Understanding Time Complexity of Linear Search in Java
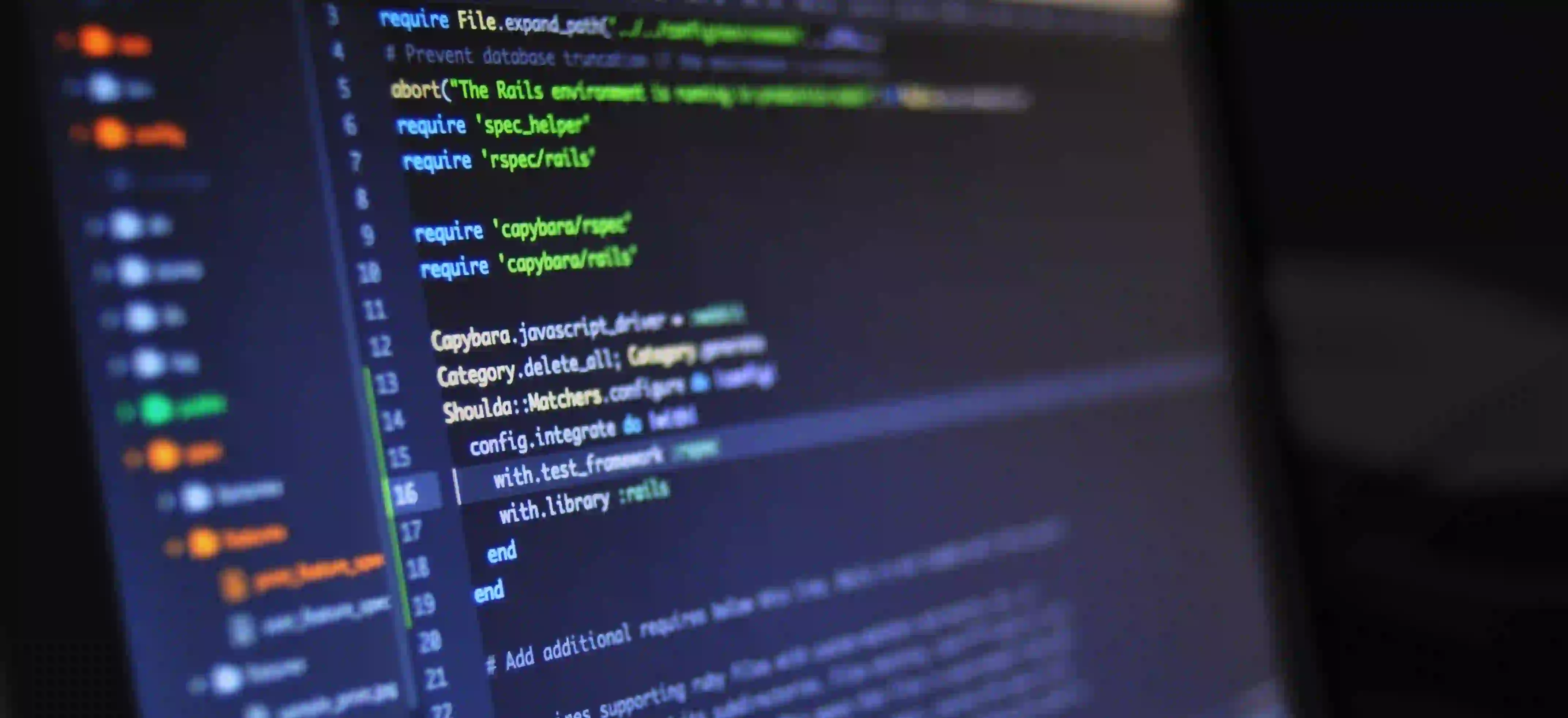
Understanding Time Complexity of Linear Search in Java
When processing data, understanding the efficiency of your algorithms is crucial. One fundamental algorithm is linear search. In this blog post, we will delve into how linear search works in Java, its time complexity, and why it is important for beginners to grasp these concepts.
What is Linear Search?
Linear search is the simplest searching algorithm. It checks each element of the collection one by one until the desired value is found or all elements have been checked. It is straightforward and easy to implement, making it an excellent starting point for beginners in algorithm design.
Here’s a simple implementation of linear search in Java:
public class LinearSearch {
public static int linearSearch(int[] array, int target) {
for (int i = 0; i < array.length; i++) {
if (array[i] == target) {
return i; // Return the index of the target
}
}
return -1; // Return -1 if the target is not found
}
}
Code Explanation
- Method Signature:
public static int linearSearch(int[] array, int target)
defines the method which takes an integer array and a target value to search for.
- Loop through the Array:
for (int i = 0; i < array.length; i++)
iterates through each element of the array.
- Comparison:
if (array[i] == target)
checks if the current element matches the target.
- Return Index:
- If found, it returns the index. If not found after checking all elements, it returns -1.
This implementation is efficient for small or unsorted datasets but can become inefficient as the dataset size grows.
Time Complexity of Linear Search
Time complexity is a way to express the efficiency of an algorithm in relation to the size of the input data. For linear search:
- Best Case: O(1)
- Average Case: O(n)
- Worst Case: O(n)
Best Case Scenario
In the best case, the target value is the first element of the array. Therefore, the algorithm only needs one iteration. This scenario is represented as O(1).
Average and Worst Case Scenario
In the average and worst-case scenarios, the algorithm may need to check all n elements in the array. Thus, it performs on average n/2 checks, resulting in a linear time complexity of O(n).
Visualization of Time Complexity
To visualize this, imagine searching through an array of ten elements. The time taken to find the element (or determine it's not there) increases linearly as the array size increases.
- O(1): The element is the first one.
- O(n): The element is the last one or not present.
When to Use Linear Search
Linear search is often used:
- For Small Arrays: The performance penalty becomes negligible.
- Unsorted Data: When sorting the data isn't feasible or practical.
- Simplicity: In scenarios where code clarity takes precedence over performance.
If you require faster searching in large datasets, you might consider other algorithms such as binary search, which is O(log n), but it requires sorted data.
For an in-depth comparison of various search algorithms, check out this comprehensive article on searching algorithms.
Final Considerations
Understanding the time complexity of linear search is essential in computer science. Not only does it give you insight into algorithm efficiency, but it also aids in making informed decisions about which searching mechanism to employ based on your specific needs.
Linear search provides a vital lesson in the basic principles of searching algorithms. Knowing when to utilize linear search—especially in unsorted or small data sets—enhances your toolkit as a programmer.
Summary of Key Points
- Linear search goes through each element until it finds the target or exhausts the array.
- Efficient for small or unsorted datasets.
- Time complexity:
- Best Case: O(1)
- Average/Worst Case: O(n)
Harnessing these insights into the time complexity of linear search allows for better decision-making as you build complex systems or applications. Embrace the learning curve, and let this fundamental algorithm be a stepping stone into the world of algorithm design.
Further Reading
If you're interested in diving deeper into algorithms, take a look at the following resources:
- Introduction to Algorithms - A staple textbook in the field of computer science.
- Big O Cheat Sheet - An excellent resource summarizing time complexities of various algorithms.
Engage with these learning materials to expand your understanding of algorithmic efficiency and performance. Happy coding!