Overcoming Thread Safety: The Power of Synchronized Decorators
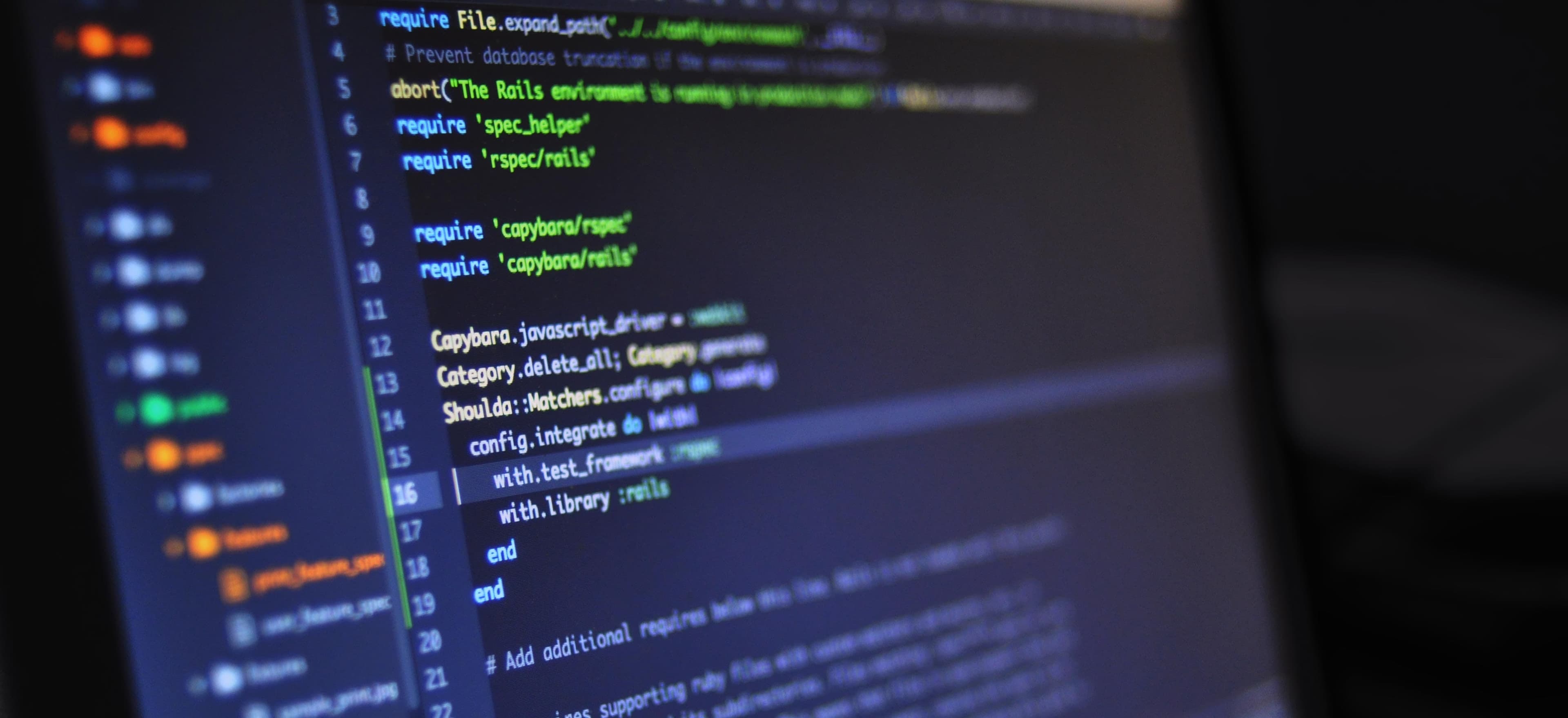
- Published on
Overcoming Thread Safety: The Power of Synchronized Decorators
In today's fast-paced world of software development, crafting applications that provide a seamless user experience is crucial. One of the often-overlooked aspects of this is thread safety. When multiple threads access shared data, it can lead to unpredictable behavior and bugs that are notoriously difficult to trace. This blog post aims to elucidate the concept of thread safety in Java and introduce the use of synchronized decorators as a powerful tool for overcoming these challenges.
Understanding Thread Safety
Thread safety is the property that ensures that shared data structures are used by multiple threads without causing any undesirable interactions. In Java, this is particularly important because Java applications can perform numerous operations concurrently. Without proper synchronization, condition races, data inconsistency, and unpredictable behaviors can occur.
To grasp the importance of thread safety, consider this simple analogy: imagine a bank with one cashier and a long queue of customers. If multiple customers attempt to withdraw money simultaneously, confusion will ensue. The cashier must manage each transaction carefully, just like threads managing shared resources in a multithreaded program.
Why Use Synchronized Decorators?
Synchronized decorators provide a clear and efficient way to enforce thread safety on shared resources. They act as wrappers around methods or objects, ensuring that only one thread accesses the synchronized code block at a time.
Using synchronized methods or blocks can be tedious and cluttered, especially when you have multiple methods needing thread safety. Synchronized decorators simplify this process by abstracting the synchronization mechanism, allowing for cleaner and more maintainable code.
The Basics of Synchronized Decorators
Before we delve deeper into synchronized decorators, let’s review the core syntax for synchronized methods in Java.
public synchronized void updateAccountBalance(double amount) {
this.balance += amount;
}
In the above example, the synchronized
keyword ensures that only one thread can execute updateAccountBalance()
at a time. However, using this approach for multiple methods can quickly become unwieldy.
Implementing Synchronized Decorators
To create a synchronized decorator in Java, you typically define a functional interface and use that interface with a method that handles the synchronization. Here’s a step-by-step example:
Step 1: Define a Functional Interface
A functional interface can be any interface with a single abstract method. This allows us to create a lambda expression later.
@FunctionalInterface
interface SynchronizedAction {
void execute();
}
Step 2: Create a Synchronized Decorator Method
Next, we need a method that accepts our functional interface and synchronizes execution.
public class SynchronizedDecorator {
public static void synchronizedExecute(SynchronizedAction action) {
synchronized (SynchronizedDecorator.class) {
action.execute();
}
}
}
Here, we are synchronizing the execution of any code block that the execute
method will represent. The use of SynchronizedDecorator.class
ensures that the lock is applied appropriately.
Step 3: Use the Synchronized Decorator
Now we can use this decorator in our application wherever thread safety is required. For instance, let’s apply it to an Account class that manipulates the balance.
public class Account {
private double balance;
public Account(double initialBalance) {
this.balance = initialBalance;
}
public void deposit(double amount) {
SynchronizedDecorator.synchronizedExecute(() -> {
balance += amount;
System.out.println("Deposited: " + amount + ", New Balance: " + balance);
});
}
public void withdraw(double amount) {
SynchronizedDecorator.synchronizedExecute(() -> {
if (amount <= balance) {
balance -= amount;
System.out.println("Withdrew: " + amount + ", New Balance: " + balance);
} else {
System.out.println("Withdrawal failed: Insufficient funds");
}
});
}
}
Commentary on the Code Snippet
- Functional Interface: The
SynchronizedAction
functional interface enables a clean method signature for our synchronized execution. - Decorator Implementation: By isolating the synchronization logic from the business logic, we keep our code modular and maintainable. The
synchronizedExecute
method encapsulates the locking mechanism. - Lambda Expressions: This approach allows for concise syntax when defining actions to be synchronized. This makes our code easier to read and understand.
Benefits of Using Synchronized Decorators
- Clarity: Your synchronization logic is centralized and reusable, which reduces the cognitive load when maintaining the code.
- Flexibility: You can wrap any action with a synchronized decorator indiscriminately, making it easy to adapt to different contexts.
- Modularity: This approach promotes a clean separation of concerns. The logic of what needs to be synchronized is separate from how it is synchronized.
Example Usage
Let’s see how our Account
class operated within a multithreaded environment.
public class BankExample {
public static void main(String[] args) {
Account account = new Account(1000);
Thread t1 = new Thread(() -> account.deposit(200));
Thread t2 = new Thread(() -> account.withdraw(150));
t1.start();
t2.start();
try {
t1.join();
t2.join();
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
System.out.println("Final Account Balance: " + account.getBalance());
}
}
Commentary on the Example Usage
In this example, two threads attempt to update the same account
object concurrently. The synchronized decorator ensures that each transaction completes without interference, maintaining a consistent state.
Additional Considerations
While using synchronized decorators is beneficial, it’s essential to be aware of their limitations:
- Performance Overhead: Excessive synchronization can lead to contention, causing threads to wait unnecessarily. Always analyze the bottlenecks in your application.
- Granularity: Consider the scope of your locked sections. More granular control tends to yield better performance while ensuring thread safety.
- Deadlocks: Carelessly managing locks can lead to deadlocks, where threads are waiting indefinitely for each other’s locks.
Closing Remarks
Synchronized decorators offer a powerful and elegant solution to the common challenges associated with thread safety in Java. Whether you are working on a finance application with real money transactions or a simpler program requiring shared resource access, ensuring thread safety is paramount. By following the structured approach outlined in this post, you can improve the maintainability and clarity of your code while keeping it secure from the pitfalls of concurrent programming.
While Java provides built-in mechanisms for thread safety, employing synchronized decorators can enhance your coding experience, providing synchronization in a compact and readable form. Always remember that the key to effective multithreading is not just making your code work, but making it clean, understandable, and maintainable.
For further reading on thread safety in Java, you can check out Oracle's Official Documentation.
Embrace the power of synchronized decorators and build robust Java applications that can handle the intricacies of concurrent processing!