Overcoming Challenges in Top-Down Web Service Development
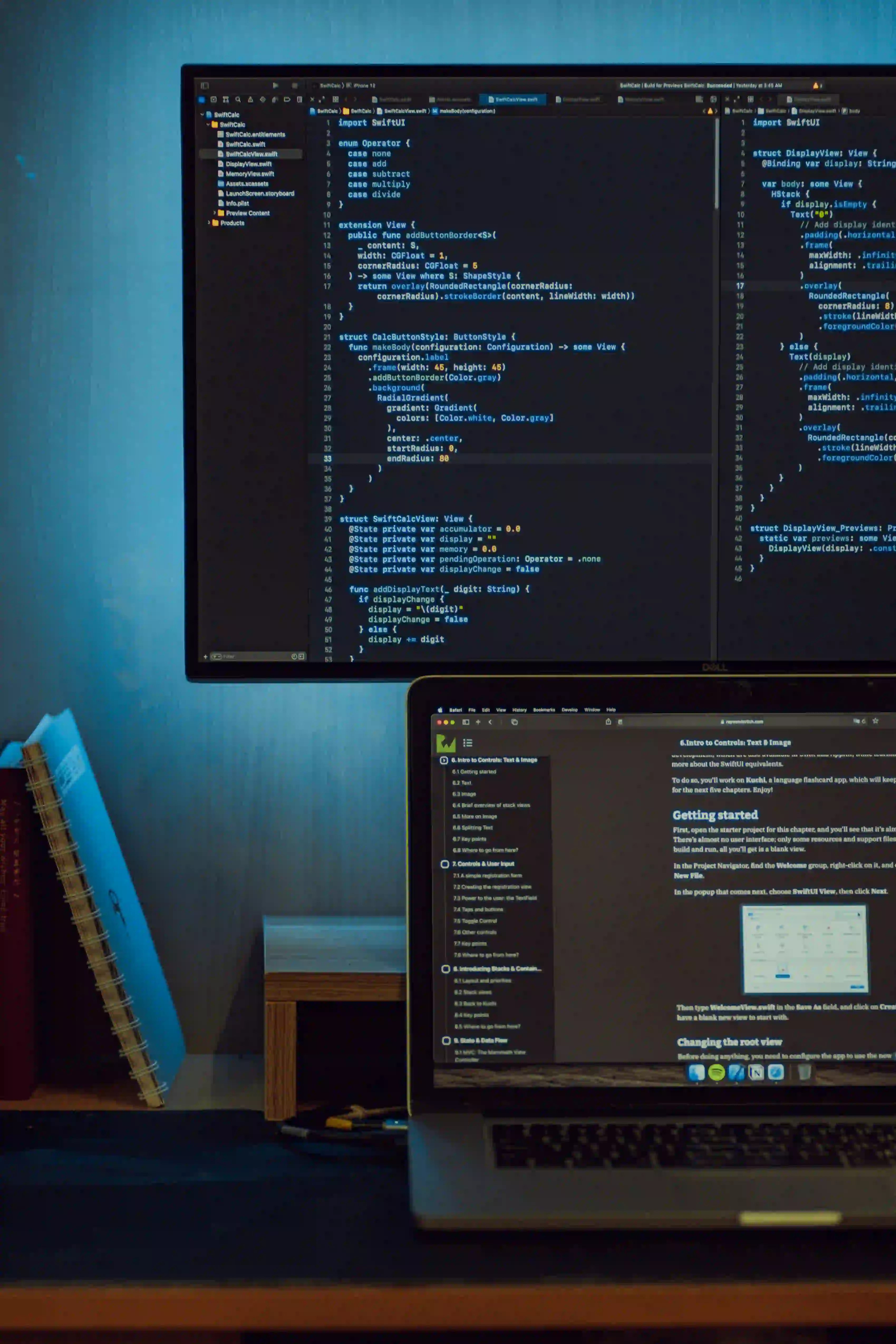
Overcoming Challenges in Top-Down Web Service Development
In the ever-evolving world of software architecture, web services play a critical role in enabling applications to communicate over the internet. One popular design approach for constructing web services is called "top-down development." This method involves starting with a comprehensive service specification (often via a WSDL file), followed by incremental implementation. Despite its strengths, developers face unique challenges with this approach. Below, we will explore these challenges and strategies to overcome them using Java, complemented with illustrative code snippets.
Understanding Top-Down Web Service Development
Top-down web service development is a design methodology where the service interface is defined before the implementation. This means that any client application must adhere to the predetermined structure of the web service.
Advantages of Top-Down Development
- Early Validation: By defining the service specifications first, developers can validate client requirements before coding.
- Standardization: Using WSDL enforces a structured format for service interfaces.
- Better Integration: All teams know the schema up front, which eases integration across different applications.
Disadvantages of Top-Down Development
- Rigid Structure: Changes in business logic may lead to service revisions, causing potential chaos in client applications.
- Higher Initial Overhead: Setting up a detailed service definition takes time upfront.
- Complex Maintenance: Updating the service specification requires careful handling to avoid breaking existing clients.
Key Challenges in Top-Down Development
1. Specification Overhead
Creating an extensive WSDL or service contract can be time-consuming, particularly for large applications. This overhead may lead to analysis paralysis, where developers get stuck understanding specifications instead of coding.
Solution: Start Small and Iterate
Begin with a minimal viable service definition, then iteratively enhance it based on client feedback.
@WebService
public class SimpleWebService {
@WebMethod
public String greet(String name) {
return "Hello, " + name + "!";
}
}
In this example, we define a simple web service with a single method greet
. By starting with a straightforward design, we mitigate initial overhead while still delivering value.
2. Change Management
As requirements evolve, updating the service definition can present challenges, especially with existing clients that depend on the original structure.
Solution: Versioning
Implement service versioning to manage changes effectively without disrupting existing integrations.
<wsdl:service name="SimpleWebServiceV1">
<wsdl:port name="SimpleWebServicePort" binding="tns:SimpleWebServiceBinding">
<soap:address location="http://example.com/SimpleWebServiceV1" />
</wsdl:port>
</wsdl:service>
In this WSDL excerpt, we can see how we might define different versions of the SimpleWebService
. By using a unique endpoint, client applications can seamlessly use the older or newer versions of the web service.
3. Compliance with Standards
While top-down service development often aims for compliance, various stakeholders may have different interpretations of standards. This can lead to misunderstandings and misalignments.
Solution: Documentation and Validation
Document service expectations comprehensively and validate compliance using tools like Apache CXF or JAX-WS.
@WSDLDocumentation("This method greets a user based on the given name.")
@WebMethod
public String greet(@WebParam(name = "name") String name) {
return "Hello, " + name + "!";
}
This snippet showcases how documentation within service methods helps clarify intent and expectations. Well-documented services improve communication across teams and enhance compliance.
4. Performance Considerations
Web services often introduce overhead in terms of latency compared to other architectures like microservices. Performance is crucial as it can affect user experience.
Solution: Optimize Your Service
Measure performance using tools like JMeter or Apache Bench and continuously refine the service layers.
@WebService
public class OptimizedWebService {
@WebMethod
public String processData() {
// Assuming this method processes large data
long startTime = System.currentTimeMillis();
// ...perform data processing...
long processingTime = System.currentTimeMillis() - startTime;
return "Processing time: " + processingTime + " ms";
}
}
In this service method, we are measuring the processing time for a specific task. By keeping track of performance metrics, we can identify bottlenecks and improve efficiency.
5. Error Handling
Error identification can become cumbersome in a top-down approach, as services are heavily dependent on predefined XML schemas.
Solution: Robust Error Handling
Implement structured error handling to provide clear feedback when issues arise.
@WebMethod
public String safeGreet(@WebParam(name = "name") String name) {
if (name == null || name.isEmpty()) {
throw new IllegalArgumentException("Name must not be empty");
}
return "Hello, " + name + "!";
}
This approach introduces error handling at the service level. If a client passes an invalid argument, the service throws an informative exception, improving the overall resilience of the system.
To Wrap Things Up
Top-down web service development provides a unique set of advantages and challenges. While it promotes standardization and early validation, careful attention must be paid to overhead, change management, compliance, performance, and error handling.
By applying the strategies outlined above, developers can effectively navigate the challenges inherent in top-down web service development using Java. For further reading, consider exploring resources like the Java Web Services Tutorial and best practices in API design.
As we continue to innovate in web service design, remembering these challenges and strategies will foster smoother transitions to successful implementations, ensuring top-notch user experiences in our applications.