUp() {
mockMvc = MockMvcBuilders.webAppContextSetup(webApplicationContext).build();
}
@Test
public void testHomePage() throws Exception {
mockMvc.perform(get("/home"))
.andExpect(status().isOk())
.andExpect(view().name("home"))
.andExpect(model().attributeExists("message"));
}
}
Why This Works: This ensures complete but isolated testing of your controller while mocking the interaction with the view layer. This strategy helps identify and address issues early in the development process.
Best Practices to Keep in Mind
Keep Dependencies Updated
Regularly update your libraries to take advantage of the latest features, bug fixes, and performance improvements.
Document Your Configuration
Maintain clear documentation about the setup process so any developer can understand how the frameworks are integrated, which aids in onboarding and troubleshooting.
Refactor Often
As your project scales, refactoring will help you keep your codebase clean and maintainable. Look for common patterns to promote reusability, such as creating custom tags or helper classes.
A Final Look
Integrating Spring, Velocity, and Tiles provides the opportunity to streamline web applications significantly. By understanding and addressing common integration challenges, developers can create well-structured web applications that are easy to maintain and enhance.
In your journey with these frameworks, remember that learning from challenges is part of the process. For more information on Spring, consult the Spring Framework Documentation. To dive deep into templating with Velocity, check the Velocity User Guide.
Integrate wisely, and happy coding!